How to convert hashmap to JSON object in Java
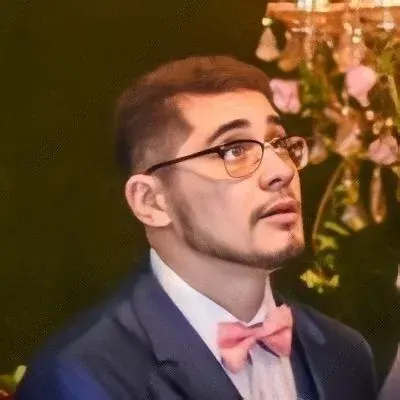
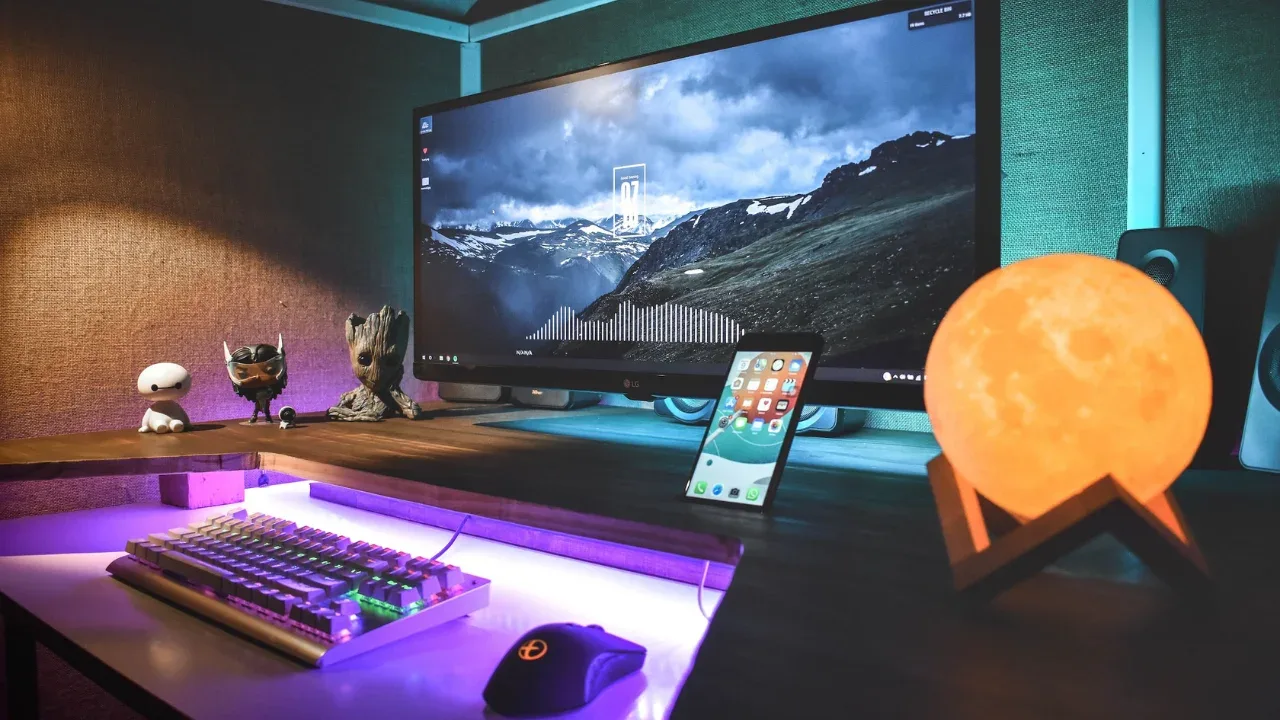
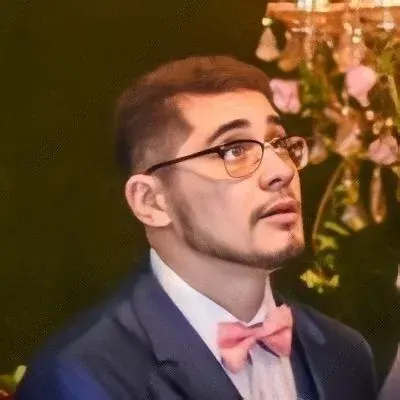
Converting Hashmap to JSON Object in Java: A Complete Guide 📚
If you've ever wondered how to convert a Hashmap to a JSON object in Java, you've come to the right place! 🤩 This guide will walk you through the process, step by step, so you can easily tackle this common coding challenge.
🔍 Let's start by understanding the problem:
The Dilemma: Converting Hashmap to JSON Object
Imagine you have a Hashmap in your Java code, and you need to convert it into a JSON object. Additionally, once you have the JSON object, you may want to convert it into a JSON string.
The Solution: JSON Libraries to the Rescue! 🚀
To convert a Hashmap to a JSON object in Java, we can utilize JSON libraries that provide handy methods and functionalities. Two popular libraries are Gson and Jackson. Let's explore both approaches:
Using Gson Library
First, ensure you have the Gson library added to your Java project. Add the following dependency to your
pom.xml
file if you're using Maven:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>{version}</version>
</dependency>
In your Java code, import the necessary Gson classes:
import com.google.gson.Gson;
import com.google.gson.JsonObject;
Now, let's convert the Hashmap to a JSON object using Gson:
HashMap<String, String> hashmap = new HashMap<>();
// Populate the hashmap with your desired key-value pairs
Gson gson = new Gson();
String json = gson.toJson(hashmap);
JsonObject jsonObject = gson.fromJson(json, JsonObject.class);
Using Jackson Library
Similar to the Gson approach, make sure you have the Jackson library added to your project. Add the following Maven dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>{version}</version>
</dependency>
Import Jackson's ObjectMapper class into your Java code:
import com.fasterxml.jackson.databind.ObjectMapper;
Convert the Hashmap to a JSON object using Jackson:
HashMap<String, String> hashmap = new HashMap<>();
// Populate the hashmap with your desired key-value pairs
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(hashmap);
JsonNode jsonNode = mapper.readTree(json);
That's it! You've Successfully Converted Hashmap to JSON Object 🎉
With the help of either Gson or Jackson, you can effortlessly convert your Hashmap to a JSON object in Java. Isn't that cool? 😎
Feel free to choose whichever library suits your preferences or project requirements. Both Gson and Jackson are powerful and widely used in the Java community.
However, as a good practice, ensure that you handle any exceptions that may occur during the conversion process, such as JsonProcessingException
, to ensure smooth execution of your code.
🌟 Your Turn: Share Your Experience! 🌟
Now that you know how to convert Hashmap to JSON object in Java, why not give it a try? Experiment with different scenarios, test the conversion process, and see it in action! 💡
If you have any questions, suggestions, or even tips to share with our tech community, feel free to leave a comment below. We'd love to hear from you! Let's learn and grow together! 🌱
Happy Coding! 💻🚀