How does the Java "for each" loop work?
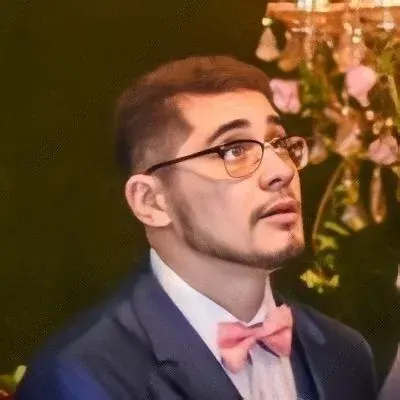
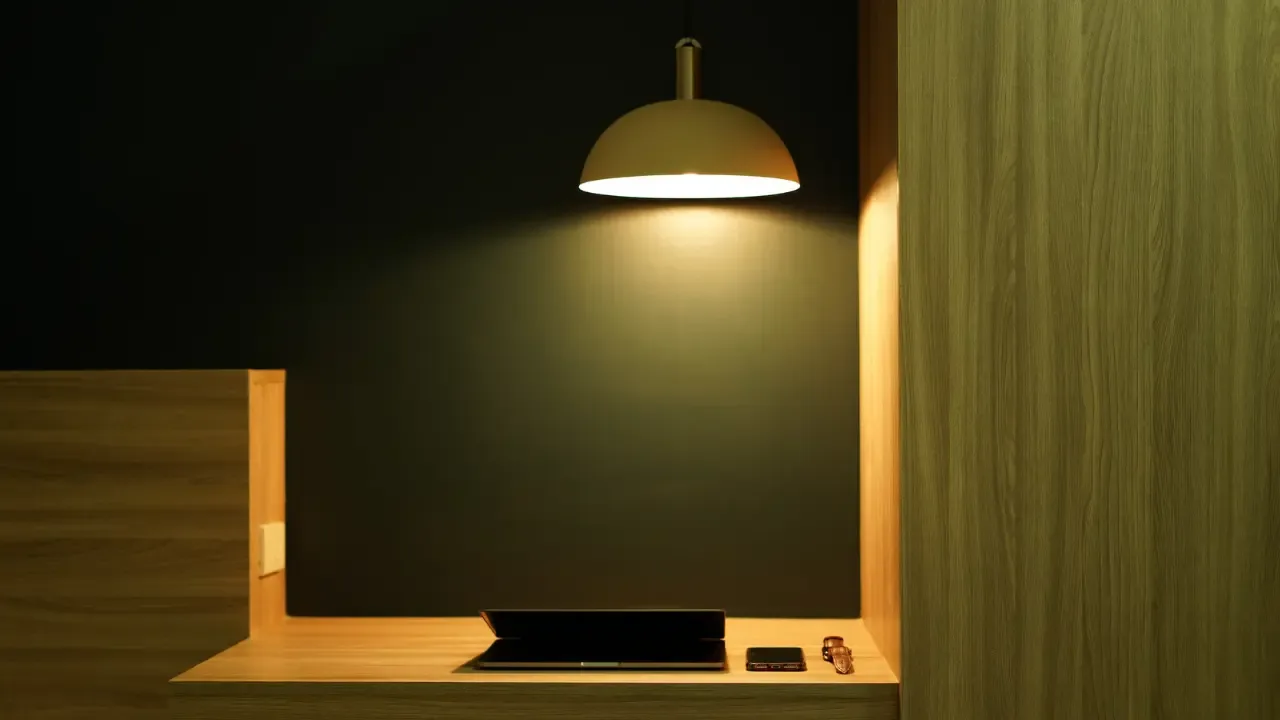
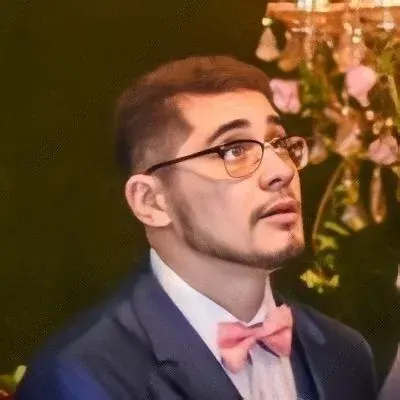
The Magic Behind the Java 'for each' Loop 🪄
Have you ever come across the Java 'for each' loop and wondered how it actually works? 🤔 Well, wonder no more! In this post, we'll demystify this loop and show you how it works under the hood. We'll also address common issues and provide easy solutions to help you become a Java loop master! 💪
The 'for each' Loop Explained 🔄
Let's start with the basics. The 'for each' loop, officially known as the enhanced for loop, is a convenient way in Java to iterate over elements of a collection or an array. It saves you from writing boilerplate code and makes your code more concise. 🚀
In the given context, we have a List<String>
called someList
, which is initialized with the values "monkey", "donkey", and "skeleton key". Now, let's explore how the 'for each' loop processes this collection. 📚
for (String item : someList) {
System.out.println(item);
}
When executed, this loop iterates over each element in someList
and assigns it to the variable item
. The loop body then executes, which in this case, prints the value of item
using System.out.println()
. This process repeats until there are no more elements in the collection. It's as simple as that! 🎉
The Equivalent 'for' Loop 🔢
Now, you might be wondering how to achieve the same result without using the 'for each' syntax. Fear not! We've got you covered. 😎
To create the equivalent 'for' loop, you need to understand that under the hood, the 'for each' loop uses an iterator or indexed access to traverse the collection. Here's how the equivalent 'for' loop would look like:
for (int i = 0; i < someList.size(); i++) {
String item = someList.get(i);
System.out.println(item);
}
In this version, we use a classic 'for' loop to iterate over the collection. We start with an index i
set to zero and compare it against the size of someList
. The loop continues as long as i
is less than the size of the collection. We retrieve the element at index i
using someList.get(i)
and assign it to the variable item
. Finally, we can perform any desired operations using item
. 💡
Common Pitfalls and Easy Solutions 🐛
When working with the 'for each' loop, there are a couple of things to keep in mind to avoid stumbling blocks. Let's have a quick look at some common issues and easy solutions:
1. Concurrent Modification Exception 🚷
If you try to modify the collection while iterating over it using the 'for each' loop, you'll encounter a Concurrent Modification Exception. To prevent this, use an iterator or remove elements using the Iterator
object's remove()
method.
2. Performance Concerns 🐢
While the 'for each' loop is convenient, it may have a slight performance impact compared to traditional 'for' loops, especially when working with huge collections. If performance is critical, consider using a 'for' loop with indexed access instead.
Engage and Become a Loop Master! 💻
Now that you've mastered the magic behind the Java 'for each' loop, it's time to put it into practice! 🚀 Experiment with it, explore different collections and arrays, and get creative with your code!
Let us know in the comments section how the 'for each' loop has made your coding life easier. Share your insights, experiences, and any cool tricks you've discovered along the way. Join the loop master community and keep the discussion going! 😄
Happy looping! 🔄💥