How to convert a char array back to a string?
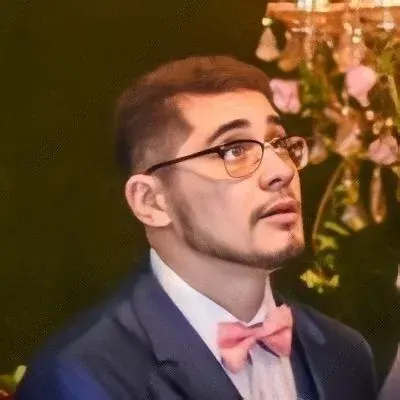
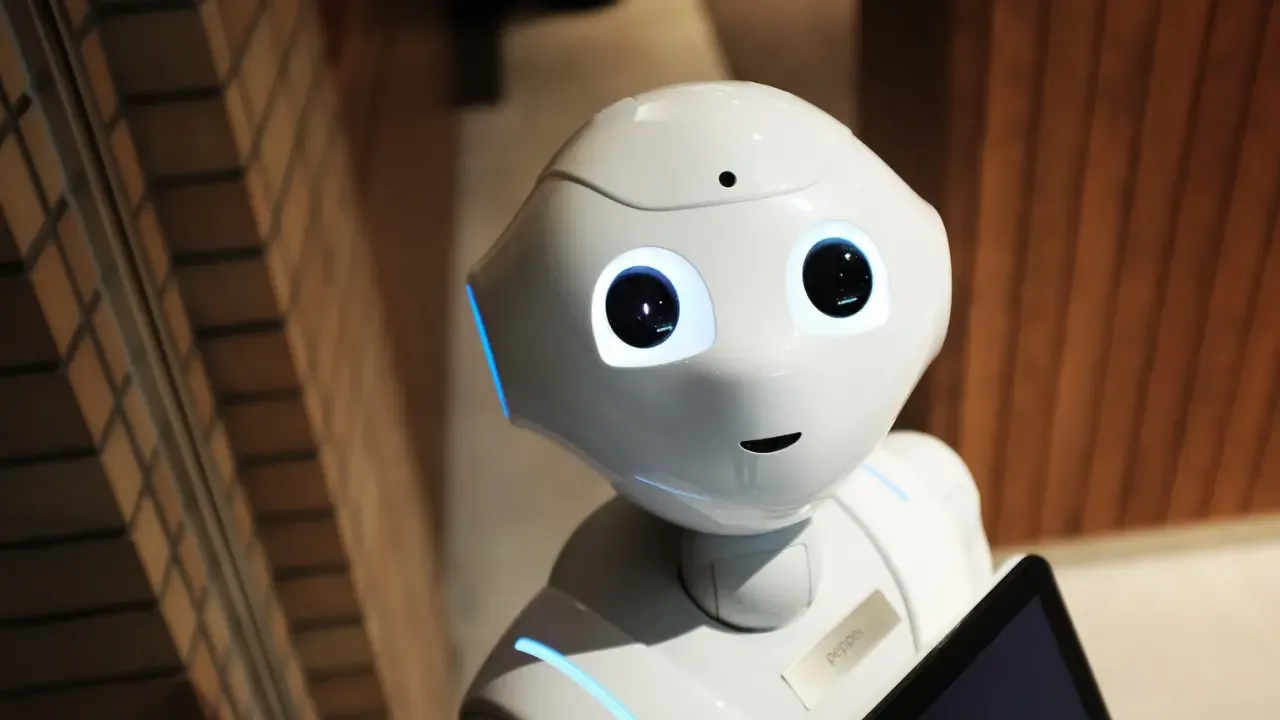
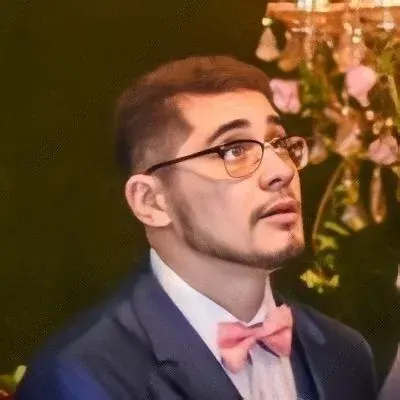
Title: 🔄 Converting a Char Array Back to a String: The Ultimate Guide 🎯
Introduction: Hey there tech enthusiasts! 😄 We're here today to tackle a common issue that developers often face: converting a char array back to a string. You know those times when you have a char array and need it to be in string form? We've got you covered! 💪
Problem: Let's dive right into it! So, you have a char array like this:
char[] a = {'h', 'e', 'l', 'l', 'o', ' ', 'w', 'o', 'r', 'l', 'd'};
And you are currently using the following solution:
String b = new String(a);
But deep down, you have that feeling that there must be a better way to accomplish this task.
Solution 1: Simple and Effective 🎉 Fortunately, you're right! There is a more concise way to convert that char array back to a string. Brace yourself for this game changer!
String b = String.valueOf(a);
Yes, it's that easy! By utilizing the String.valueOf()
method, you can directly convert your char array into a string with just one line of code. Say goodbye to unnecessary complexity! 👋
Solution 2: Use the String Constructor
Alternatively, if you prefer a more explicit approach, you can stick with the usage of the new String()
constructor. Although it might seem less concise, it provides the same result. Here's an example:
String b = new String(a);
Both solutions produce the desired outcome, so feel free to choose the one that resonates with you the most. 🤓
A Quick Explanation:
Under the hood, when you pass a char array to the String
constructor or the String.valueOf()
method, Java automatically converts it into a string, maintaining the order of the characters in the array. This conversion allows you to work with the char array seamlessly, just like a regular string.
Want to go further? Sure you do! 🔍
Common Issues and Troubleshooting:
Null or Empty Array: If you encounter a scenario where the char array is null or empty, both solutions will handle it gracefully. You won't face any unexpected behavior or errors.
char[] emptyArray = {}; // An empty array
String bEmpty = String.valueOf(emptyArray); // returns an empty string
Performance Considerations: When dealing with large char arrays, it's important to be mindful of performance. The solutions discussed herein are optimized and will work efficiently for most use cases. However, if your array contains an extremely high number of elements, it may impact performance. In that case, you could consider using a
StringBuilder
orStringBuffer
for better efficiency.
The Best Path Forward: Embrace Simplicity! 🚀 Now that you have learned two straightforward solutions to convert your char array back to a string, it's time to put that knowledge into practice! Experiment with these methods and decide which one makes your code more readable and maintainable. Remember, simplicity is your ally. 😎
Leave a comment below and let us know which solution clicked with you. Are there any additional tricks you've discovered? We'd love to hear from you and expand our tech knowledge! 💬
Keep coding, keep exploring, and until next time! Happy converting! 🎉✨