How to call a method after a delay in Android
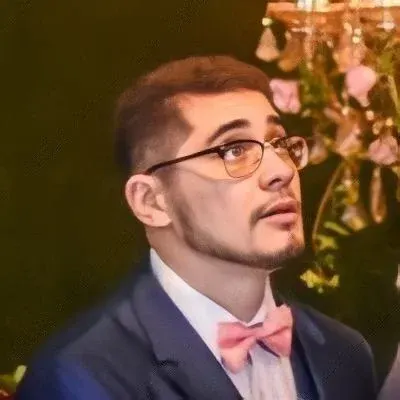

ππ± Calling a Method After a Delay in Android: Easy Solutions! ππ
Hey there, fellow Android developers! π Have you ever wondered how to call a method after a specific delay in Android, just like you can do in Objective C? π€ Well, you're in luck, because in this blog post, I'm going to address that common issue and provide you with easy solutions to achieve it in Java. π
β¨ The Objective C Equivalentβ¦
In Objective C, you can simply use the performSelector:withObject:afterDelay
method to call a method after a specified delay. But what about in Android? π€·ββοΈ
Fortunately, there's a similar concept in Android that allows you to achieve the same result. π
π Solution 1: Using Handler and Runnable
One way to call a method after a delay in Android is by using a Handler
and a Runnable
object.
Here's an example of how you can do that:
Handler handler = new Handler();
Runnable runnable = new Runnable() {
@Override
public void run() {
// Call your method here
doSomething();
}
};
handler.postDelayed(runnable, 5000); // Delay in milliseconds (5 seconds)
In this code snippet, we create a Handler
object and a Runnable
object. Inside the run
method of the Runnable
, you can call your desired method.
Finally, we use the postDelayed
method of the Handler
to schedule the execution of the Runnable
after the specified delay (in this case, 5000 milliseconds, which is equivalent to 5 seconds).
It's as simple as that! π
π Solution 2: Utilizing TimerTask
Another way to achieve the desired delay is by using a TimerTask
.
Here's how you can do it:
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
// Call your method here
doSomething();
}
}, 5000); // Delay in milliseconds (5 seconds)
In this approach, we create a Timer
object and schedule a TimerTask
. Inside the run
method of the TimerTask
, you can call your desired method.
By specifying the delay (in this case, 5000 milliseconds), the method will be executed after the specified time.
Voila! You've successfully called a method after a delay in Android. π
π‘ Pro tip: Remember to replace doSomething()
with the actual name of your method. π
Now that you know the solutions, go ahead and try them out in your Android project! π
π’ I want to hear from you!
Have you encountered this issue before? How did you handle it? Share your thoughts and experiences in the comments section below. Let's learn together! ππ¬
That's it for today's blog post. I hope you found it helpful and easy to understand. If you have any more questions or need further assistance, feel free to ask. Happy coding! ππ©βπ»π¨βπ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
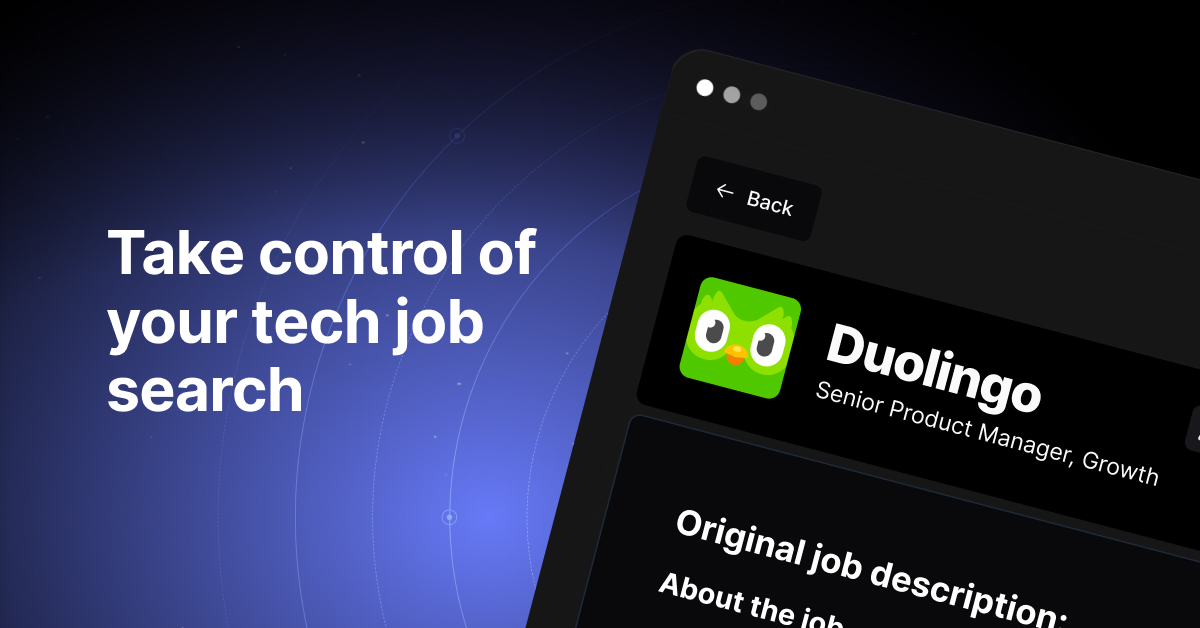