How to convert Java String into byte[]?
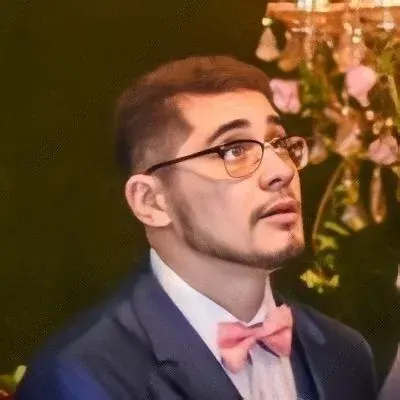
![Cover Image for How to convert Java String into byte[]?](https://images.ctfassets.net/4jrcdh2kutbq/1SyGC32MagR62gclzVUxTj/6b5fc3cceba5135aae98a5d7f1866772/Untitled_design__15_.webp?w=3840&q=75)
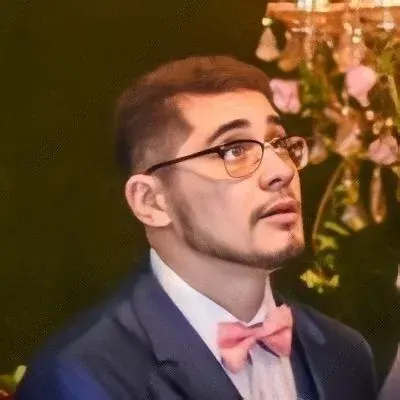
📝 How to Convert Java String into byte[]?
Are you struggling with the task of converting a Java String into a byte[]? You're not alone! Converting a String to a byte[] can be tricky, but fear not, we're here to help. In this blog post, we'll address common issues and provide easy solutions to help you accomplish this task seamlessly.
🔎 Understanding the Problem
Let's start by understanding the problem you encountered. You mentioned that you tried splitting the response using "\r\n\r\n"
and then converting the resulting substring into a byte[] using getBytes()
. However, the output you received was not as expected.
Here's the code snippet you used:
System.out.println(response.split("\r\n\r\n")[1]);
System.out.println("******");
System.out.println(response.split("\r\n\r\n")[1].getBytes().toString());
And the corresponding output:
<A Gzip String>
******
[B@38ee9f13
The first output is a gzip string, but you couldn't display it properly, while the second output is an address. So, what went wrong?
🤔 Identifying the Issue
The issue lies in your usage of getBytes()
. When you call toString()
on the byte[] returned by getBytes()
, it doesn't give you the actual content of the byte[]. Instead, it gives you the default representation, which includes the object's address and type.
Therefore, the line response.split("\r\n\r\n")[1].getBytes().toString()
is not the correct way to convert a Java String into a byte[].
💡 The Solution
To convert a Java String into a byte[], you need to use the getBytes()
method without calling toString()
. Here's the revised code:
byte[] byteArray = response.split("\r\n\r\n")[1].getBytes();
With this code, you'll get the desired byte[] representation of your String.
🔧 Applying the Solution
Now that you have the byte[] representation of your String, let's move forward and address your specific requirement of feeding it to a gzip decompressor. Below is the code snippet you provided for the decompressor:
String decompressGZIP(byte[] gzip) throws IOException {
java.util.zip.Inflater inf = new java.util.zip.Inflater();
java.io.ByteArrayInputStream bytein = new java.io.ByteArrayInputStream(gzip);
java.util.zip.GZIPInputStream gzin = new java.util.zip.GZIPInputStream(bytein);
java.io.ByteArrayOutputStream byteout = new java.io.ByteArrayOutputStream();
int res = 0;
byte buf[] = new byte[1024];
while (res >= 0) {
res = gzin.read(buf, 0, buf.length);
if (res > 0) {
byteout.write(buf, 0, res);
}
}
byte uncompressed[] = byteout.toByteArray();
return (uncompressed.toString());
}
To use this decompressor with the byte[] you obtained earlier, you can simply call the method like this:
String decompressedString = decompressGZIP(byteArray);
The decompressedString
will now hold the uncompressed String obtained from the gzip data.
📢 Engaging with the Readers
Congratulations! You've successfully learned how to convert a Java String into a byte[]. Now, it's time for you to put this knowledge to use and explore further. Why not experiment with different Strings or try applying this technique in your own projects?
We'd love to hear about your experiences or any other questions you might have. Feel free to leave a comment below and join the conversation!
✨ In Summary
To convert a Java String into a byte[], remember the following steps:
Split the String based on your specific delimiter.
Retrieve the desired substring.
Use
.getBytes()
to obtain the byte[] representation.Avoid calling
.toString()
on the resulting byte[].
By following these steps, you'll be able to convert Java Strings into byte[] effortlessly.
So go ahead, take what you've learned, and convert with confidence! 🚀