How to get an enum value from a string value in Java
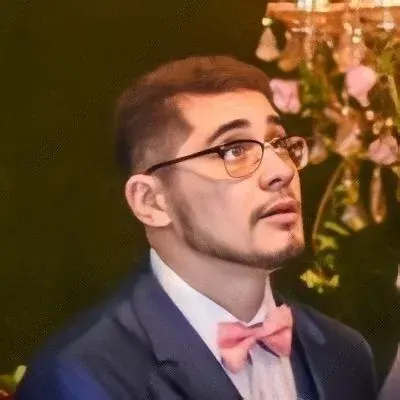
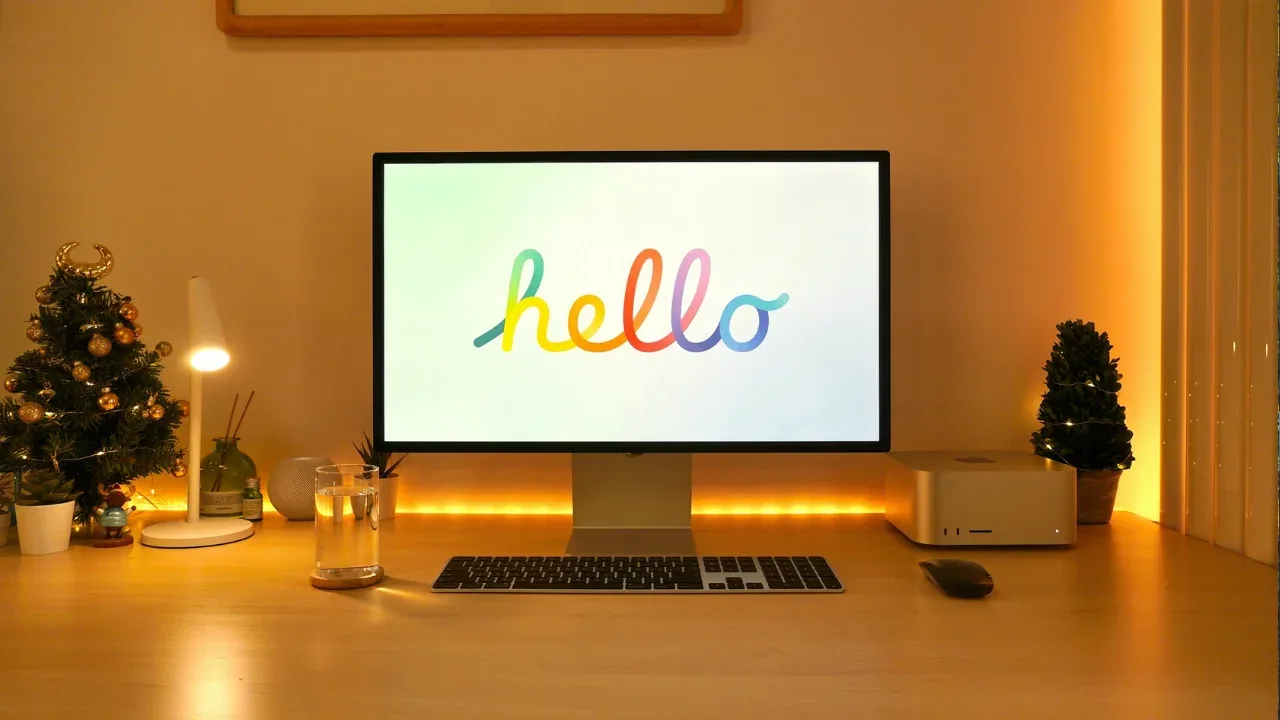
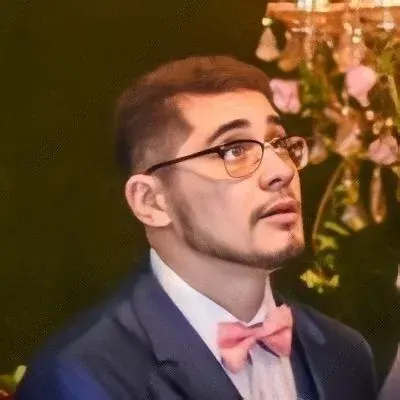
How to Get an Enum Value from a String Value in Java 😎
So, you want to find the enum value of a string in Java? 🤔 You've come to the right place! In this guide, we'll walk through the common issues related to this question and provide you with easy solutions. Let's dive in! 💪
The Enum and String Conundrum 🤷♀️
Let's start with a simple enum called Blah
, which consists of the values A
, B
, C
, and D
. Your goal is to find the enum value of a string like "A"
, which should correspond to Blah.A
. Sounds tricky, right? 🤔
The Solution: Enum.valueOf() to the Rescue! 🚀
Thankfully, Java provides a handy method called Enum.valueOf()
that can help us achieve our goal! This method takes two parameters: the enum class and the string value you want to convert. Here's how you can use it to find the enum value of "A"
in our Blah
enum:
Blah enumValue = Enum.valueOf(Blah.class, "A");
That's it! 🎉 By using Enum.valueOf()
, you can easily retrieve the enum value from a corresponding string.
Handling Exceptions 👐
It's important to note that Enum.valueOf()
throws an IllegalArgumentException
if the given string doesn't match any of the enum's values. To handle this situation gracefully, you can wrap the method invocation in a try-catch block. For example:
Blah enumValue;
try {
enumValue = Enum.valueOf(Blah.class, "Z");
} catch (IllegalArgumentException e) {
// Handle the exception appropriately
enumValue = Blah.DEFAULT; // Assuming you have a DEFAULT value in your enum
}
Remember to take care of potential exceptions that may arise while using Enum.valueOf()
to ensure a smooth execution of your code!
Share Your Wisdom! 🌟
Now that you know how to get an enum value from a string value in Java, go ahead and give it a try in your own projects! If you have any questions or tips to add, feel free to leave a comment below. We'd love to hear from you! 😊
Happy coding! 💻