How to get the current time in YYYY-MM-DD HH:MI:Sec.Millisecond format in Java?
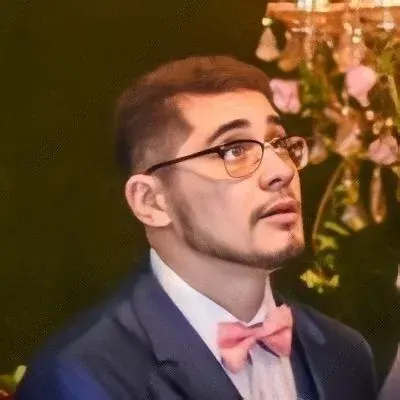
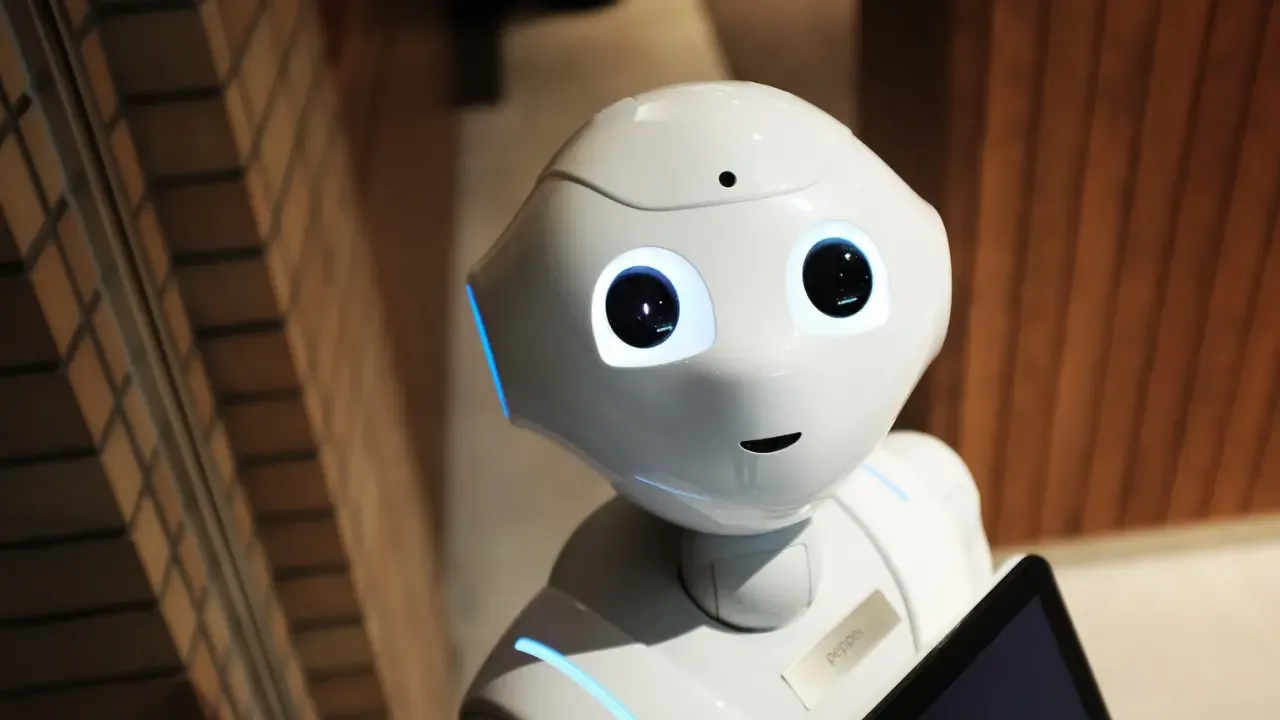
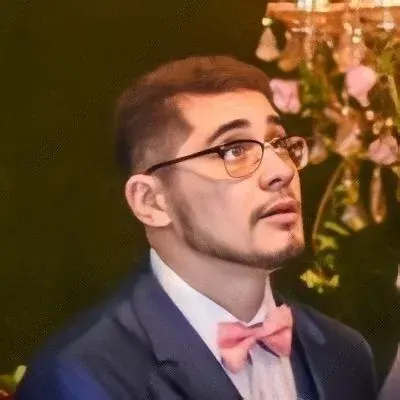
How to Get the Current Time in YYYY-MM-DD HH:MI:Sec.Millisecond Format in Java?
š Have you ever wondered how to get the current time in Java but with milliseconds included? The code snippet you provided is a good start, but it's missing that extra precision. š„ In this blog post, we will address this common issue and provide you with an easy solution. So let's dive in and learn how to retrieve the current time in the desired format!
The Problem
š The code you shared:
public static String getCurrentTimeStamp() {
SimpleDateFormat sdfDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date now = new Date();
String strDate = sdfDate.format(now);
return strDate;
}
does a great job of giving you the current time in the format YYYY-MM-DD HH:MM:SS
(e.g., 2009-09-22 16:47:08
). However, it doesn't include the milliseconds component, which is what you're looking for.
The Solution
š To retrieve the current time in the format YYYY-MM-DD HH:MM:SS.MS
(e.g., 2009-09-22 16:47:08.128
), we need to make a slight modification to the code.
public static String getCurrentTimeStampWithMillis() {
SimpleDateFormat sdfDate = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
Date now = new Date();
String strDate = sdfDate.format(now);
return strDate;
}
By adding an extra SSS
in the date format pattern, we are telling Java to include the milliseconds component. This will give us the desired output with milliseconds.
Example Usage
š” Here's an example of how you can use the getCurrentTimeStampWithMillis()
method:
public static void main(String[] args) {
String currentTime = getCurrentTimeStampWithMillis();
System.out.println("Current Time: " + currentTime);
}
Running the above code will display the current time in the YYYY-MM-DD HH:MM:SS.MS
format in the console.
Conclusion
š And there you have it! With just a small modification to your code, you can now easily retrieve the current time in the YYYY-MM-DD HH:MM:SS.MS
format. This additional precision can be useful in various scenarios where milliseconds matter.
So go ahead, update your code, and enjoy the precision of milliseconds in your timestamps! š
š¢ If you found this blog post helpful, share it with your fellow developers and inspire them to embrace the power of milliseconds in their Java applications! And don't forget to leave a comment below if you have any questions or further suggestions on how to improve this solution.
Happy coding! š»āØ