How to check String in response body with mockMvc
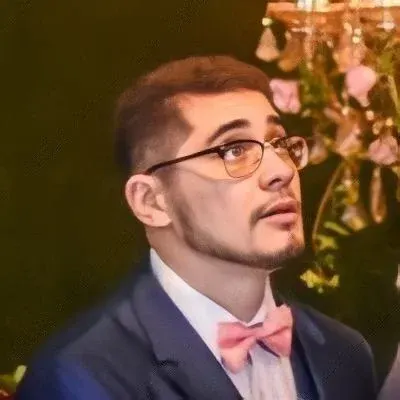

๐ How to check String in response body with mockMvc? ๐
So you're working on an integration test using mockMvc and you want to check if a specific string is present in the response body. You've tried a few things but haven't had any success. Don't worry, I'm here to help you out! Let's dive right in. ๐ช
The first thing we need to do is perform the request and store the response in a variable. In your case, it looks like you're already doing that with the mockMvc.perform(...)
method. This is great, we're off to a good start! ๐
Next, we need to extract the response body from the MockHttpServletResponse
object. This can be done using the getResponse().getContentAsString()
method. This will give us the response body as a string that we can work with. ๐
Now that we have the response body as a string, we can compare it to the expected string using any method or assertions of your choice. In this case, you want to check if the response body contains the string "Username already taken".
Here's an example of how you can achieve this:
andExpect(content().string(containsString("Username already taken")))
You can add this assertion right after the andExpect(status().isBadRequest())
assertion. This will check if the response body contains the expected string and pass the test if it does. โ
Here's how your updated test method will look like:
@Test
public void shouldReturnErrorMessageToAdminWhenCreatingUserWithUsedUserName() throws Exception {
mockMvc.perform(post("/api/users").header("Authorization", base64ForTestUser).contentType(MediaType.APPLICATION_JSON)
.content("{\"userName\":\"testUserDetails\",\"firstName\":\"xxx\",\"lastName\":\"xxx\",\"password\":\"xxx\"}"))
.andDo(print())
.andExpect(status().isBadRequest())
.andExpect(content().string(containsString("Username already taken")));
}
And that's it! You should now be able to check if a specific string is present in the response body using mockMvc. ๐
I hope this guide was helpful in solving your problem. If you have any further questions or need clarification, feel free to leave a comment below. Happy testing! ๐งช๐ป
Have you ever encountered a similar issue while testing with mockMvc? How did you solve it? Share your experiences in the comments below! โจโ๏ธ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
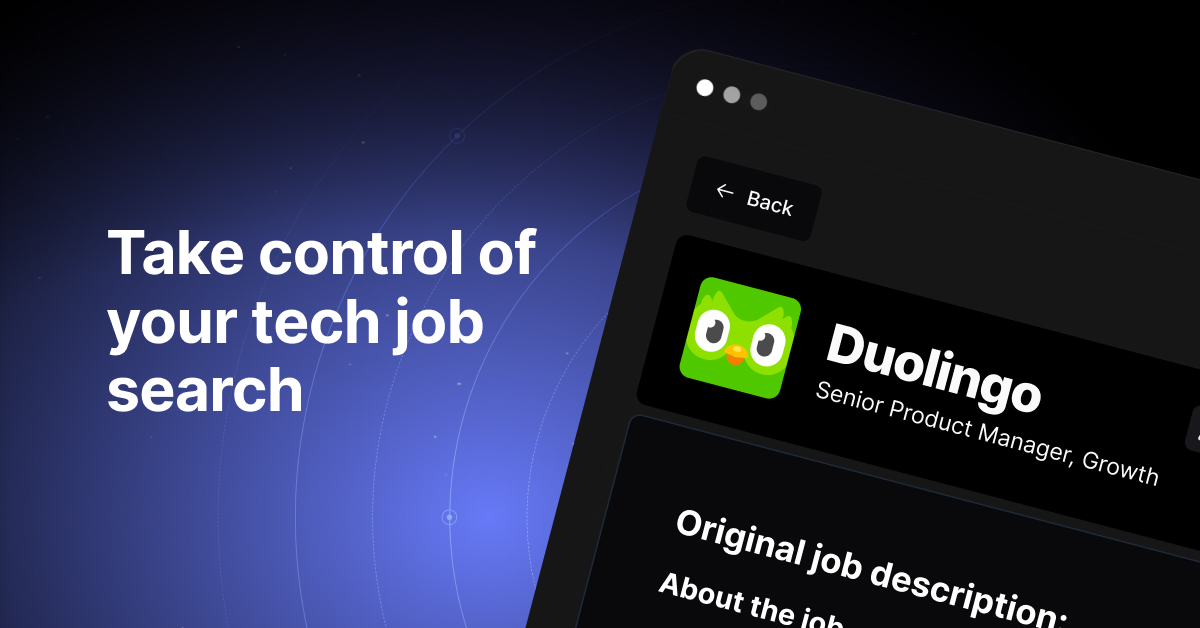