How to make a new List in Java
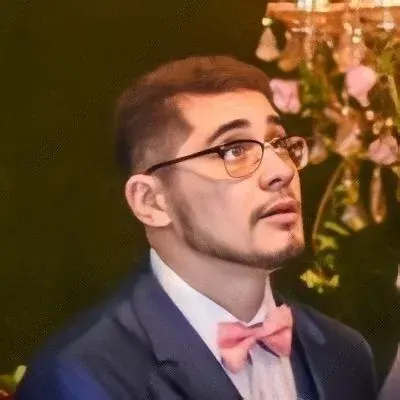
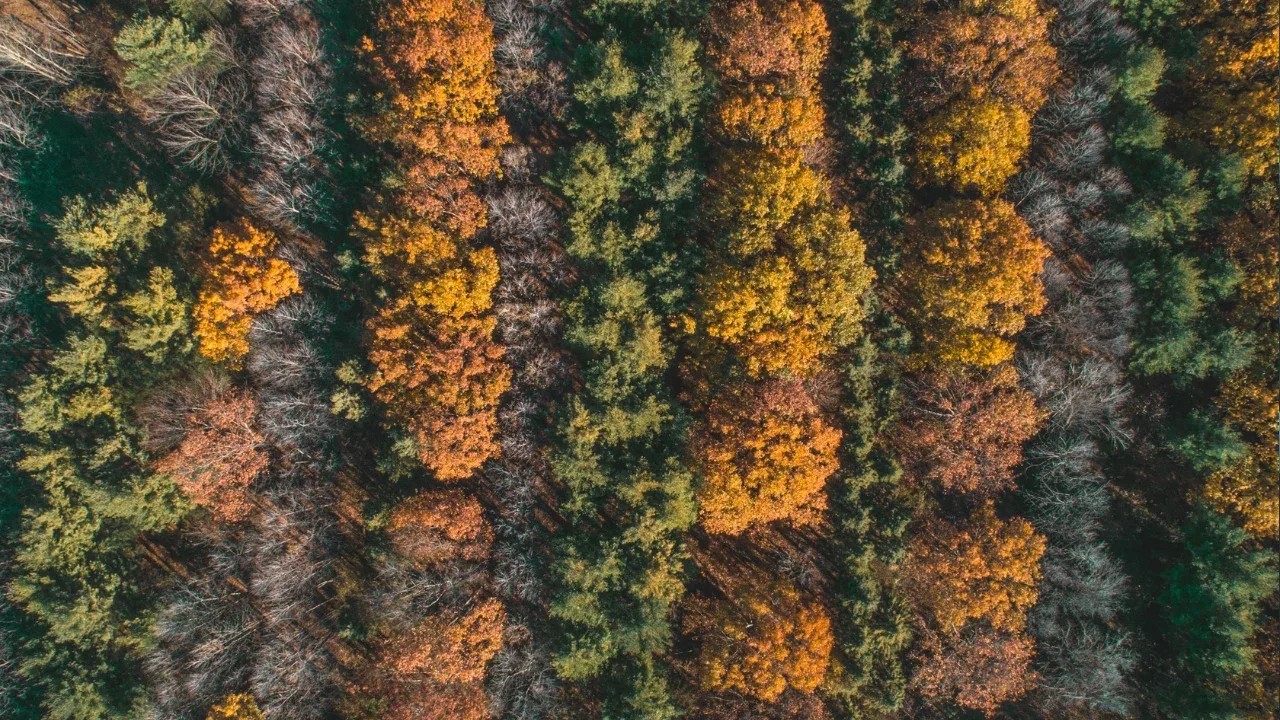
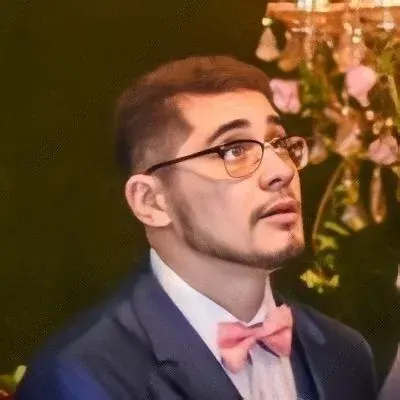
📝 Creating a New List in Java: A Simple Guide 📝
Are you tired of trying to figure out how to create a new List in Java? Look no further! In this blog post, we will walk you through the process step-by-step and provide easy solutions to common issues. So, let's dive right in! 💪
💡 Understanding Lists in Java
First, let's discuss what a List is in Java. A List is an ordered collection of elements that allows duplicates. Unlike a Set, which uses the Set
interface, a List utilizes the List
interface in Java.
🚀 Creating a List in Java
To create a new List in Java, follow these simple steps:
Import the necessary package:
import java.util.List;
import java.util.ArrayList;
Declare the List variable:
List<String> myList = new ArrayList<>();
Here, we have declared a List named myList
that can store elements of type String
. You can replace String
with any other data type that you need.
Initialize the List (optional):
myList.add("Element 1");
myList.add("Element 2");
If you want to add elements to the List during its creation, you can use the add()
method as shown above. This step is optional, and you can add elements later as well.
That's it! You have successfully created a new List in Java. Wasn't that easy? 😊
⚡️ Common Issues and Easy Solutions
Issue 1: Forgetting to import the necessary package
If you forget to import the required packages (java.util.List
and java.util.ArrayList
), you will encounter a compilation error. To fix this, simply add the following import statements at the top of your Java file:
import java.util.List;
import java.util.ArrayList;
Issue 2: Adding elements of the wrong data type
When adding elements to your List, make sure they are of the correct data type. For instance, if you have declared a List of type Integer
, trying to add a String-type element will result in a runtime error.
Issue 3: Accidentally initializing an empty List
If you forget to add elements to your List during its creation (i.e., forget to use the add()
method), you will end up with an empty List. To avoid this, remember to add the required elements right after creating the List.
💬 Your Turn: Share Your Thoughts!
Now that you know how to create a new List in Java, it's time to put your knowledge into action! ✍️ Did you find this guide helpful? Do you have any additional tips or tricks to share? Let us know in the comments below!
Happy coding! 💻🎉
Disclaimer: This blog post assumes basic knowledge of Java programming. If you are new to Java, we recommend checking out some beginner-friendly resources before diving into Lists and other data structures.