How to convert comma-separated String to List?
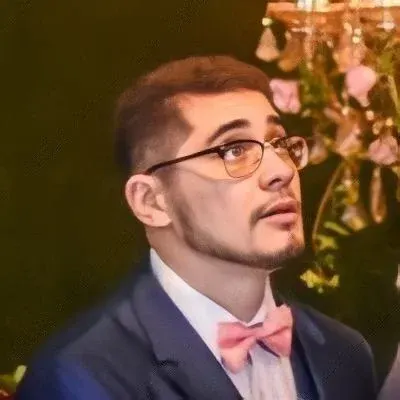
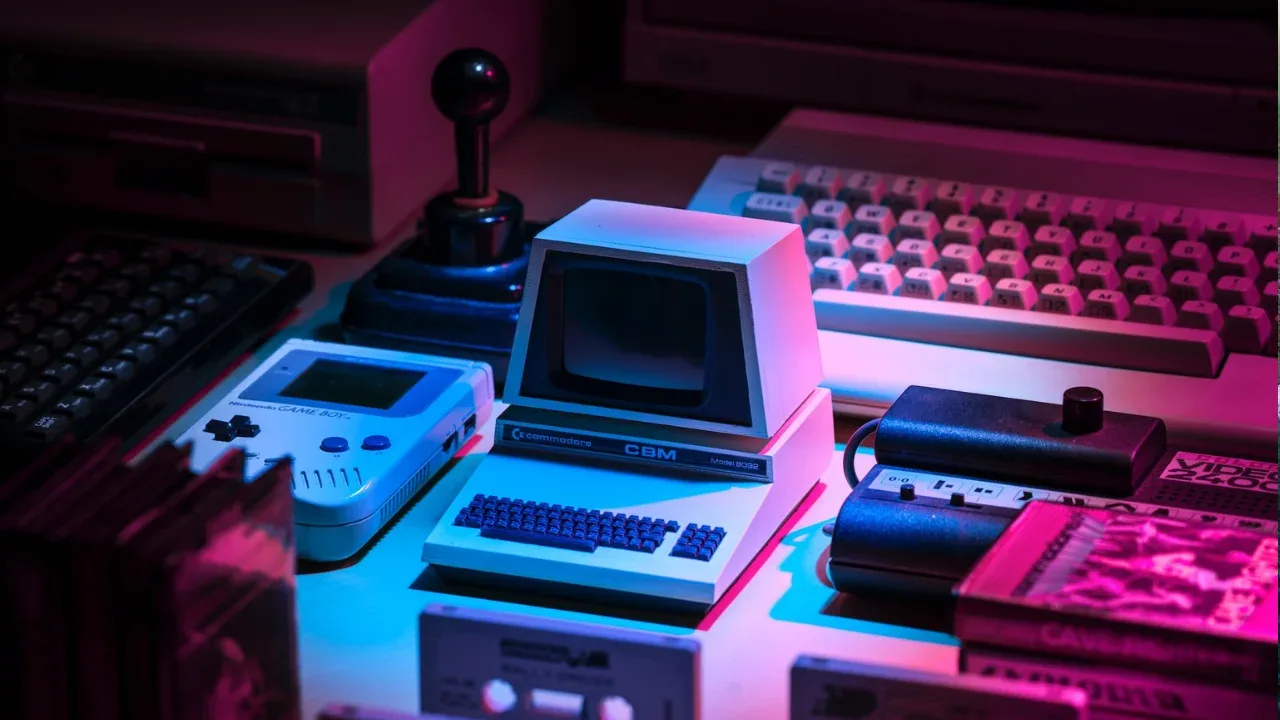
How to Convert Comma-Separated String to List in Java ๐๐ป
Are you struggling with converting a comma-separated string into a list in Java? Look no further! In this blog post, we will explore some easy solutions to your problem and guide you step by step. ๐ค๐
The Challenge ๐ค
Let's start with the challenge at hand. You have a comma-separated string, like "item1, item2, item3"
, and you want to convert it into a list. You might be wondering if there's a built-in method in Java for this or if you need to write custom code. ๐คทโโ๏ธ๐คทโโ๏ธ
The Solution ๐ก
Thankfully, Java provides a simple and elegant solution to convert a comma-separated string into a list using the Arrays.asList()
method. Let's take a look at how you can implement it in your code. ๐
String commaSeparated = "item1, item2, item3";
List<String> itemList = Arrays.asList(commaSeparated.split("\\s*,\\s*"));
First, we declare a string variable called
commaSeparated
and assign the comma-separated string to it. In our example, it is"item1, item2, item3"
.Next, we use the
split()
method to break the string into an array of substrings, using\\s*,\\s*
as the delimiter. This regular expression splits the string on a comma, while also accounting for potential white spaces before and after each item.Finally, we pass the resulting array into the
Arrays.asList()
method, which returns a fixed-size list containing the array elements. We assign this list to theitemList
variable.
