How to create RecyclerView with multiple view types
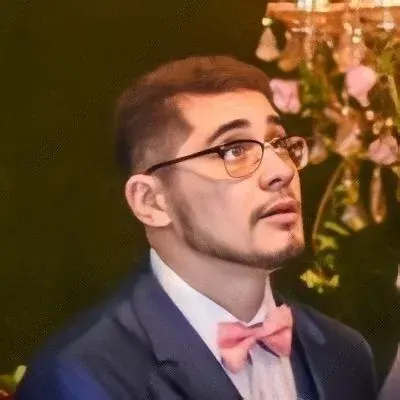
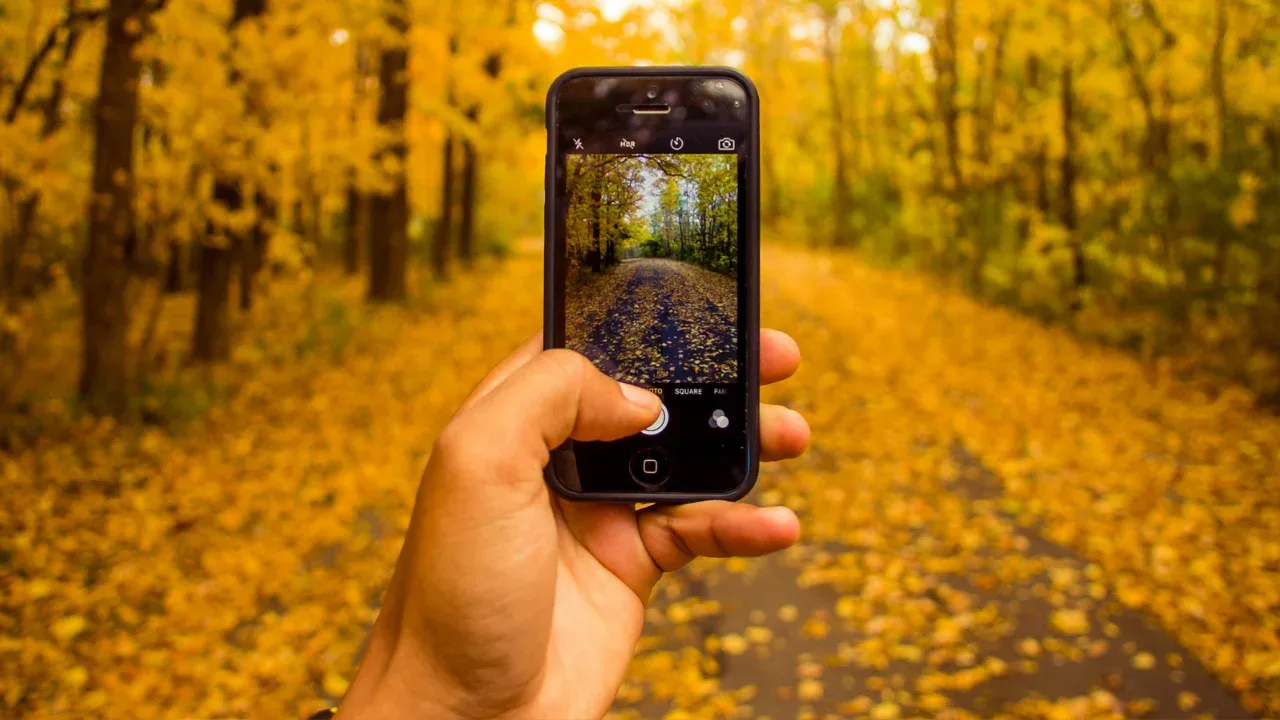
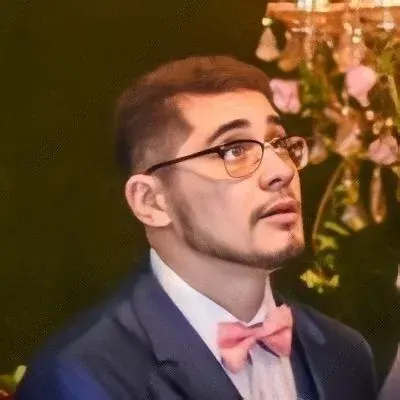
How to Create RecyclerView with Multiple View Types 🔄📋
So you want to create a RecyclerView with multiple view types? You've come to the right place! 🙌 In this blog post, we'll dive into the process of setting up a RecyclerView that supports different view types, addressing common issues and providing simple solutions along the way. Let's get started! 🚀
The Challenge 😅
By default, when creating a RecyclerView, you specify a single ViewHolder that binds with the adapter. But what if you want to display different types of data or layouts within the same RecyclerView? That's when things start to get interesting. 😏
The Solution 💡
To create a RecyclerView with multiple view types, you'll need to override the getItemViewType
method in your adapter. This method determines the view type based on the position of the item in the dataset. Here's an example implementation:
@Override
public int getItemViewType(int position) {
// logic to determine the view type based on the position
return viewType;
}
In the above code snippet, you'll need to replace viewType
with your own logic to determine the view type for the given position. Once you have the view type, you can use it in the onCreateViewHolder
method to inflate the appropriate layout file:
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view;
// inflate different layout files based on the view type
if (viewType == VIEW_TYPE_ONE) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.layout_type_one, parent, false);
} else if (viewType == VIEW_TYPE_TWO) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.layout_type_two, parent, false);
} else {
// handle other view types...
