How to generate a random alpha-numeric string
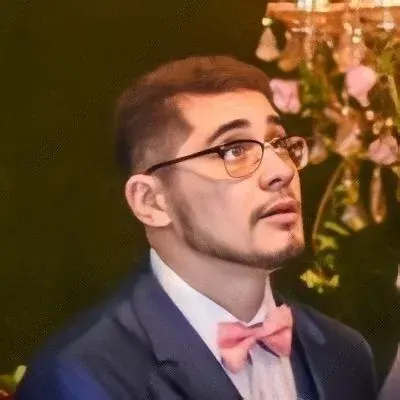
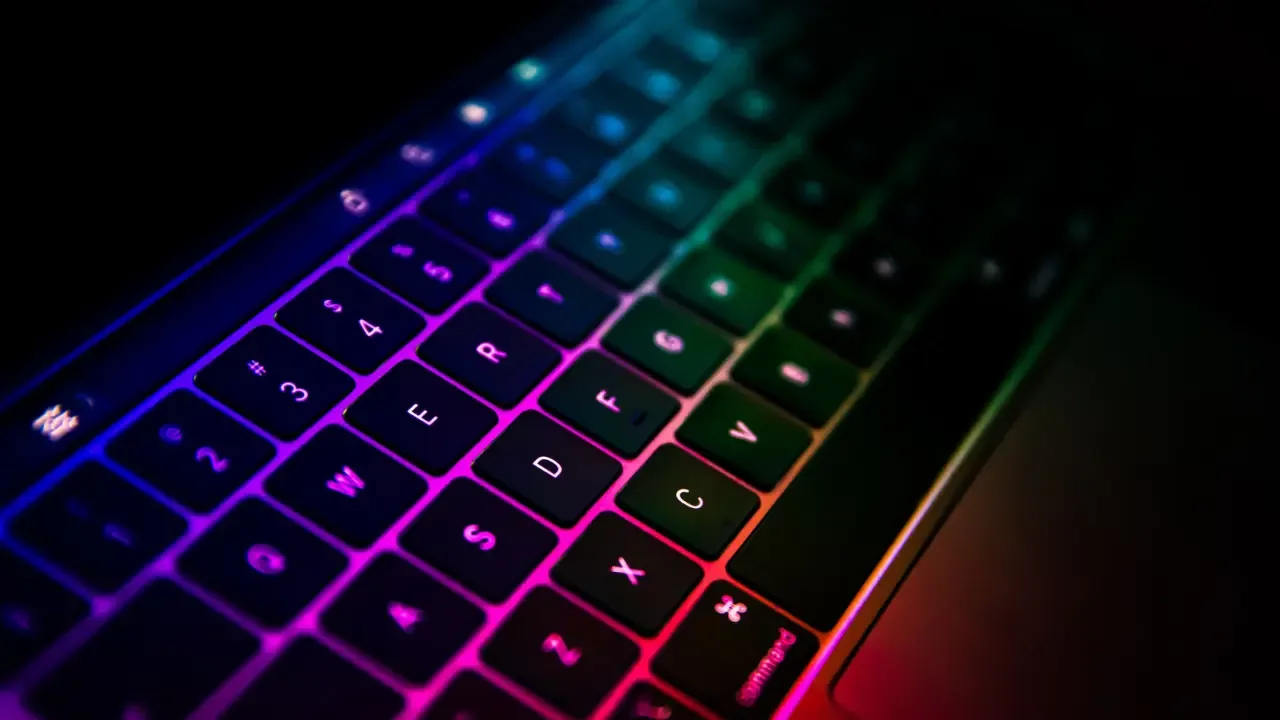
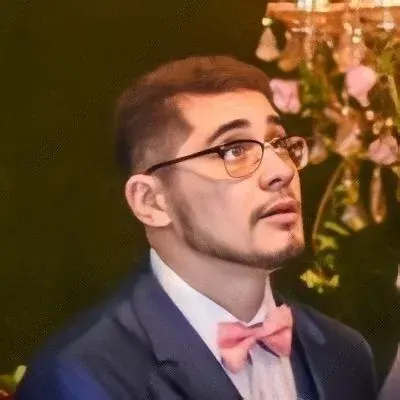
💡 How to Generate a Random Alpha-Numeric String 💡
Are you stuck in a coding vortex, tirelessly browsing through countless forums for a simple Java algorithm to generate a random alpha-numeric string? Look no further, for we're about to unlock the secret to generate pseudo-random, unique session/key identifiers effortlessly!
📝 The Problem: In our case, we're seeking a Java algorithm that can generate a pseudo-random alpha-numeric string of a specified length. This string will serve as a unique session/key identifier, ensuring a reasonably high level of uniqueness for over 500K generations.
🔍 The Solution:
To meet these requirements, we can leverage the power of Java's built-in libraries and features. Let's dive right into a simple solution using the SecureRandom
class and some clever string manipulation.
import java.security.SecureRandom;
import java.util.Locale;
public class RandomStringGenerator {
// Define our pool of alpha-numeric characters
private static final String ALPHANUMERIC_POOL = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
// Generate a random alpha-numeric string of a specific length
public static String generateRandomString(int length) {
SecureRandom random = new SecureRandom();
StringBuilder stringBuilder = new StringBuilder(length);
for (int i = 0; i < length; i++) {
// Get a random index from the alpha-numeric pool
int randomIndex = random.nextInt(ALPHANUMERIC_POOL.length());
// Append the corresponding character to the string
stringBuilder.append(ALPHANUMERIC_POOL.charAt(randomIndex));
}
return stringBuilder.toString();
}
public static void main(String[] args) {
// Generate a random alpha-numeric string of length 12
String randomString = generateRandomString(12);
System.out.println(randomString);
}
}
🔬 How It Works:
We import the necessary classes, including
SecureRandom
for secure pseudo-random number generation.Our
ALPHANUMERIC_POOL
string contains all the valid alpha-numeric characters we'll use to generate our random string.Inside the
generateRandomString()
method, we create an instance ofSecureRandom
and aStringBuilder
to construct our random string.Using a loop, we iterate
length
times, picking random characters from theALPHANUMERIC_POOL
string and appending them to ourstringBuilder
.Finally, we return the generated random string.
🎉 How to Use:
To generate a random alpha-numeric string, simply call the generateRandomString()
method and specify the desired length as an argument. For example, generateRandomString(12)
will give you a 12-character long string.
💡 Call to Action: Now that you have the power to effortlessly generate random alpha-numeric strings, go forth and conquer your coding challenges! Enhance your session/key identification systems, add security layers to your applications, or dive deeper into your coding adventures.
🚀 Take it for a spin and tell us about the creative ways you used this algorithm! Share your experiences and let's push the boundaries of what can be achieved with simple yet effective solutions. Happy coding! 😊✨