How to convert an Array to a Set in Java
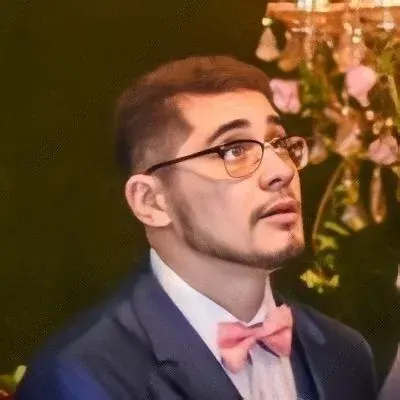
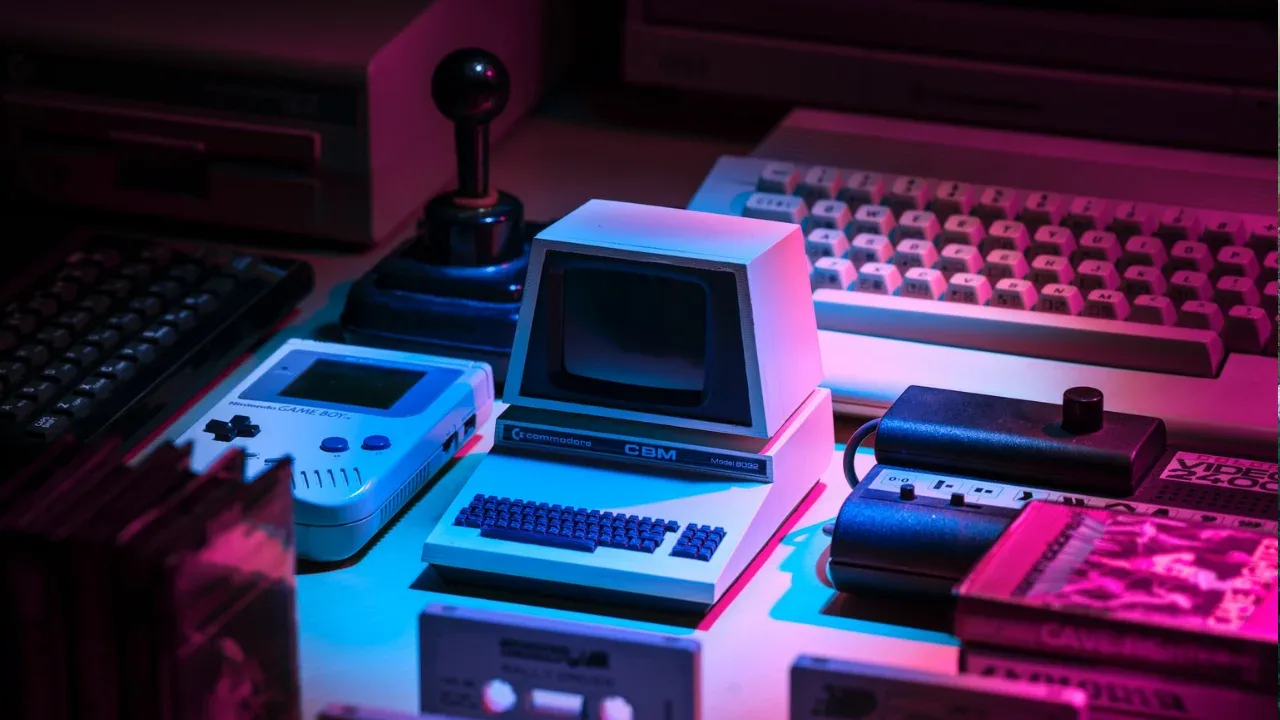
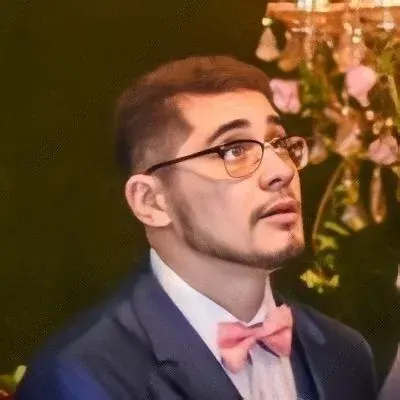
How to Convert an Array to a Set in Java 🔄
Have you ever faced the challenge of converting an array to a set in Java? 🤔 It may seem like a simple task at first, but finding an elegant and efficient solution can be quite tricky. Don't worry, though, we've got you covered! In this blog post, we'll explore various methods to convert an array to a set in Java, addressing common issues and providing easy solutions. Let's dive right in! 💪
The Problem 🕵️♀️
So, you have an array in Java and you want to convert it to a set. 🔄 Converting an array to a set is useful when you need to remove duplicate elements or perform set operations like intersection, union, or difference. While a simple solution could involve iterating over the array and adding each element to the set manually, this approach might not be the most efficient or elegant. 😕
The Neat Solution 💡
Luckily, Java provides a neat solution to convert an array to a set using the java.util.Arrays
class. 🌟 By utilizing the asList()
method from this class, we can seamlessly convert our array into a List, and then effortlessly create a Set from that List. 🎉 Here's the code snippet:
Object[] array = {1, 2, 3, 2, 4, 5};
Set<Object> set = new HashSet<>(Arrays.asList(array));
In this example, we have an array containing some elements, including duplicates. By applying Arrays.asList(array)
to our array, we convert it to a List. Then, by passing this List to the constructor of HashSet
, we instantly obtain a Set containing the unique elements. No need for manual iteration or additional libraries! 🙌
A Word of Caution ⚠️
While the above solution is concise and effective, it's crucial to note that it has some limitations. The Arrays.asList()
method actually returns a java.util.Arrays.ArrayList
, which is a fixed-size list backed by the original array. This means that modifying the resulting List will also affect the original array. Therefore, any modifications made to the Set obtained from this List will also affect the original array. Keep this in mind to avoid unexpected behavior! 😯
The Engaging Part 🎊
Now that you've learned an efficient way to convert an array to a set in Java, it's time to put that knowledge into practice! 🚀 Try converting your own arrays to sets using the method we described above. Experiment with different types of arrays, including arrays of objects or custom classes. Share your experiences, challenges, and any additional insights you've gained in the comments section below! Let's learn and grow together! 🌱
Conclusion 📚
Converting an array to a set in Java doesn't have to be complicated. By leveraging the Arrays.asList()
method and a simple constructor call, we can achieve our goal effortlessly. Just remember the potential side effects when modifying the resulting Set, and you're good to go! 💫
We hope this guide has shed some light on the topic and provided you with a practical solution. If you found this post helpful, don't hesitate to share it with your fellow developers. Together, we can simplify complex problems and make our coding lives more enjoyable! 🙌✨