How to convert jsonString to JSONObject in Java
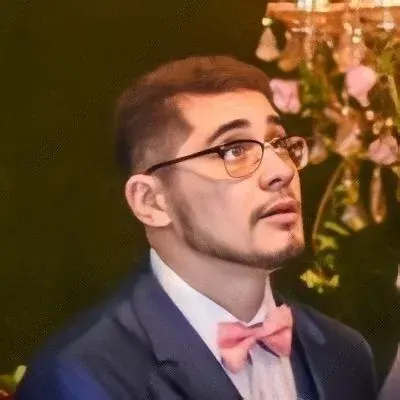
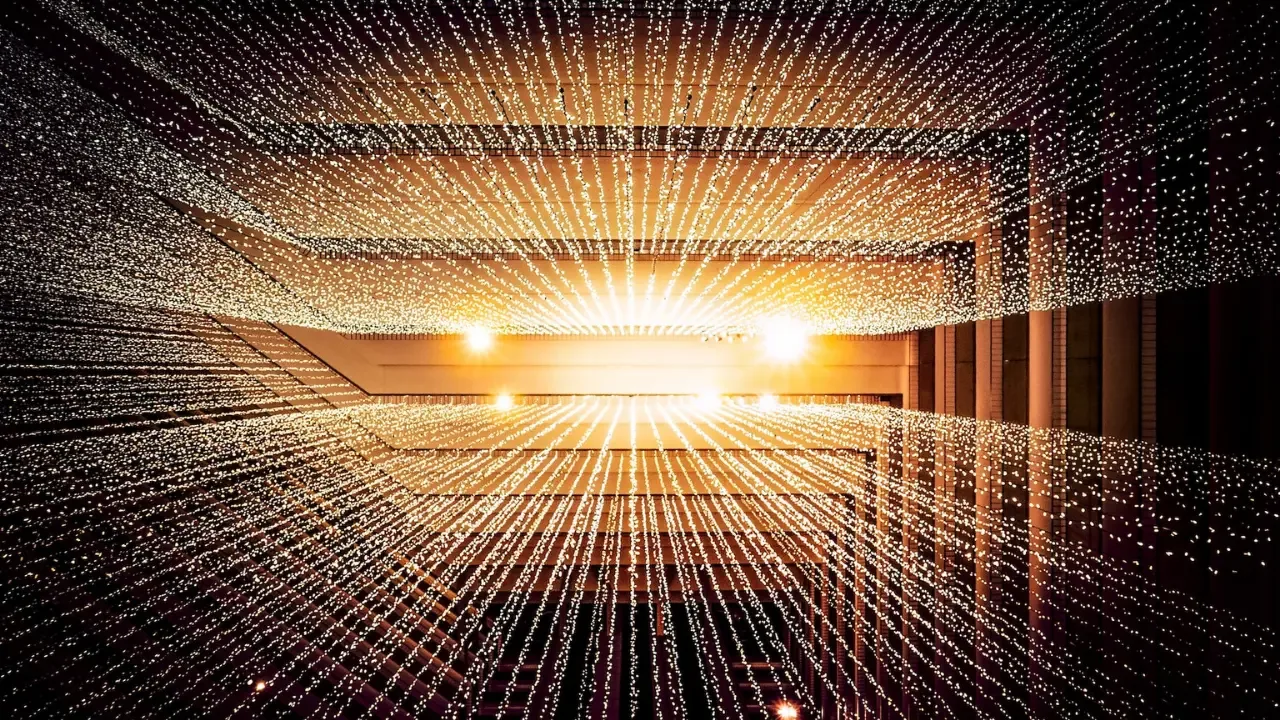
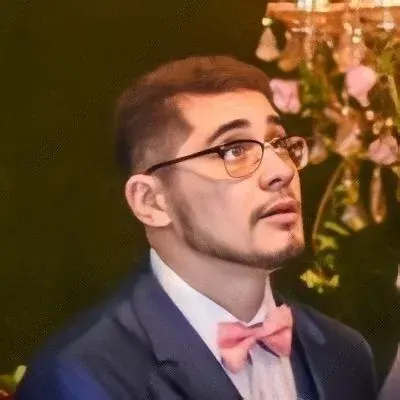
Convert jsonString to JSONObject in Java: A Simple Guide 👩💻📚
Are you struggling to convert a jsonString to a JSONObject in Java? 🤔 Don't worry, we've got you covered! In this post, we'll walk you through the common issues you might encounter and provide you with easy solutions to tackle this problem. Let's dive in! 🏊♀️🚀
Understanding the Problem
So, you have a String variable called jsonString
that contains a JSON object:
String jsonString = "{ \"phonetype\": \"N95\", \"cat\": \"WP\" }";
And now, you want to convert it into a JSON object. However, your online search for a solution didn't yield the expected results. 😞
The Solution: Import Libraries 📚
To convert the jsonString
into a JSONObject, we need to make use of the org.json
library. If you haven't already, you'll need to import the library into your project. You can do this by adding the following dependency to your pom.xml
file if you're using Maven:
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20210307</version>
</dependency>
Or if you're using Gradle, add this line to your build.gradle
file:
implementation 'org.json:json:20210307'
Make sure to sync your project dependencies before proceeding! ⚙️💪
Converting jsonString to JSONObject
With the necessary library imported, you can now convert the jsonString
to a JSONObject
using the following code:
import org.json.JSONObject;
public class JsonConverter {
public static void main(String[] args) {
String jsonString = "{ \"phonetype\": \"N95\", \"cat\": \"WP\" }";
JSONObject jsonObject = new JSONObject(jsonString);
// Now you can work with the JSONObject
// ...
}
}
That's it! You now have your jsonString
converted into a JSONObject
. You can access its properties, perform manipulations, and do whatever you need to do with the JSON object. 🎉🙌
Handling Common Issues
1. "NoClassDefFoundError: org/json/JSONObject"
If you encounter this issue, it means that the json
library has not been properly imported into your project. Double-check your dependencies, sync your project, and make sure the library is available in your classpath.
2. Malformed JSON String
If your jsonString
is not properly formatted, you may encounter a JSONException
. Ensure that your JSON string adheres to the correct syntax. A handy resource to validate your JSON is https://jsonlint.com/. Remember to escape special characters when necessary.
Share Your Thoughts and Experiences! 📢💬
We hope this guide helped you successfully convert a jsonString to a JSONObject in Java! If you have any further questions or insights, let us know in the comments below. 👇 We'd love to hear from you!
Happy coding! 💻🎉