How to create a generic array in Java?
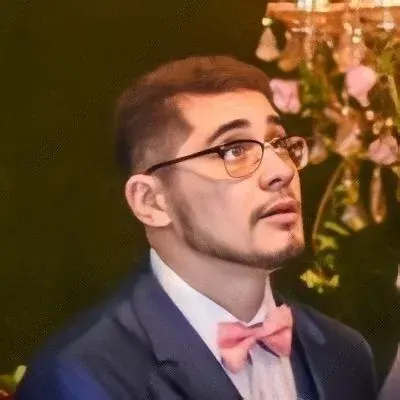
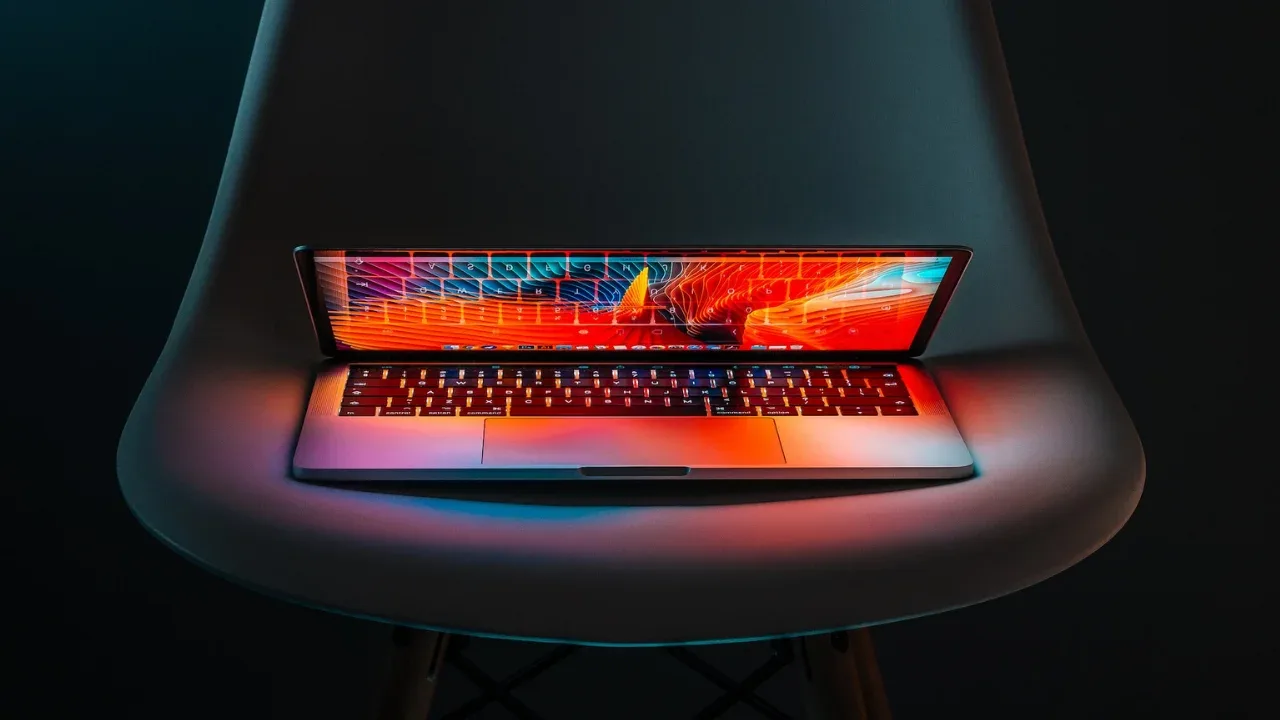
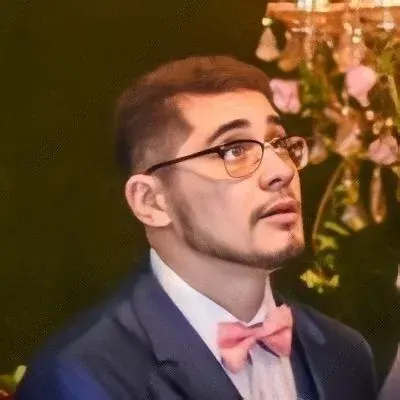
How to Create a Generic Array in Java 🤔
Do you ever come across a situation where you need to create a generic array in Java, but you find yourself scratching your head because of the limitations imposed by Java generics? 🤷♂️ Don't worry! In this blog post, I'll break down the problem, provide you with an easy solution, and also give you a helpful explanation of what's happening under the hood. Let's dive in! 💪
The Problem ❌
Let's say you have a class called GenSet
that needs to store an array of elements of a generic type E
. You might be tempted to create an array like this:
public class GenSet<E> {
private E a[];
public GenSet() {
a = new E[INITIAL_ARRAY_LENGTH]; // error: generic array creation
}
}
Unfortunately, Java doesn't allow you to create generic arrays directly due to the way generics are implemented. 😕 This leads to a compilation error, leaving you puzzled about how to proceed.
The Solution ✅
Luckily, there is a way to create a generic array in Java while maintaining type safety. Here's a solution inspired by the Java forums:
import java.lang.reflect.Array;
class Stack<T> {
private final T[] array;
public Stack(Class<T> clazz, int capacity) {
array = (T[]) Array.newInstance(clazz, capacity);
}
}
Breaking Down the Solution 🔍
At first glance, the solution might seem a bit confusing. Let me explain what's happening here in simple terms. 🧐
We import the
Array
class from thejava.lang.reflect
package. This class provides useful methods for dynamically creating arrays at runtime.In the
Stack
class, we declarearray
as a private instance variable of typeT[]
. This will be our generic array.In the constructor, we pass
Class<T>
andcapacity
as parameters. TheClass<T>
parameter is used to determine the component type of the array we want to create.We use the
Array.newInstance(clazz, capacity)
method to create a new array of typeclazz
with the specifiedcapacity
. This method returns anObject
reference, so we need to cast it to the generic typeT[]
to make it compatible with our array.
And that's it! We now have a generic array array
of type T
that can be safely used within our Stack
class. 🎉
Conclusion and Call-to-Action 🎯
Creating a generic array in Java might seem like a daunting task, but with the help of the Array.newInstance()
method, you can overcome this limitation and maintain type safety in your code. 💪
I hope this guide has provided you with a clear understanding of how to create a generic array. If you still have doubts or need further clarification, don't hesitate to leave a comment below. I'll be more than happy to help you out! 😊
Now, it's your turn to put this knowledge into practice! Try implementing a generic array in your own project and see how it simplifies your code. Don't forget to share your experiences and any additional tips you discover along the way. Let's keep learning and growing together! 🌱
Happy coding! 👨💻