How to check if a String is numeric in Java
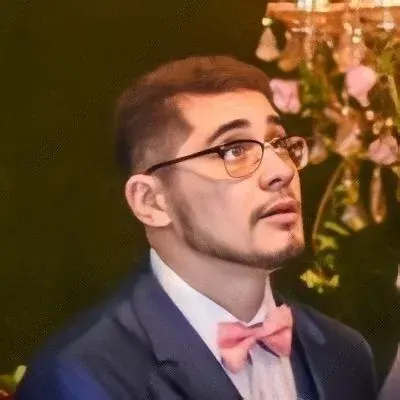
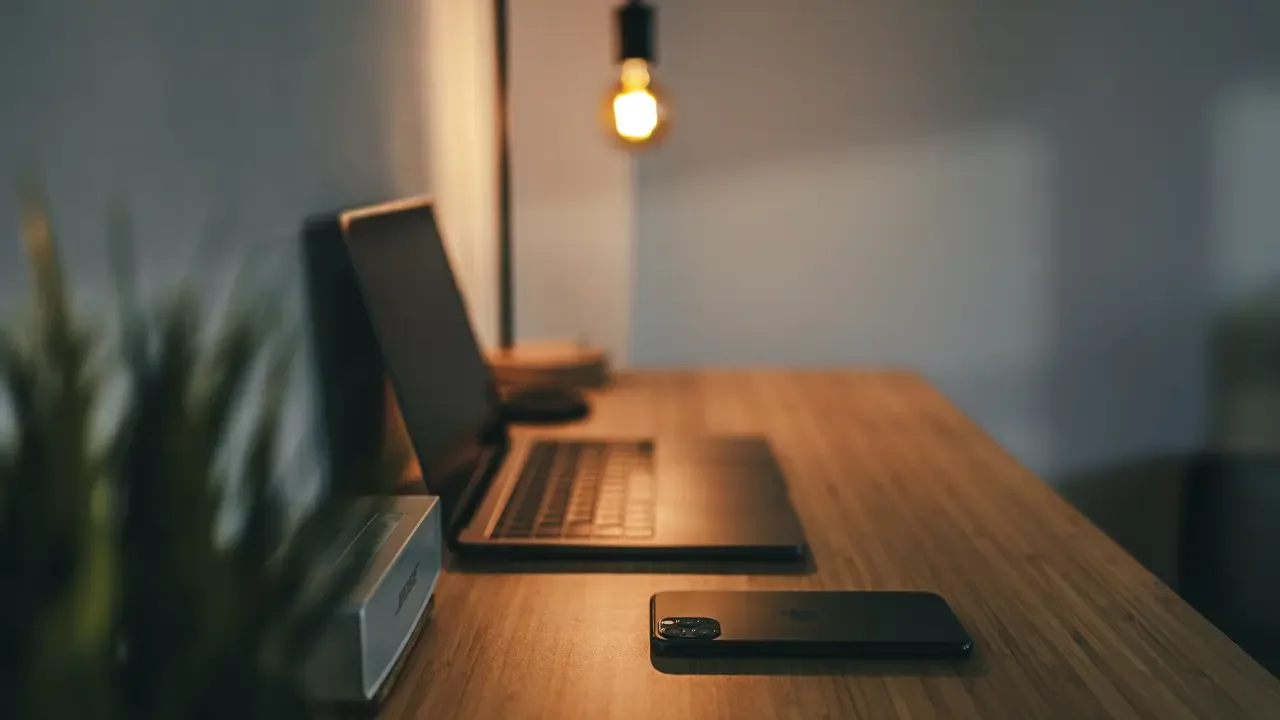
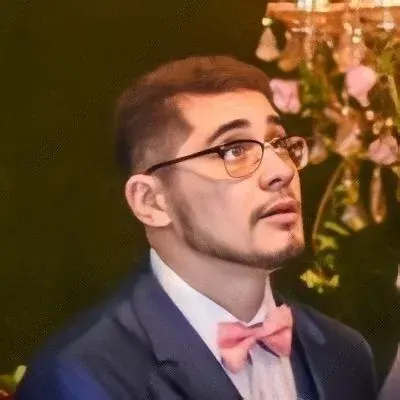
How to Check if a String is Numeric in Java: The Ultimate Guide! ๐๐ข
Have you ever been in a situation where you needed to determine whether a string represents a numeric value in Java? It can be quite a common issue, especially when dealing with user inputs or reading data from external sources. Fear not! In this blog post, we will explore some easy and practical solutions to this problem. Let's dive in!
The Challenge: Checking if a String is Numeric ๐ญโ
Before we jump into the solutions, let's quickly understand the problem at hand. The challenge is to identify whether a given string contains a valid numeric value before attempting to parse it. In other words, we want to avoid potential parsing exceptions by ensuring the string is numeric.
Solution 1: Using a Regex Pattern ๐งต๐
One of the simplest ways to check if a string is numeric is by using regular expressions (regex). We can define a pattern that matches numeric values and check if the given string matches this pattern. Here's how you can do it in Java:
public boolean isNumeric(String str) {
return str.matches("-?\\d+(\\.\\d+)?");
}
In the above code snippet, we use the matches()
method of the String
class, which internally uses a regex to match the entire string against the given pattern. The pattern "-?\\d+(\\.\\d+)?"
matches both integer and decimal numbers, allowing an optional negative sign ("-") and an optional decimal point followed by decimal places.
Solution 2: Using NumberFormatException ๐ฃโ
Another way to check if a string is numeric is by attempting to parse it as a number and catching any potential exceptions that might occur. In Java, the NumberFormatException
is thrown when a string cannot be parsed as a valid number. We can leverage this exception to determine if the string is numeric. Here's an example:
public boolean isNumeric(String str) {
try {
double number = Double.parseDouble(str);
return true;
} catch (NumberFormatException e) {
return false;
}
}
In the above code sample, we use the Double.parseDouble()
method to parse the string as a double value. If the parsing is successful, we can safely assume the string is numeric. However, if a NumberFormatException
is caught, it means the string is not numeric.
Solution 3: Using Apache Commons Lang ๐๐
If you are already using Apache Commons Lang library in your project, you can take advantage of their StringUtils
class, which provides helpful methods for string manipulation and analysis. One such method is isNumeric()
, which does exactly what we need. To use it, make sure you have the Apache Commons Lang library included in your project dependencies. Here's an example:
import org.apache.commons.lang3.StringUtils;
public boolean isNumeric(String str) {
return StringUtils.isNumeric(str);
}
In the above code snippet, we import the StringUtils
class from Apache Commons Lang and call the isNumeric()
method on our string. This method internally checks if each character of the string is a digit, ensuring that the string is numeric.
Wrapping Up and Encouraging Reader Engagement ๐๐ฃ
Congratulations! You now have multiple ways to check if a given string is numeric in Java. Whether you prefer regex, handling exceptions, or relying on a third-party library like Apache Commons Lang, you can confidently validate user inputs and prevent any pesky parsing errors.
Now that you have mastered this topic, why not share your newfound knowledge with your peers? Comment below and let us know which solution worked best for you or if you have any further questions. Happy coding! ๐ป๐๐
[Editor's Note: This blog post is part of our series "Java Gems" where we explore useful tips and tricks for Java development. Make sure to follow our blog for more Java goodness!]