How to initialize HashSet values by construction?
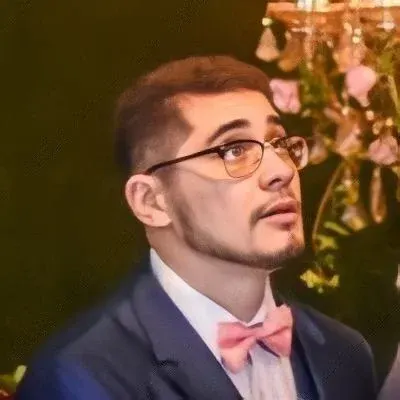
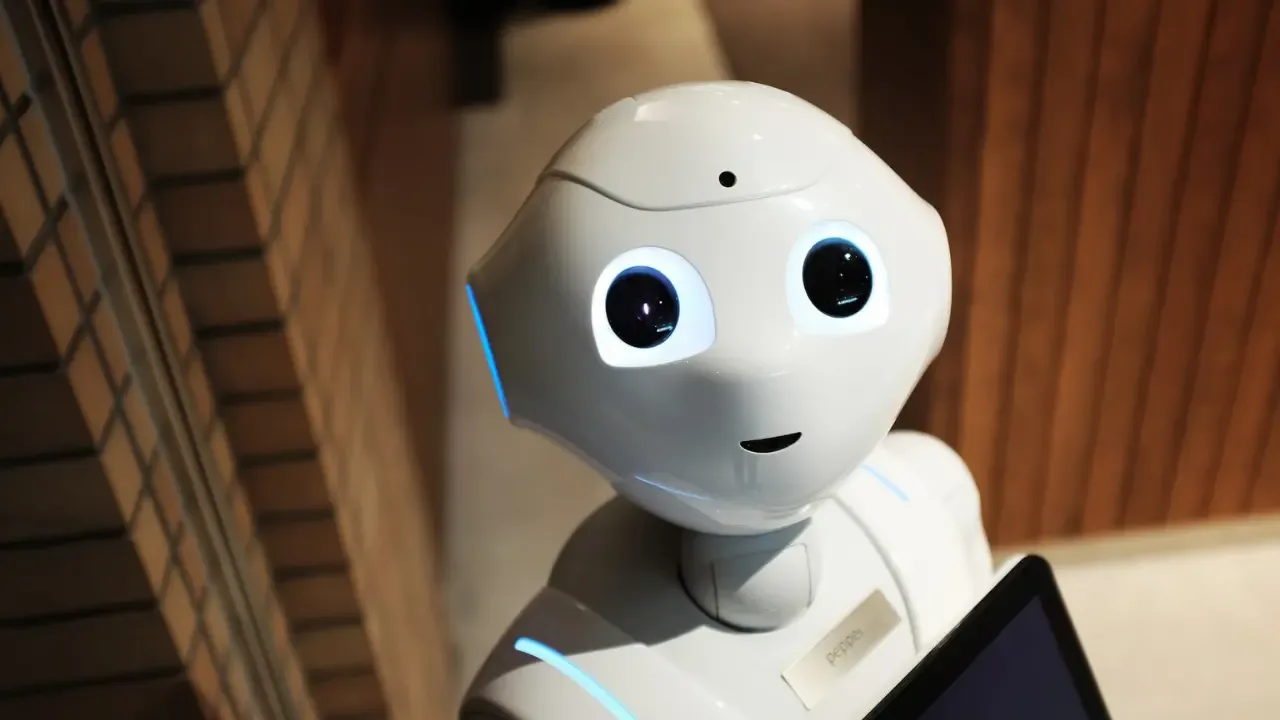
🌟 Get Started with Initializing HashSet Values by Construction 🌟
Have you ever found yourself in a situation where you need to create a Set
with initial values? Typically, you would instantiate a HashSet
and then manually add each element one by one. But what if there's a way to achieve this in just one line of code? 🤔
Let's dive into the common issue and discover easy solutions to help you initialize HashSet values by construction. By the end of this post, you'll be equipped with a simple and efficient way to handle this problem. 💪
🔎 The Common Issue: Manual Initialization 📝
Picture this scenario: you're working on a project, and you need to create a HashSet
with initial values. The usual approach involves instantiating an empty HashSet
and then individually adding each element:
Set<String> h = new HashSet<String>();
h.add("a");
h.add("b");
But doesn't it feel tedious and time-consuming to add multiple elements one by one? Plus, what if you need to declare a final static field using this approach? Manually initializing each value in separate lines would be far from ideal. 😫
🚀 The Easy Solution: Constructor Initialization 💡
Fortunately, Java provides us with a convenient way to tackle this issue using constructor initialization. Instead of manually adding each element, you can initialize the HashSet
with a collection of elements right from the start.
