How to inject dependencies into a self-instantiated object in Spring?
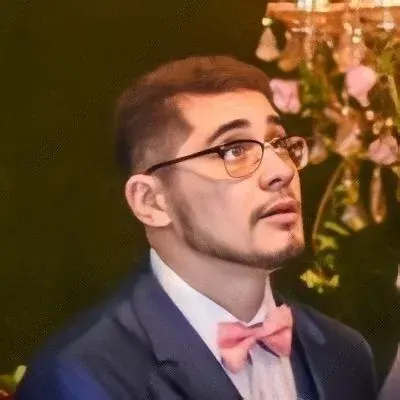
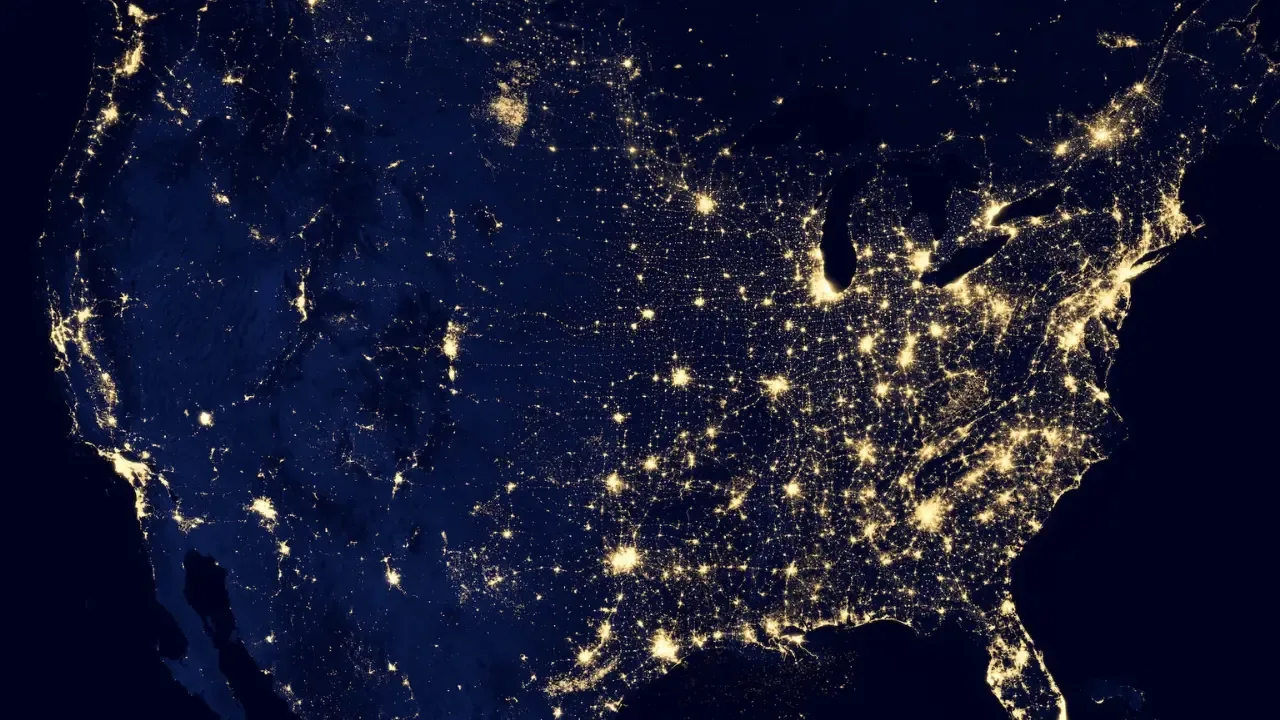
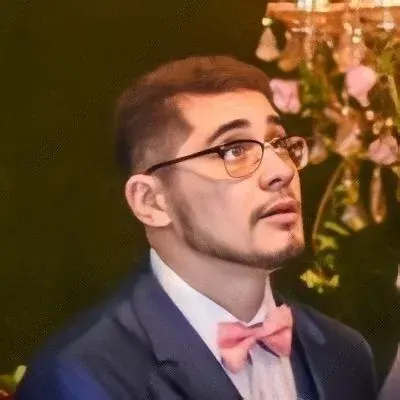
🌟 Injecting Dependencies into a Self-Instantiated Object in Spring 🌟
So you've stumbled upon a tricky question in the world of Spring dependency injection: how to inject dependencies into a self-instantiated object? 🤔 Don't worry, my tech-savvy friend, because we've got you covered! In this blog post, we'll explore this common issue and provide you with easy solutions. 💪
First, let's set the stage. Imagine we have a neat class called MyClass
with an @Autowired
annotation on a private field. It looks something like this:
public class MyClass {
@Autowired private AnotherBean anotherBean;
}
Now, hold your breath, we come across a situation where we create an object directly by ourselves (or perhaps some alien framework instantiates this object). Brace yourself, here comes the code:
MyClass obj = new MyClass();
Naturally, the question arises: can we still inject the dependencies into this self-instantiated object? Wouldn't it be great if we could do something like this:
applicationContext.injectDependencies(obj);
(Google Guice enthusiasts know what I'm talking about here 😉)
Well, dear reader, here's the thing: Spring doesn't provide a direct mechanism to inject dependencies into self-instantiated objects like our MyClass
example. But, don't let that deflate your tech-loving spirit! There are alternative ways to achieve what you're looking for. Let's dive into a couple of practical solutions:
Solution 1: Leveraging the ApplicationContext
A clever way to inject dependencies into a self-instantiated object is by utilizing the ApplicationContext
. You can follow these steps to leverage this approach:
Make sure your
MyClass
instance is a Spring-managed bean. You can do this by either annotating it as a@Component
or registering it through XML configuration.Obtain a reference to the
ApplicationContext
in your code. You can achieve this by implementing theApplicationContextAware
interface.Use the
ApplicationContext
to retrieve an instance of yourMyClass
object. This ensures that the dependencies are properly injected.
Here's an example implementation:
@Component // or register it in XML configuration
public class MyClass implements ApplicationContextAware {
@Autowired private AnotherBean anotherBean;
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext context) {
applicationContext = context;
}
public static MyClass getInstance() {
MyClass obj = applicationContext.getBean("myClass", MyClass.class);
applicationContext.getAutowireCapableBeanFactory().autowireBean(obj);
return obj;
}
}
Voilà! By utilizing the ApplicationContext
and making use of its getBean()
method, you can inject dependencies into your self-instantiated object. 😎
Solution 2: Manual Dependency Injection
If you prefer a more hands-on approach, fear not, my eager friend! Another way to inject dependencies into a self-instantiated object is through manual dependency injection. This involves explicitly setting the required dependencies after instantiating the object.
Let's take a look at how this can be achieved:
MyClass obj = new MyClass();
obj.setAnotherBean(anotherBean); // Manually set the dependency
By providing a setAnotherBean()
method in the MyClass
class and manually assigning the dependency, you've triumphantly injected the necessary dependencies into your self-instantiated object. 🙌
Your Action Time! 🎉
Now that you're armed with the knowledge of injecting dependencies into self-instantiated objects, it's time to put this newfound wisdom into action!
Choose the approach that best suits your needs and give it a try. Experiment, tinker, and share your experience in the comments section below! We'd love to hear about the approach you took and any tips or tricks you discovered along the way. Let's celebrate the wonderful world of Spring dependency injection together! 🎊
Remember, learning is an adventure, so always embrace new challenges and share the knowledge with fellow tech enthusiasts. Happy coding! 👩💻👨💻
Now you know how to inject dependencies into a self-instantiated object in Spring! 🌈