How to extract a substring using regex
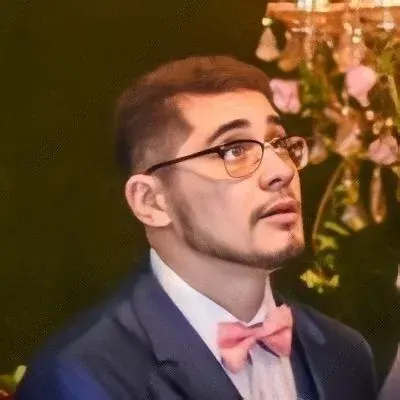
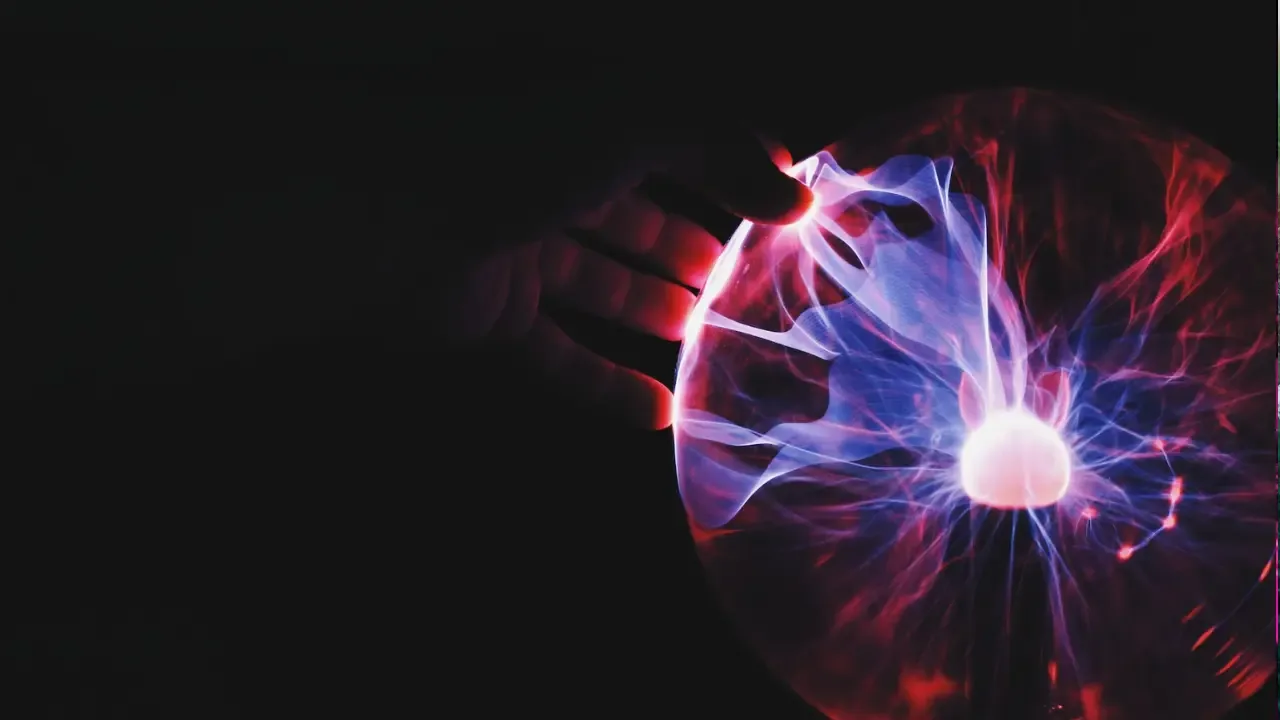
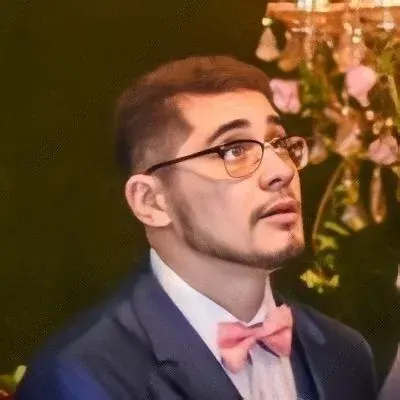
Extracting a Substring with Regex: Unleashing the Power of Regex Magic ✨✂️
So, you have a string that contains a valuable piece of information trapped between two single quotes, and you're wondering how to use regex to extract it. Well, fear not! We're about to embark on a regex adventure that will leave you feeling empowered and ready to conquer any substring extraction challenge!
Understanding the Problem 🤔
Let's start by analyzing the provided example:
mydata = "some string with 'the data i want' inside";
The goal is to extract the substring 'the data i want'
from the given text. We can achieve this by utilizing the power of regular expressions (regex). Regex provides a concise and flexible way to search, match, and extract text patterns from strings.
Crafting the Perfect Regex 🧙♂️🔍
To extract the desired substring, we need to define a regex pattern that identifies the opening and closing single quotes. We can use the following regex pattern to achieve that:
'([^']*)'
Let's break down the pattern and examine each component:
'
matches the opening single quote.[^']*
matches any character that is not a single quote. The[^ ]
is a negated character class, and the*
quantifier allows for zero or more occurrences.'
matches the closing single quote.
Inside the brackets ()
, we have [^']*
, which captures everything inside the single quotes.
Implementing the Solution 💻✨
To extract the desired substring using our regex pattern, follow these simple steps:
Import the
re
module in Python (if you haven't already):
import re
Use the
re.search()
function to find the first match of the pattern in the given string:
mydata = "some string with 'the data i want' inside"
match = re.search(r"'([^']*)'", mydata)
Retrieve the extracted substring from the match object:
if match:
extracted_data = match.group(1)
print(extracted_data)
When you run the above code, it will output:
the data i want
Dealing with Common Issues ⚠️
Multiple Matches
If there are multiple occurrences of the substring you want to extract, and you wish to retrieve all of them, you can use the re.findall()
function instead of re.search()
:
matches = re.findall(r"'([^']*)'", mydata)
The re.findall()
function returns a list of all matches found.
Escaping Special Characters
If your substring contains special regex characters like (
, )
, [
, ]
, {
, }
, .
, *
, +
, ?
, ^
, $
, |
, \
, these characters need to be escaped with a backslash \
in your regex pattern. For example, to extract a substring containing parentheses, (like this)
, the regex pattern would be:
'\(([^']*)\)'
Your Regex Journey Continues! 🚀
Regex is a vast and powerful subject, and this guide only scratches the surface of its capabilities. The more you explore and practice with regex, the better you'll become at extracting substrings and solving text manipulation challenges.
Go forth, fellow regex adventurer! Unleash your regex magic and conquer all your substring extraction quests! And remember, if you have any questions or need assistance, leave a comment below. Let's regex our way to success! 💪💥
Call-to-Action: Share Your Regex Victories! 💬✨
Have you ever successfully used regex to extract a substring from a complex text? We'd love to hear about your regex victories and how they helped you in your coding journey! Share your experiences and join the regex conversation in the comments below. Let's learn and grow together! 🌟🗣️