And that's it! You have successfully converted the comma-separated string into a list. ๐๐ช
Common Issues and Pitfalls to Avoid โ
When dealing with comma-separated strings, there are a few common issues you might encounter. Let's address them and provide some tips to avoid any potential pitfalls. ๐งโ
1. Trailing or Leading Whitespace
Be careful with trailing or leading whitespace in the comma-separated string. If any items have extra spaces before or after them, they will be included in the resulting list. To handle this, we used the regular expression \\s*,\\s*
in the split()
method to trim the whitespace. ๐
2. Empty Items
If your string contains empty items (e.g., "item1, , item3"
), the resulting list will include an empty string. Depending on your use case, you might want to handle this separately by ignoring or removing empty items from the list.
3. Immutable List
Keep in mind that the list created using Arrays.asList()
is immutable, meaning you cannot add or remove elements from it. If you need a mutable list, create a new ArrayList
using the Arrays.asList()
result as the constructor argument.
Share Your Thoughts and Experiences! ๐ฌ๐ข
Now that you have the knowledge to convert a comma-separated string to a list, we would love to hear your thoughts and experiences! ๐ญโจ
If you have any questions, feedback, or interesting use cases, feel free to leave a comment below. Let's create a discussion and learn from each other! ๐ฃ๐ค
So go ahead, implement this solution in your code, and share your success story with us! Happy coding! ๐๐ป
Did you find this blog post helpful? Share it with your fellow developers and spread the knowledge! ๐๐
References ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
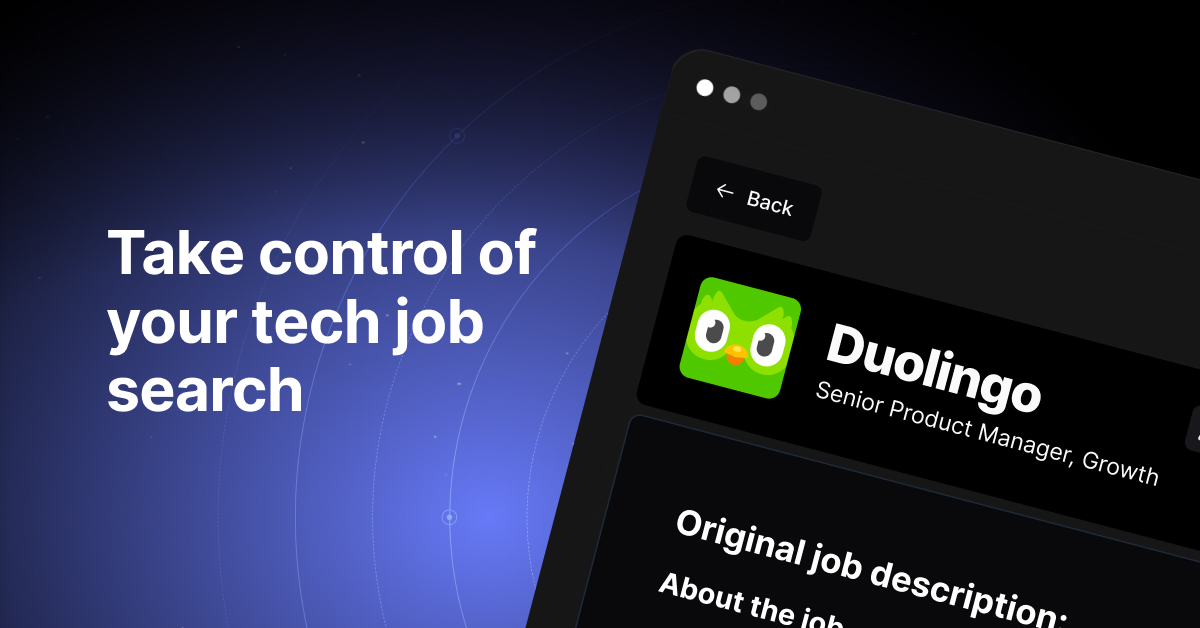