How to add a TextView to LinearLayout in Android
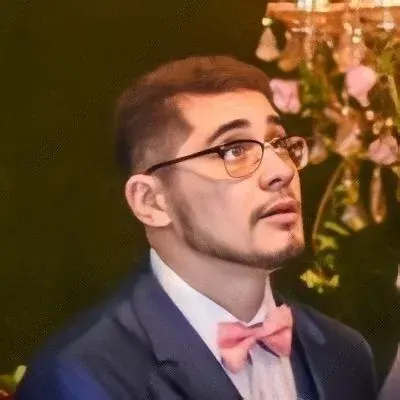
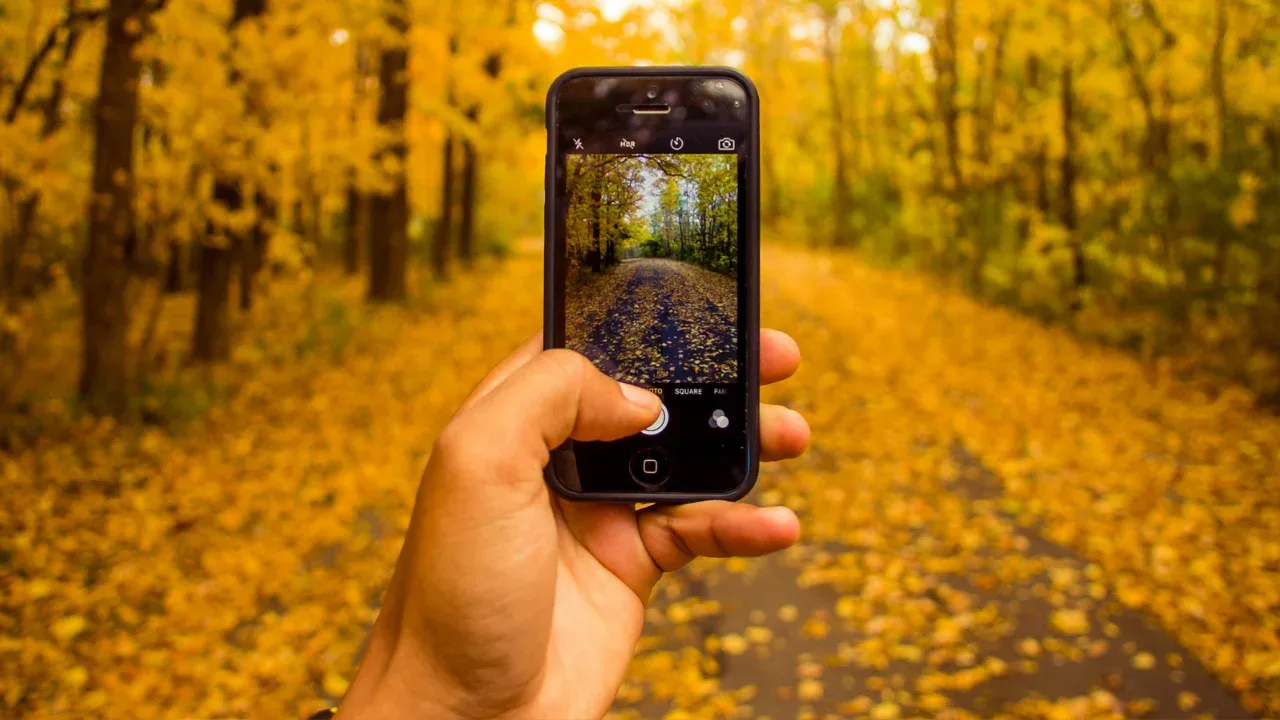
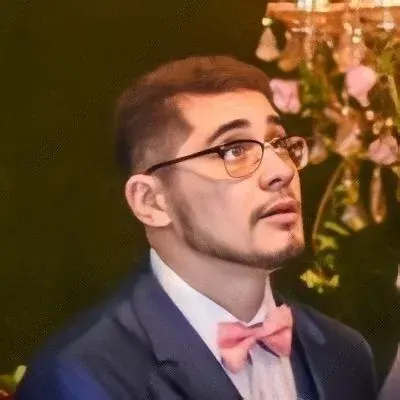
How to Add a TextView to LinearLayout in Android: A Beginner's Guide 👨💻
If you're new to Android development, you might find adding a TextView to a LinearLayout a bit challenging. Don't worry! We've got you covered. In this guide, we'll walk you through the process step by step, addressing common issues and providing easy solutions. 🔧💪
The Problem 😟
Imagine that you have an XML layout file with several Views defined, but you need to add some Views dynamically in your code. To achieve this, you create a LinearLayout in your XML layout file, like this:
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:id="@+id/info"
android:layout_height="wrap_content"
android:orientation="vertical">
</LinearLayout>
And in this layout, you want to add a TextView dynamically. So, in your code, you write the following:
View linearLayout = findViewById(R.id.info);
TextView valueTV = new TextView(this);
valueTV.setText("Hello, world!");
valueTV.setId(5);
valueTV.setLayoutParams(new LayoutParams(
LayoutParams.FILL_PARENT,
LayoutParams.WRAP_CONTENT));
((LinearLayout)linearLayout).addView(valueTV);
However, when you run your code, you encounter an error: java.lang.ClassCastException: android.widget.TextView
. 😓
The Solution ✅
To solve this issue, use the correct LayoutParams
class for your LinearLayout. Instead of using LinearLayout.LayoutParams
, use LinearLayout.LayoutParams
:
View linearLayout = findViewById(R.id.info);
TextView valueTV = new TextView(this);
valueTV.setText("Hello, world!");
valueTV.setId(5);
valueTV.setLayoutParams(new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.FILL_PARENT,
LinearLayout.LayoutParams.WRAP_CONTENT));
((LinearLayout)linearLayout).addView(valueTV);
By using LinearLayout.LayoutParams
, you ensure that the parameters are correctly assigned to your LinearLayout.
Conclusion and Call-to-Action 📝🔥
Adding a TextView to a LinearLayout in Android is now as easy as pie! 🍰 We hope this guide has helped you overcome the ClassCastException
error and add text dynamically to your layout. If you have any further questions or encounter any other issues, please let us know in the comments section below.
Go ahead and give it a try! 🚀 Add some awesome dynamic content to your Android app and let us know how it goes. Happy coding! 💻✨