How to initialize an array in Java?
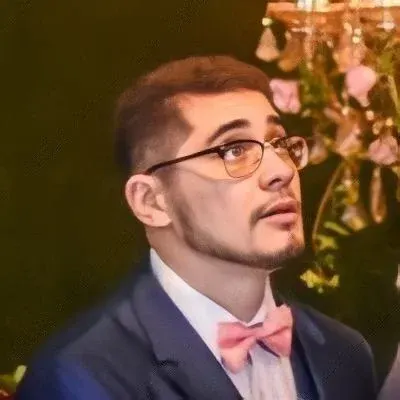

How to Initialize an Array in Java 📚
Initializing an array in Java is a common task, but it can sometimes lead to confusion and errors. In this blog post, we'll address the issue raised by one of our readers and provide easy solutions to help you overcome similar problems. Let's jump right into it! 💪
Understanding the Problem ❓
Our reader encountered an error when trying to initialize an array in their Java code. Here's the code snippet they shared:
public class Array {
int data[] = new int[10];
public Array() {
data[10] = {10,20,30,40,50,60,71,80,90,91};
}
}
The error occurs on the line:
data[10] = {10,20,30,40,50,60,71,80,90,91};
Identifying the Issue 🕵️♂️
The error message is due to incorrect syntax when assigning values to an array element. In Java, you can't assign values to multiple array elements using curly braces like you would in some other programming languages.
Easy Solutions 💡
To solve the problem, you have a couple of options:
Solution 1: Initialize the Array Using a Loop 🔄
One way to initialize the array with the desired values is to use a loop. Here's how you can modify the Array
class to achieve this:
public class Array {
int data[] = new int[10];
public Array() {
for (int i = 0; i < data.length; i++) {
data[i] = i * 10 + 10;
}
}
}
This loop assigns values to each element of the data
array based on a specific pattern. You can customize the pattern according to your needs.
Solution 2: Initialize the Array at Declaration 🌟
Another approach is to initialize the array directly at the declaration, without using a loop. Here's an example:
public class Array {
int data[] = {10,20,30,40,50,60,71,80,90,91};
public Array() {
// Constructor code
}
}
In this solution, the array is initialized with the desired values right after its declaration. This approach is more concise and can be ideal if you know the array elements in advance.
Wrap Up and Get Coding! ✨
Initializing an array in Java doesn't have to be a complicated task. By understanding the error and utilizing the provided solutions, you can easily tackle similar issues in your code.
Remember to choose the solution that best suits your specific requirements. Whether you decide to use a loop or initialize the array directly at declaration, make sure to test your code to ensure it functions as expected.
So go ahead, solve the problem, and happy coding! 😄
Got any other questions or need further clarification? Don't hesitate to leave a comment down below. We're here to help! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
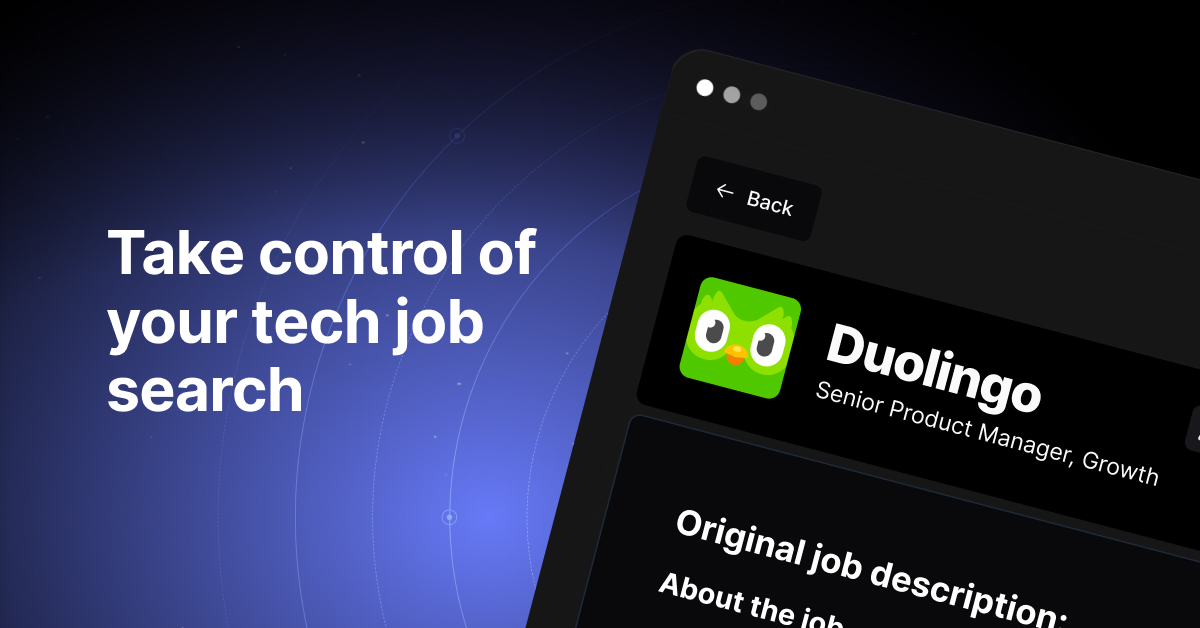