How to get the current date/time in Java
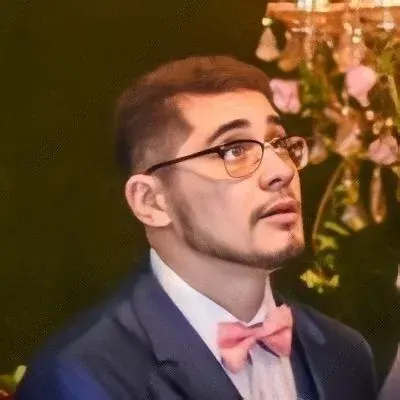
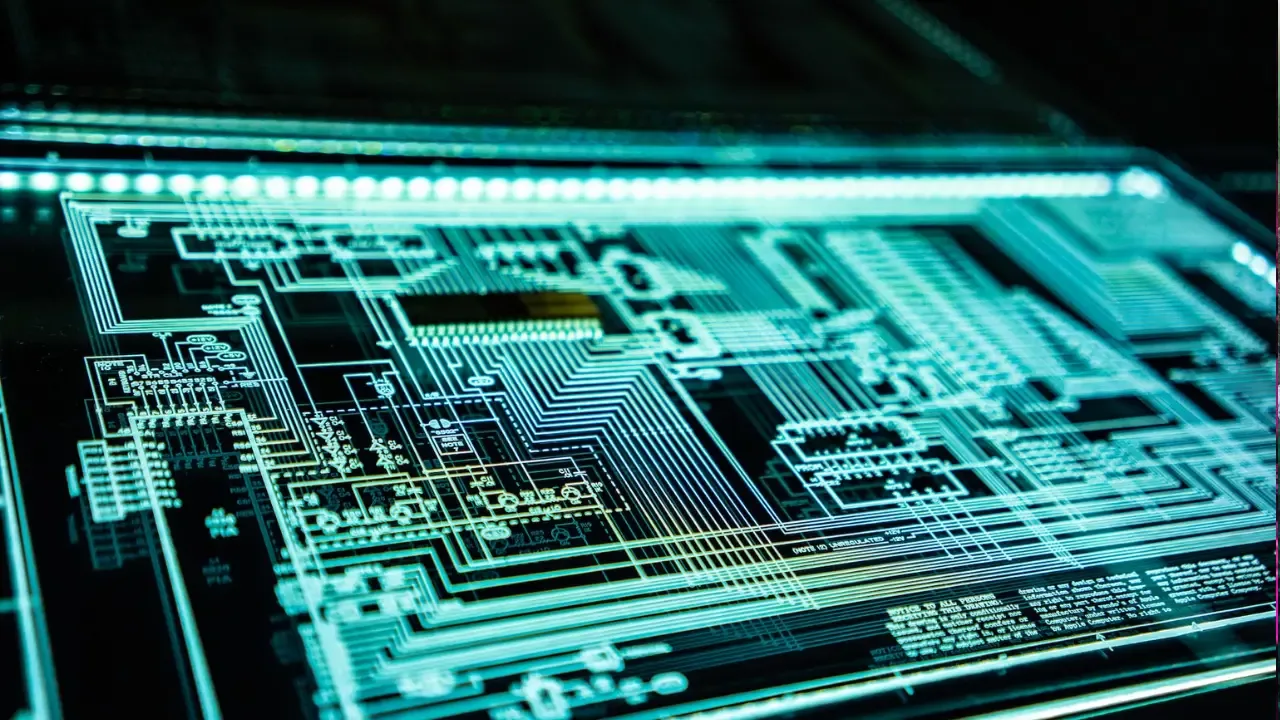
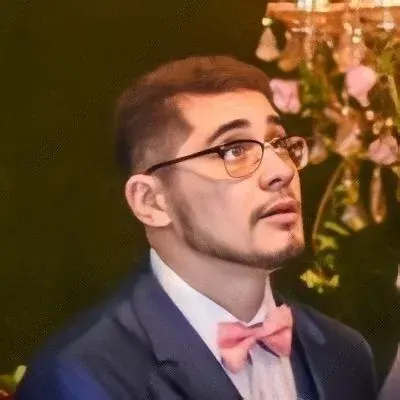
📅 How to Get the Current Date/Time in Java
Are you feeling lost in the vast universe of Java programming while trying to figure out the best way to get the current date and time? Fear not, fellow developers! 🚀 In this blog post, we'll explore common issues, easy solutions, and unleash the power of Java in obtaining the current date and time. ⏰
🕑 The Common Problems
Before diving into the solutions, let's take a moment to understand the common stumbling blocks you may encounter when dealing with dates and times in Java:
Obtaining the System Time Zone: Java has its own way of managing time zones, which can lead to confusion if not handled properly.
Formatting Date/Time Outputs: Depending on your requirements, you might need to tailor the output format of the date and time information. This can be problematic if you're not familiar with Java's formatting syntax.
Using Old Date/Time APIs: Java has evolved over time, bringing with it newer and more efficient date/time APIs. However, some developers may still be stuck using deprecated or outdated classes.
🚀 Solution #1: Using the java.util.Date
Class
The most simplistic way to obtain the current date and time in Java is to use the java.util.Date
class. Here's an example:
import java.util.Date;
public class DateTimeExample {
public static void main(String[] args) {
Date currentDate = new Date();
System.out.println("Current Date/Time: " + currentDate);
}
}
While this approach may solve the problem, it's important to note that the java.util.Date
class is considered legacy and has limitations. It's highly recommended to explore newer alternatives.
🚀 Solution #2: Utilizing the java.time
Package
Java 8 introduced a new date and time API called java.time
, often referred to as the Java 8 Date/Time API. This API provides a more robust and flexible way of handling dates and times. Let's take a look at an example:
import java.time.LocalDateTime;
public class DateTimeExample {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now();
System.out.println("Current Date/Time: " + currentDateTime);
}
}
With the java.time.LocalDateTime
class, you can now easily obtain the current date and time. This API also offers a variety of methods for handling time zones, formatting, and parsing.
🔥 Bonus Tip: Formatting Date/Time Outputs
Suppose you want to customize the output format, such as displaying the date and time in a specific pattern. You can achieve this with the help of the java.time.format.DateTimeFormatter
class.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeExample {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = currentDateTime.format(formatter);
System.out.println("Customized Date/Time: " + formattedDateTime);
}
}
Feel free to experiment with different patterns and explore the extensive options available through the DateTimeFormatter
class.
💡 The Call-to-Action
Congratulations on grasping the art of obtaining the current date and time in Java! 🙌 But don't stop here – dive deeper into the fascinating world of Java's date/time API. Familiarize yourself with its functionalities, explore formatting options, and discover new ways to solve common date and time problems.
Now, it's your turn! Share your favorite Java date/time code snippets or any cool tips and tricks in the comments below. Let's ignite a vibrant discussion and learn from each other's experiences! 🔥💬
Remember, sharing is caring! Don't forget to share this blog post with fellow Java enthusiasts who are in dire need of date/time enlightenment. Spread the knowledge! 🌟✉️
Happy coding! 😄👩💻👨💻