How to escape comma and double quote at same time for CSV file?
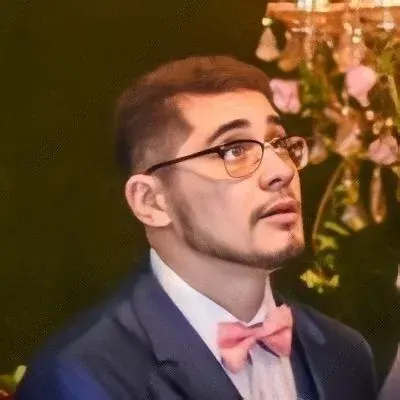
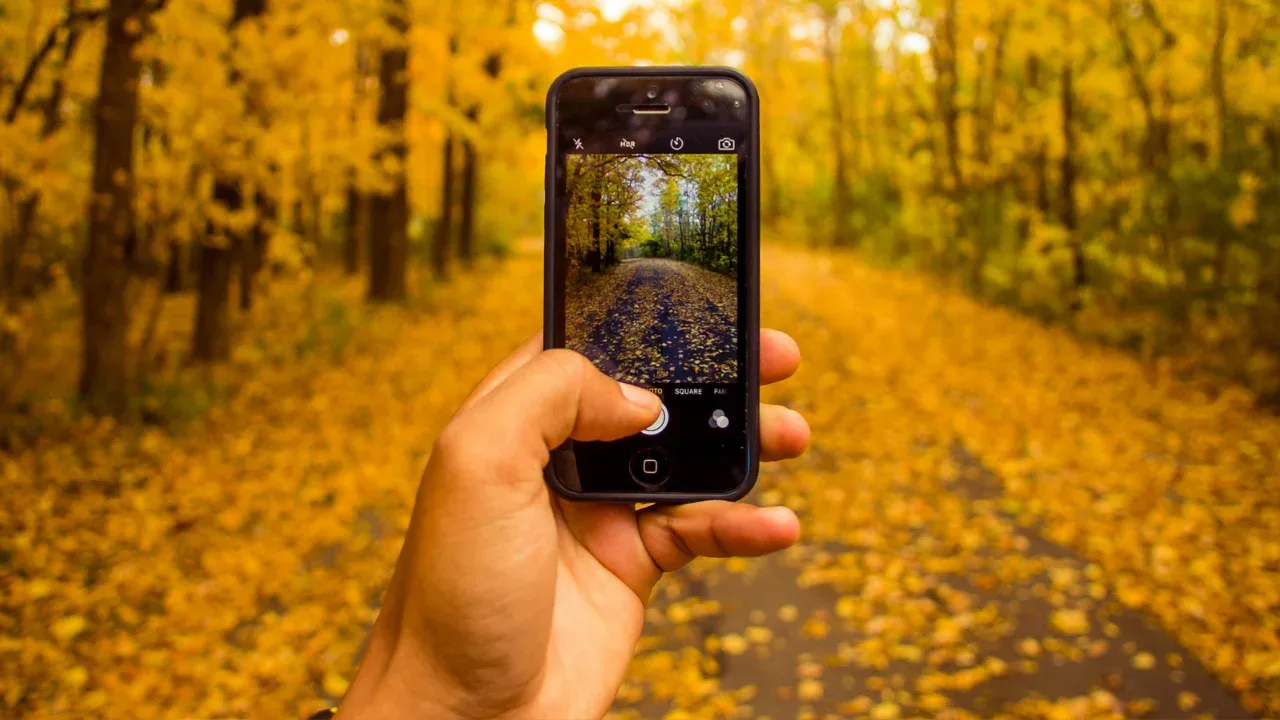
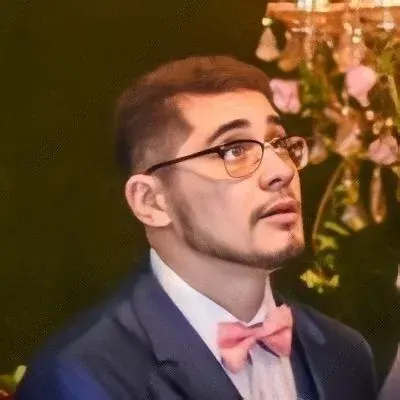
š Title: Escaping Commas and Double Quotes in a CSV File: A Java App Dilemma
š” Introduction Are you struggling with exporting data from Oracle to a CSV file using a Java app? Do you find it challenging to handle tricky data that includes commas, double quotes, and special characters? You're not alone! In this comprehensive guide, we will address a common issue and provide easy solutions for escaping commas and double quotes simultaneously in a CSV file. So hold tight and let's dive in! šŖ
š” Understanding the Problem Let's consider an example where the data contains a comment column that includes both commas and double quotes. Here's what it looks like:
| ID | FN | LN | AGE | COMMENT |
|----------------------------------------------------------------|
| 123 | John | Smith | 39 | I said "Hey, I am 5'10"." |
|----------------------------------------------------------------|
Run-of-the-mill CSV formatting won't cut it here. We need to escape the quotes around the phrase "Hey, I am 5'10"." while ensuring the commas within the tuples are treated as regular data and not delimiters. Sounds challenging, right? Let's see how we can accomplish this using regular expressions! š©āš»
š” Solution: Using Regular Expressions Here's a step-by-step guide to escaping commas and double quotes simultaneously in a CSV file using regular expressions in Java:
Import the required Java packages:
import java.util.regex.Matcher; import java.util.regex.Pattern;
Define the regular expression pattern for matching commas within double quotes:
String regex = ",(?=(?:[^\"]*\"[^\"]*\")*[^\"]*$)";
Compile the regular expression pattern:
Pattern pattern = Pattern.compile(regex);
Iterate over each row of data and apply the regular expression:
String row = "| 123 | John | Smith | 39 | I said \"Hey, I am 5'10\".\" |"; Matcher matcher = pattern.matcher(row); String escapedRow = matcher.replaceAll("\\\\,");
The
escapedRow
variable now contains the modified row with escaped commas:| 123 | John | Smith | 39 | I said \"Hey, I am 5'10\".\" |
Write the modified row to the CSV file.
š Congratulations! You have successfully escaped commas and double quotes simultaneously in your CSV file using regular expressions in Java! š
š” Call-to-Action Now that you have learned an easy solution to this CSV escapade, it's time to apply it to your Java app and conquer those tricky data scenarios. Share your success stories in the comments below and let's keep the conversation going! Don't forget to share this blog post with your friends and colleagues who might benefit from it. Stay tuned for more tech tips and tricks! š