Here's how you can accomplish that in just one line of code:
Set<String> h = new HashSet<>(Arrays.asList("a", "b"));
By using the Arrays.asList()
method with our desired elements inside, we can pass the resulting list directly to the HashSet
constructor. This way, you can populate your Set
with initial values without any hassle. 🙌
🌟 Pro Tip: Immutable Sets with Java 9+ ✨
Starting from Java 9, you can utilize the Set.of()
method to create immutable sets with initial values. This approach is especially handy when you want to declare a final static field. Check out the example below:
Set<String> immutableSet = Set.of("a", "b");
Using Set.of()
, you've initialized an immutable set right away! No need to worry about accidentally modifying its contents. 🧊
⚡️ Engage and Share Your Thoughts! 📣
Now that you've learned how to initialize HashSet values by construction, it's time to put your knowledge into action! Try implementing this code snippet in your projects and see the difference it makes. Don't hesitate to share your experience and any other tips you may have!
🔥 Remember, sharing is caring! If you found this post helpful, spread the word to your fellow developers by clicking the share button below. Let's make initializing HashSets a breeze for everyone! 💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
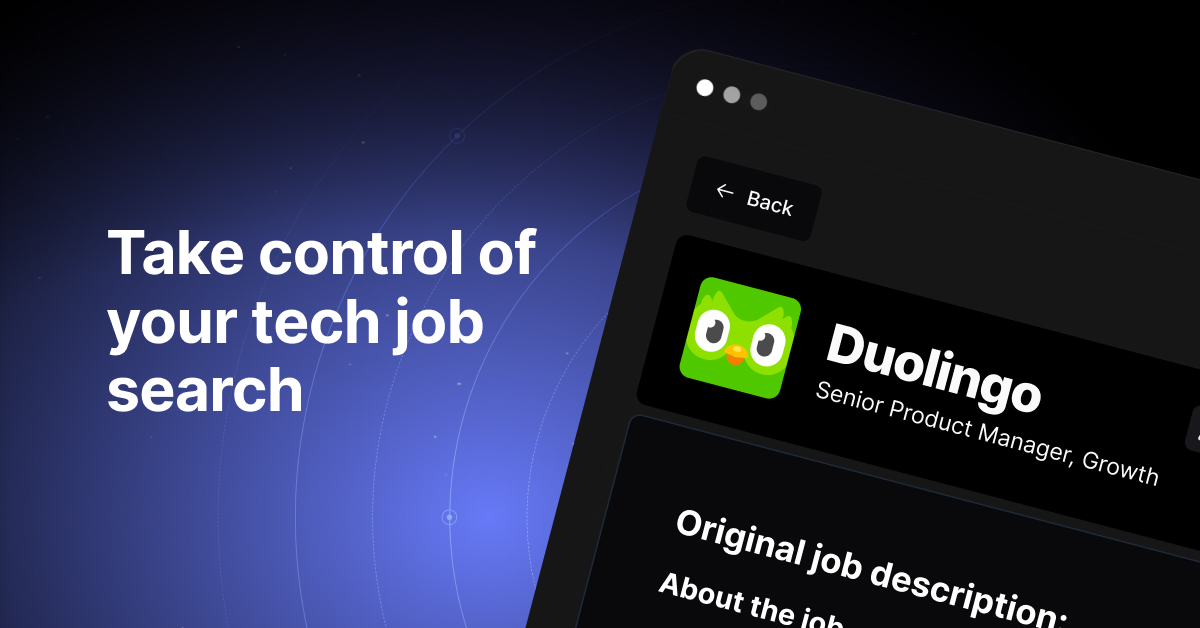