How to fix Hibernate LazyInitializationException: failed to lazily initialize a collection of roles, could not initialize proxy - no Session
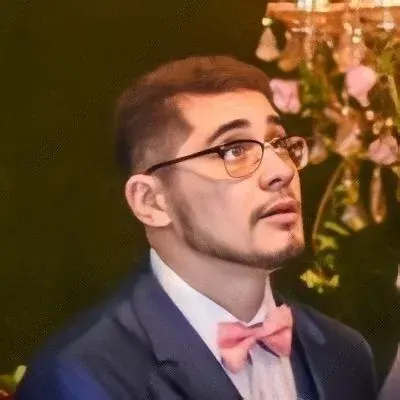
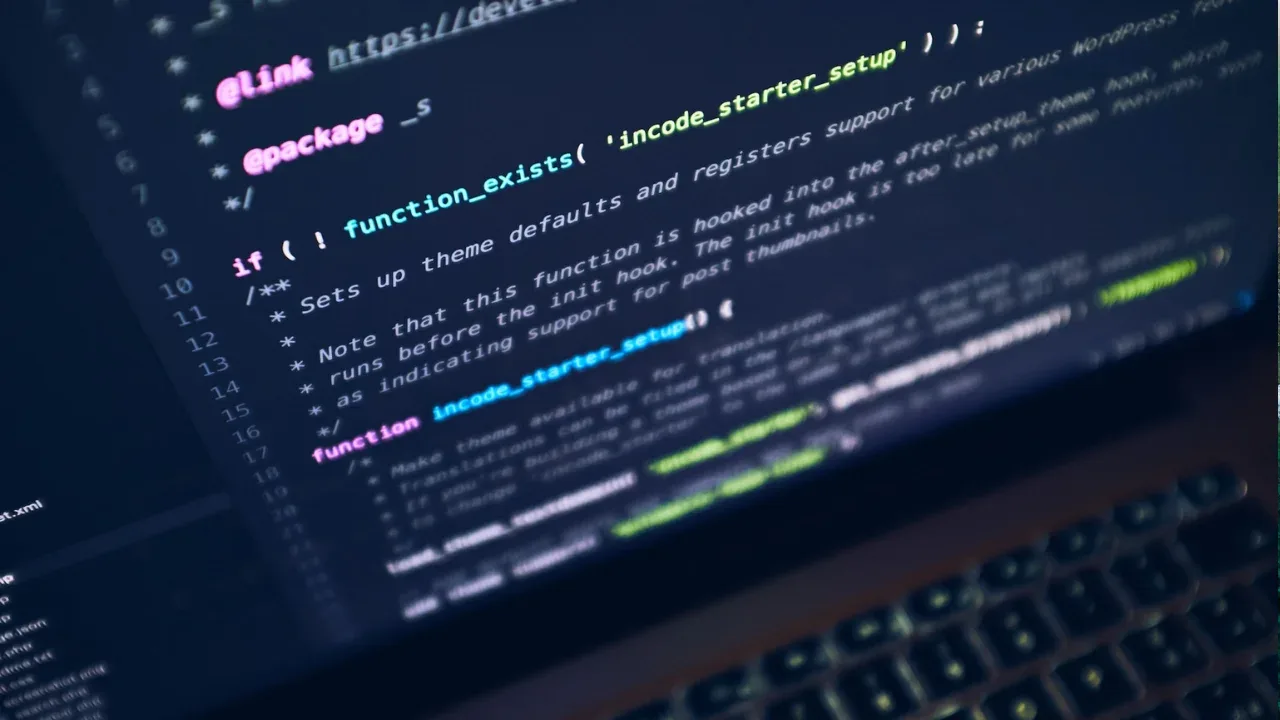
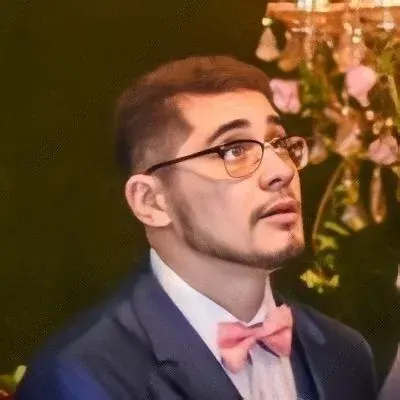
How to fix Hibernate LazyInitializationException: failed to lazily initialize a collection of roles, could not initialize proxy - no Session.
