How to configure port for a Spring Boot application
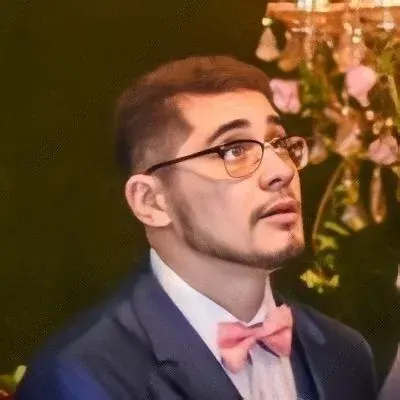
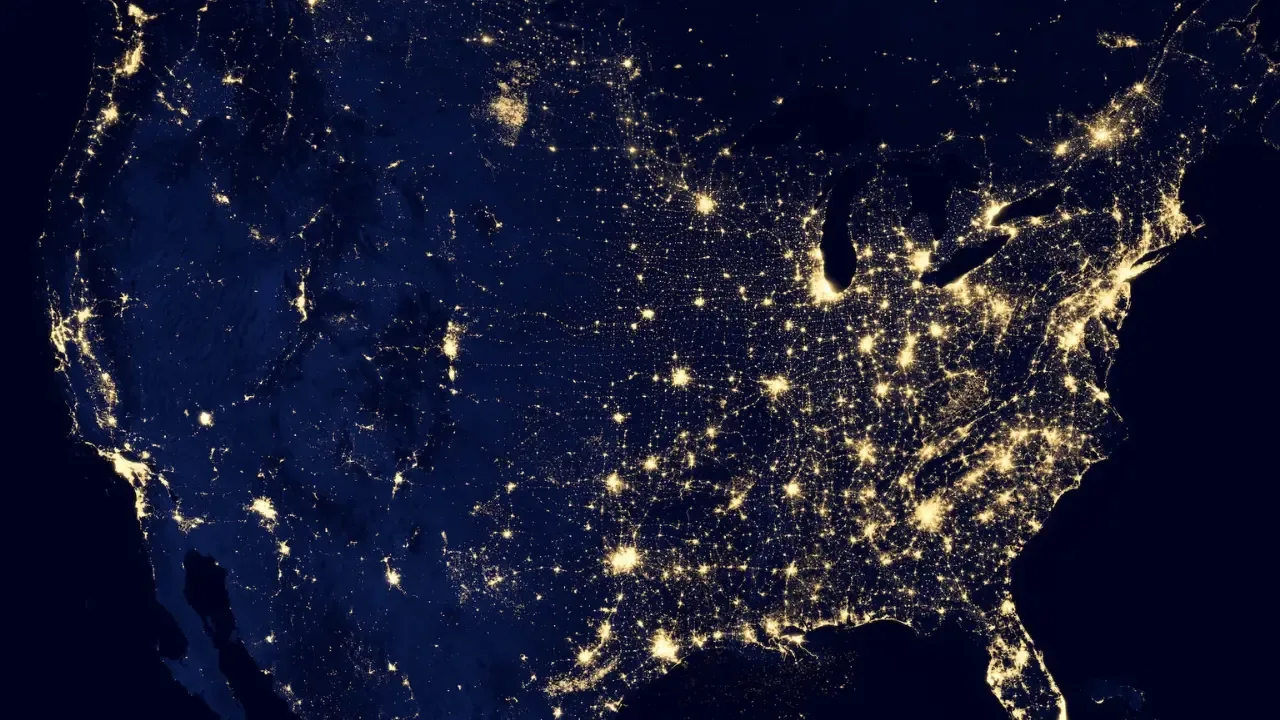
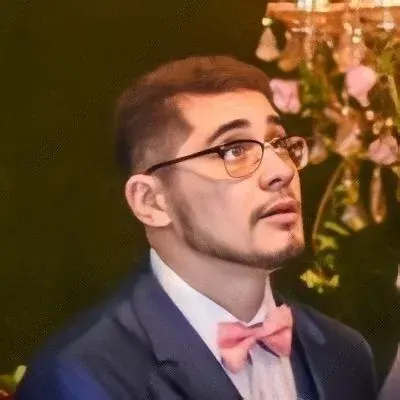
🌱 How to Configure Port for a Spring Boot Application
Are you tired of your Spring Boot application always using the default port of 8080? 🤔 Well, fear not! We're here to help you configure a different port and sail smoothly through your development process. ⚓
🛠️ Common Issues
One of the most common issues developers face is the need to change the default port. Typically, this arises when multiple applications are running simultaneously on the same machine, resulting in conflicts. 😫
🔑 The Solution
Configuring the port for your Spring Boot application can be achieved easily by modifying the application.properties
or application.yml
file. Let's dive into both options.
1. Using application.properties
Open the application.properties
file and add the following line:
server.port=YOUR_PORT_NUMBER
Replace YOUR_PORT_NUMBER
with the desired port, such as 8081
.
2. Using application.yml
If you prefer using application.yml
, add the following entry:
server:
port: YOUR_PORT_NUMBER
Again, replace YOUR_PORT_NUMBER
with your preferred port.
🐛 Example: Custom Port Configuration
Let's say you're tired of conflicting port 8080 and want to use port 8888 instead. Here's how you would do it in both application.properties
and application.yml
:
1. Using application.properties
Open application.properties
and add the following line:
server.port=8888
2. Using application.yml
If you prefer application.yml
, add the following entry:
server:
port: 8888
💡 Pro Tip
You can also configure the port directly in your code. For example, you can use the @Value
annotation in your main class to set the port programmatically:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class MyApp {
@Value("${server.port}")
private int port;
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@GetMapping("/")
public String hello() {
return "Hello from port " + port + "!";
}
}
This code snippet exemplifies how you can access the configured port value within your application.
📣 Let's Get Port-ing!
Congratulations! You've learned how to configure the port for your Spring Boot application. Now go ahead and make that change to glide smoothly through your development journey! 🚀
If you have any questions or face any issues during the process, feel free to leave a comment below. We'd love to help you out!
📢 Your Call to Action
If you found this blog post useful, share it with your developer friends who might be struggling with configuring their Spring Boot application ports. Let's spread the knowledge! 😄