view = ...;
}
return new ViewHolder(view);
}
Make sure to replace VIEW_TYPE_ONE
and VIEW_TYPE_TWO
with your own constants representing the different view types in your RecyclerView.
Finally, in the onBindViewHolder
method, you can customize the view binding logic based on the view type:
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
int viewType = getItemViewType(position);
// bind data to the appropriate views based on the view type
if (viewType == VIEW_TYPE_ONE) {
MyDataTypeOne data = dataset.get(position);
// bind data to views for type one
} else if (viewType == VIEW_TYPE_TWO) {
MyDataTypeTwo data = dataset.get(position);
// bind data to views for type two
} else {
// handle other view types...
}
}
Remember to replace MyDataTypeOne
and MyDataTypeTwo
with your own data classes representing the different types of data in your RecyclerView.
Example 👀
To better understand how this works, let's take a look at an example. Suppose we have a RecyclerView that displays a list of items, where some items are regular text and others are images. We can define two view types (VIEW_TYPE_TEXT
and VIEW_TYPE_IMAGE
) to differentiate between these two types of items.
Here's an example implementation of the adapter:
public class MyAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private List<Object> dataset;
// other necessary constructor and methods...
@Override
public int getItemViewType(int position) {
Object item = dataset.get(position);
if (item instanceof String) {
return VIEW_TYPE_TEXT;
} else if (item instanceof Integer) {
return VIEW_TYPE_IMAGE;
}
return super.getItemViewType(position);
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view;
if (viewType == VIEW_TYPE_TEXT) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_text, parent, false);
return new TextViewHolder(view);
} else if (viewType == VIEW_TYPE_IMAGE) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_image, parent, false);
return new ImageViewHolder(view);
}
throw new IllegalArgumentException("Unsupported view type: " + viewType);
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
int viewType = getItemViewType(position);
if (viewType == VIEW_TYPE_TEXT) {
String text = (String) dataset.get(position);
((TextViewHolder) holder).bind(text);
} else if (viewType == VIEW_TYPE_IMAGE) {
int imageRes = (int) dataset.get(position);
((ImageViewHolder) holder).bind(imageRes);
}
}
// ViewHolder implementations...
private static class TextViewHolder extends RecyclerView.ViewHolder {
private TextView textView;
TextViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(R.id.text_view);
}
void bind(String text) {
textView.setText(text);
}
}
private static class ImageViewHolder extends RecyclerView.ViewHolder {
private ImageView imageView;
ImageViewHolder(View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.image_view);
}
void bind(int imageRes) {
imageView.setImageResource(imageRes);
}
}
}
In this example, we define two view types (VIEW_TYPE_TEXT
and VIEW_TYPE_IMAGE
) based on the instance types of the items in the dataset. We then inflate the appropriate layout files and bind the data accordingly in the onCreateViewHolder
and onBindViewHolder
methods.
Your Turn! 🚀
Now that you know how to create a RecyclerView with multiple view types, it's time to put your learning into action! Start by implementing this approach in your own projects and explore the endless possibilities it offers. Experiment with different layouts and data types to create a dynamic and engaging user experience. 🎉
If you have any questions or face any challenges along the way, feel free to leave a comment below. I'm here to help you out! 😊
Happy coding! 💻✨