š Hey there! Are you facing the dreaded LazyInitializationException
in your Spring project? Don't worry, I've got you covered! In this blog post, I'll explain the common issues behind this exception, provide easy solutions, and offer a compelling call-to-action to keep you engaged. Let's dive in! šāāļø
Understanding the problem
So you encountered an org.hibernate.LazyInitializationException
with the message "failed to lazily initialize a collection of roles, could not initialize proxy - no Session". This exception usually occurs when you try to access a lazily fetched collection outside the scope of an active Hibernate session.
In your case, the error is happening in the CustomAuthenticationProvider
class when trying to read the list of authorities for the logged-in user. Here's the relevant code snippet:
List<AutorizacoesUsuario> list = user.getAutorizacoes();
Root cause analysis
To understand what's happening here, let's take a closer look at your entity classes. The Usuario
class has a @ManyToMany
relationship with the AutorizacoesUsuario
class. By default, Hibernate uses lazy loading for collections, which means that the autorizacoes
collection is not loaded immediately when you fetch a Usuario
object from the database. Instead, it's loaded on-demand when you access the collection for the first time.
However, in your CustomAuthenticationProvider
class, you're accessing the autorizacoes
collection outside the scope of an active Hibernate session. This leads to the LazyInitializationException
because Hibernate cannot initialize the proxy object associated with the collection.
Easy solutions
To fix this issue, you have a few options:
Option 1: Eagerly fetching the collection
One way to solve the problem is to change the fetching strategy for the autorizacoes
collection to eager loading. This means that the collection will be loaded immediately when you fetch a Usuario
object from the database.
To do this, you can modify the getAutorizacoes()
method in the Usuario
class and add the FetchType.EAGER
option to the @ManyToMany
annotation:
@ManyToMany(cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JoinTable(name = "autorizacoes_usuario", joinColumns = { @JoinColumn(name = "fk_usuario") }, inverseJoinColumns = { @JoinColumn(name = "fk_autorizacoes") })
@LazyCollection(LazyCollectionOption.TRUE)
public List<AutorizacoesUsuario> getAutorizacoes() {
return this.autorizacoes;
}
By eagerly fetching the autorizacoes
collection, you ensure that it is loaded before you access it in the CustomAuthenticationProvider
class. However, keep in mind that eager loading can lead to performance issues if the collection has a large number of elements.
Option 2: Initializing the collection manually
Another solution is to initialize the autorizacoes
collection manually before accessing it in the CustomAuthenticationProvider
class. This can be done by calling the Hibernate.initialize()
method:
import org.hibernate.Hibernate;
// ...
List<AutorizacoesUsuario> list = user.getAutorizacoes();
Hibernate.initialize(list);
By explicitly initializing the collection, you ensure that it is loaded within the scope of an active Hibernate session. This avoids the LazyInitializationException
.
Option 3: Using a custom query
If neither of the previous options works for you, you can consider writing a custom query to fetch the necessary data directly in the CustomAuthenticationProvider
class. This way, you have full control over what data is fetched and how it is loaded.
š£ Your turn to engage!
I hope this blog post helped you understand and solve the LazyInitializationException
issue in your Spring project! If you found this guide helpful, let me know in the comments section below. Also, don't hesitate to share your own experiences and challenges with Hibernate or Spring.
And remember, if you have any other tech-related questions or topics you'd like me to cover, feel free to reach out. I'm here to help you navigate the exciting world of technology! šš
Happy coding! š