How to accept Date params in a GET request to Spring MVC Controller?
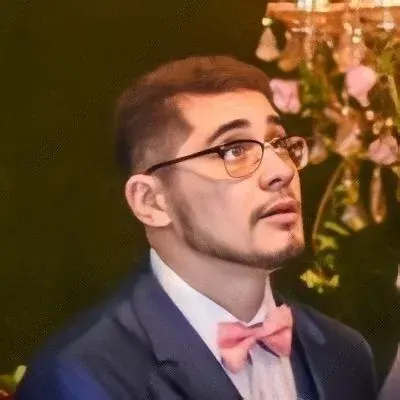
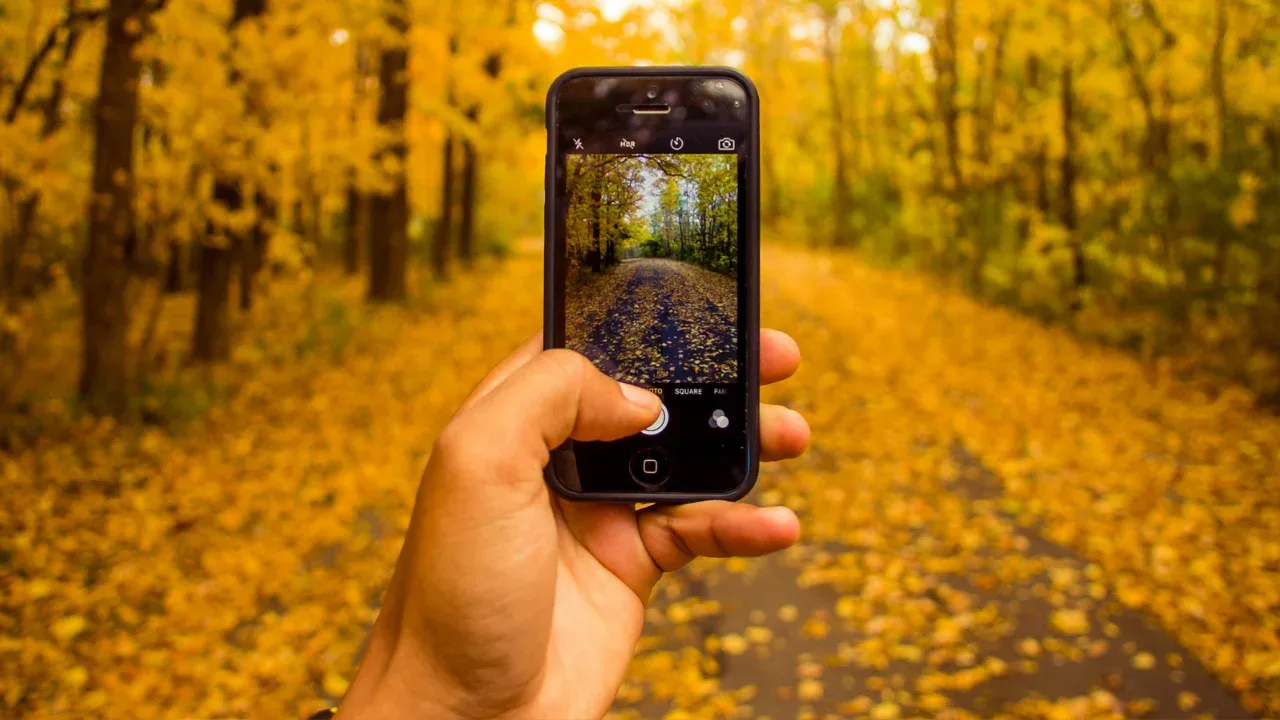
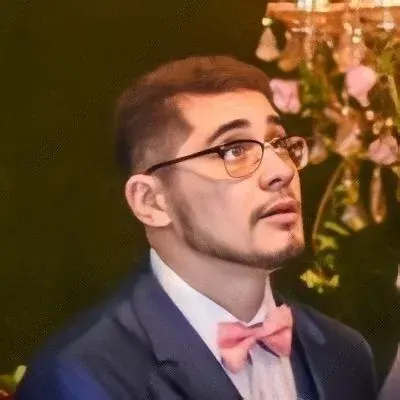
How to Accept Date Params in a GET Request to Spring MVC Controller? 📅
So, you're trying to send a date in the format YYYY-MM-DD
to your Spring MVC Controller via a GET request, but you're encountering an error, and you're not sure what you're doing wrong. Fear not! We'll help you fix this issue and get your controller to accept the date format you desire. Let's jump right into it! 💪
The Problem 🤔
Based on the code snippet you provided, it seems like you're using the @RequestParam
annotation to map the request parameter named "from" to a Date
object in your fetchResult
method. However, Spring is giving you a syntax error when you try to send the request with the date in the YYYY-MM-DD
format.
The Solution 💡
The issue with your current code lies in the fact that Spring's default behavior for binding request parameters to a Date
object is strict, meaning it expects the date to be in a specific format (MM/DD/YYYY
or MMM DD, YYYY
, depending on the locale).
To make your controller accept the YYYY-MM-DD
format, you can use a custom PropertyEditor
or Converter
in Spring. Let's walk through the steps to implement this solution:
Step 1: Create a Custom Converter
Create a class that implements the Converter
interface from Spring. Let's call it CustomDateConverter
. Here's an example implementation:
public class CustomDateConverter implements Converter<String, Date> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public Date convert(String source) {
try {
return dateFormat.parse(source);
} catch (ParseException e) {
throw new IllegalArgumentException("Invalid date format. Please use YYYY-MM-DD.", e);
}
}
}
Step 2: Register the Custom Converter
Next, you need to register your custom converter with Spring. There are a few ways to do this, but for simplicity, we'll use the WebMvcConfigurer
interface to configure our converters. Here's an example:
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addFormatters(FormatterRegistry registry) {
registry.addConverter(new CustomDateConverter());
}
}
Step 3: Test It Out! 🎉
That's it! You've created a custom converter and registered it with Spring. Now, you can try sending your GET request with the YYYY-MM-DD
date format, and your controller should be able to accept it without any issues.
Conclusion 🚀
In this guide, we've addressed the common issue of accepting Date
params in a GET request to a Spring MVC Controller. By creating a custom converter and registering it with Spring, you can effortlessly handle date formats outside of the default expectations.
We hope this guide has been helpful in resolving your issue. If you have any further questions or need clarification, please feel free to leave a comment below. Happy coding! 😄👩💻👨💻