How to manage exceptions thrown in filters in Spring?
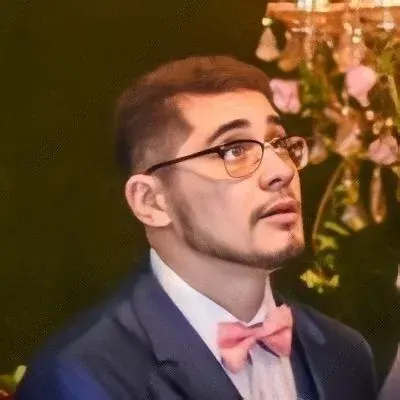
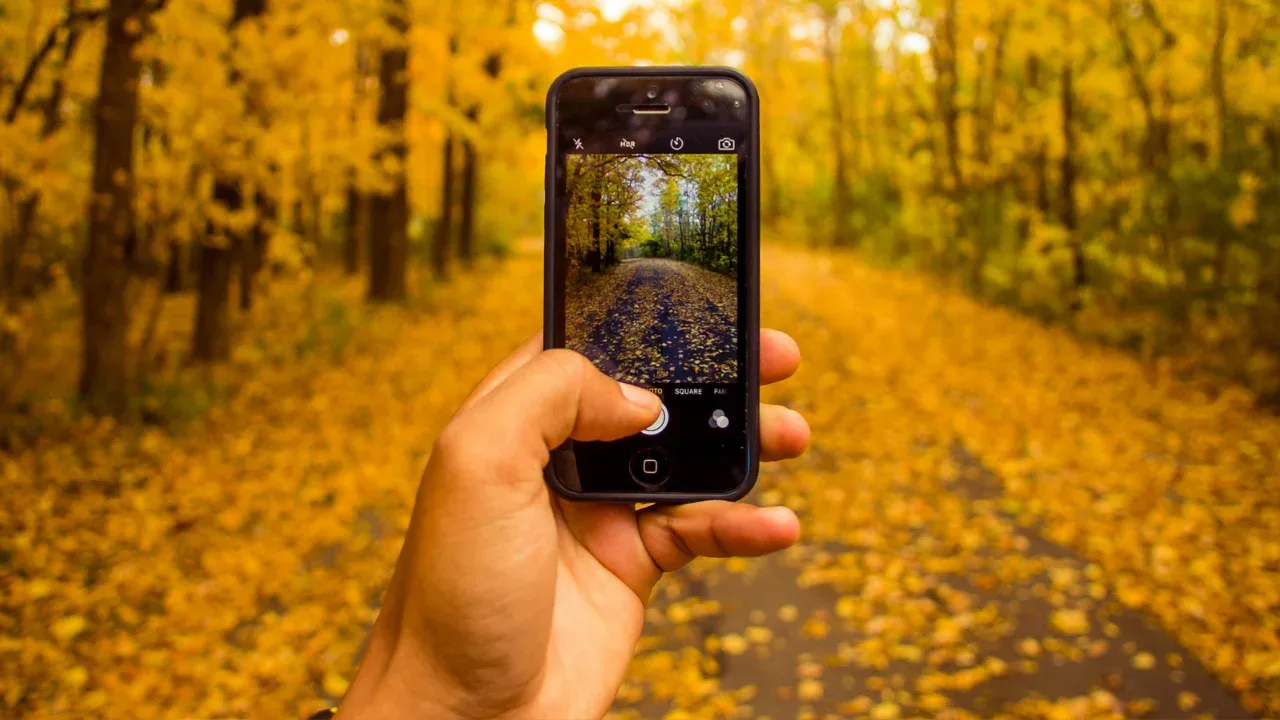
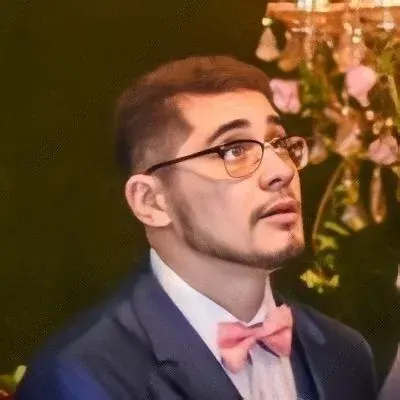
How to Manage Exceptions Thrown in Filters in Spring? 😕💥🔧
Are you having trouble managing exceptions thrown in filters in your Spring application? 😟 Don't worry, you're not alone! Many developers struggle with this issue as well. In this blog post, we will address common problems and provide easy solutions that will help you handle exceptions gracefully. So, let's dive in! 🏊♂️💻
The Problem at Hand 😬🔍
One of our readers, let's call them DevPro2000, is facing an issue where they want to handle 5xx error codes generically. Specifically, they want to display a pretty error JSON message instead of a stack trace when the database is down across their entire Spring application. So far, they have implemented a @ControllerAdvice
class to handle exceptions thrown by controllers, which works well for most cases, including when the database stops in the middle of a request. However, they also have a custom CorsFilter
that extends OncePerRequestFilter
, and when calling doFilter
, they encounter a CannotGetJdbcConnectionException
, which is not being managed by the @ControllerAdvice
.
Addressing the Questions ❓📢
Do I need to implement a custom filter? 😕 DevPro2000 mentioned finding the
ExceptionTranslationFilter
, but it seems to handle onlyAuthenticationException
orAccessDeniedException
, not the specific exception they are facing. While creating a custom filter is an option, let's explore more obvious alternatives.Should I implement my own HandlerExceptionResolver? 😕 DevPro2000 considered this approach but had doubts since they don't have a custom exception to manage. They also attempted to use a try/catch block with an implementation of
HandlerExceptionResolver
, but it resulted in a 200 status code and an empty response body. Let's find a better solution together!
The Obvious Way(s) to Handle Exceptions in Filters 🤷♂️✅🔌
DevPro2000, great questions! Here are a couple of approaches you can take to tackle this issue:
Approach 1: Extend OncePerRequestFilter
and Override doFilterInternal
🔍🛠
By extending OncePerRequestFilter
, you can override the doFilterInternal
method to handle exceptions thrown in your custom CorsFilter
.
public class CustomCorsFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
try {
filterChain.doFilter(request, response);
} catch (CannotGetJdbcConnectionException ex) {
// Handle the exception here
}
}
}
Inside the catch
block, you can handle the CannotGetJdbcConnectionException
as required. This will ensure that the exception is caught within the filter itself.
Approach 2: Use HandlerExceptionResolver
in @ControllerAdvice
🤝🔌
You can leverage the power of HandlerExceptionResolver
in your existing @ControllerAdvice
class to manage the exception thrown by your filter.
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
// ... existing exception handlers ...
@ExceptionHandler(CannotGetJdbcConnectionException.class)
public ResponseEntity<Object> handleCannotGetJdbcConnectionException(CannotGetJdbcConnectionException ex, WebRequest request) {
// Handle the exception and return a ResponseEntity with the desired error JSON
}
}
By adding an @ExceptionHandler
method for CannotGetJdbcConnectionException
, you can define how you want to handle this specific exception. Return a ResponseEntity
with the desired error JSON to ensure a clean and informative response.
Your Turn! 🙌🚀
DevPro2000, we've provided you with two approaches to handle exceptions thrown in filters in your Spring application. Now, it's your turn to implement the solution that suits your needs best. 💪 Don't forget to let us know how it goes or if you have any more questions!
If you found this blog post helpful, feel free to share it with your fellow developers. Let's spread the knowledge! 📢✨
Happy coding! 😄👨💻