How to determine programmatically the current active profile using Spring boot
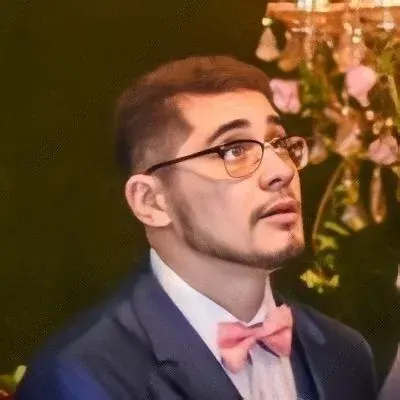
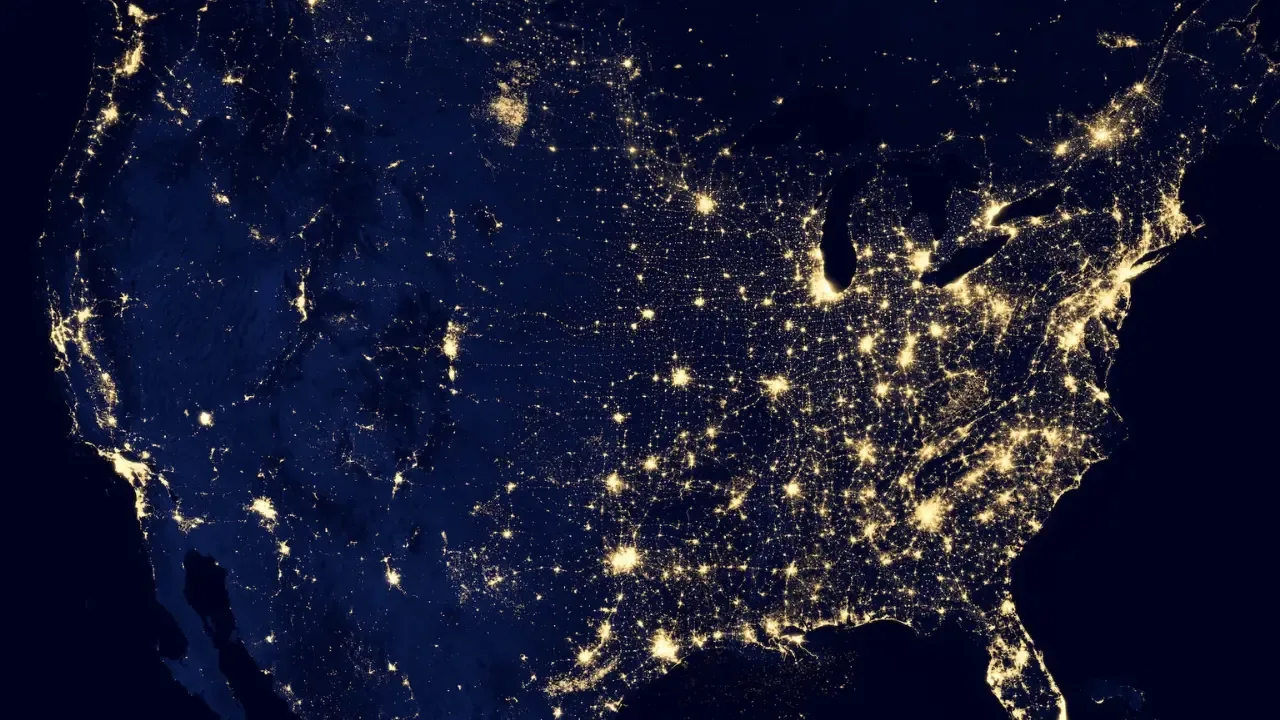
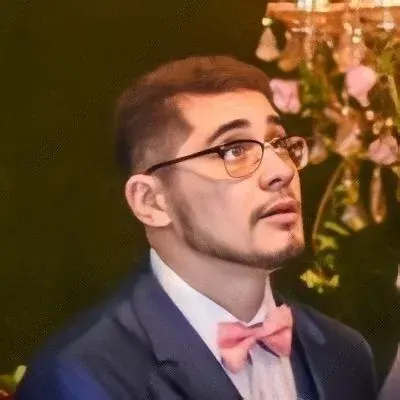
🌐 How to determine programmatically the current active profile using Spring Boot? 🤔
Hey there, tech enthusiasts! 👋 Are you using Spring Boot for your projects and wondering how to programmatically determine the current active profile? Well, you've come to the right place! 🎉 In this blog post, we'll explore a simple and efficient solution to this problem. So, let's get started! 🚀
🐣 Understanding the problem
The context of this question suggests that you want to retrieve the active profile within a bean programmatically. In Spring Boot, profiles allow you to configure different sets of beans based on the environment your application is running in. It's a powerful feature that helps you manage different configurations for development, testing, and production environments. 🌱
🚀 The Spring Environment class
To determine the current active profile programmatically, we can utilize the Spring Environment
class provided by Spring Framework. This class offers various methods to access and manipulate properties and profiles within your application. One such method is getActiveProfiles()
which returns an array of active profiles. 📚
Here's an example of how you can use Environment
to obtain the active profile within your bean:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.EnvironmentAware;
import org.springframework.core.env.Environment;
import org.springframework.stereotype.Component;
@Component
public class MyBean implements EnvironmentAware {
private Environment environment;
@Override
public void setEnvironment(Environment environment) {
this.environment = environment;
}
public void someMethod() {
String[] activeProfiles = environment.getActiveProfiles();
// Do something with the active profiles
}
}
In the above code snippet, we have a MyBean
class that implements the EnvironmentAware
interface. This interface allows us to get a reference to the Environment
object, which we can use to retrieve the active profiles.
🎉 Problem solved!
That's it! 🎉 By utilizing the Environment
class and implementing the EnvironmentAware
interface, you can easily determine the current active profile within your bean. Now you can customize your beans based on the profile and perform different actions accordingly.
💪 Take it to the next level!
Now that you know how to programmatically determine the active profile, why not explore other cool features offered by Spring Boot? Dive deeper into the world of profiles, configuration properties, and other powerful Spring Boot functionalities. 🚀
So go ahead, experiment, and create amazing applications with Spring Boot! And don't forget to share your experiences and thoughts in the comments below. We'd love to hear from you! 😊
Happy coding! 💻🌈