How does autowiring work in Spring?
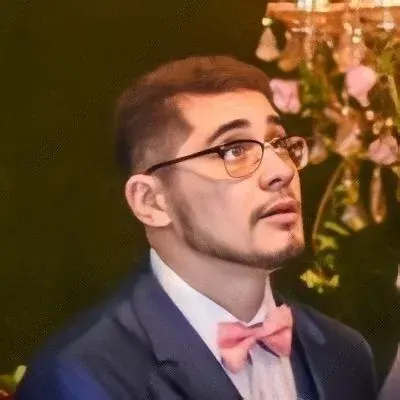
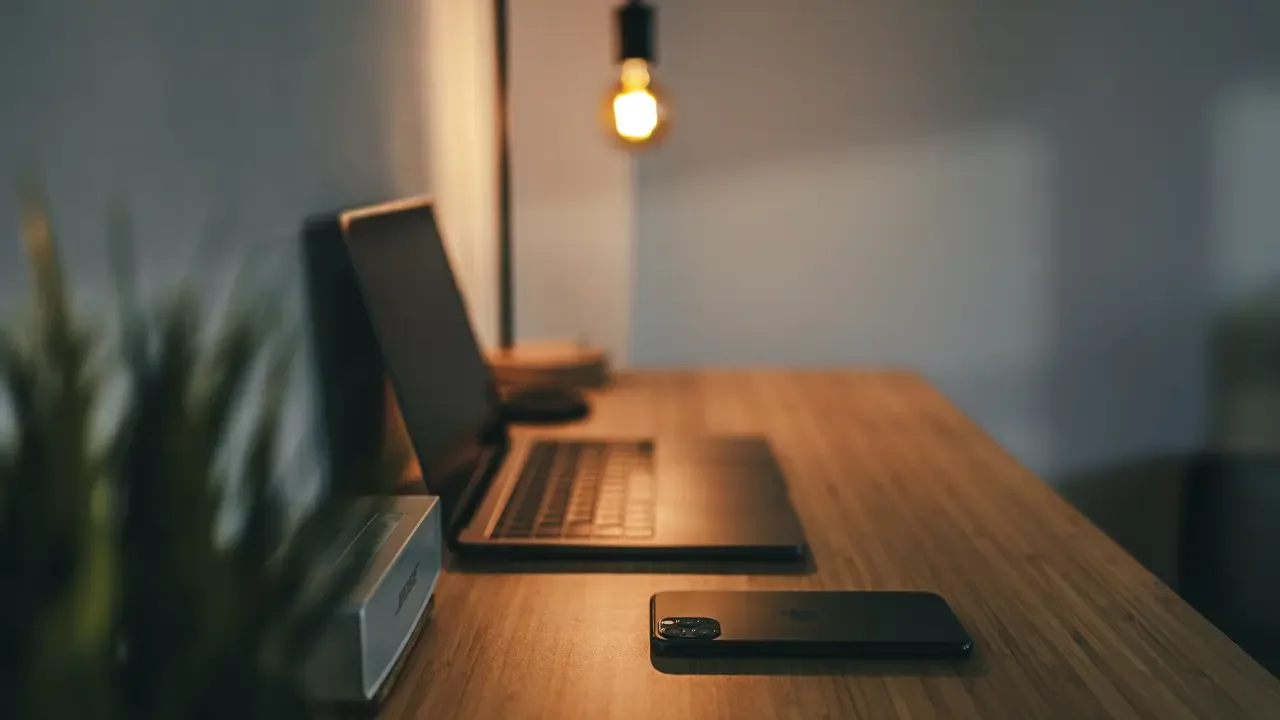
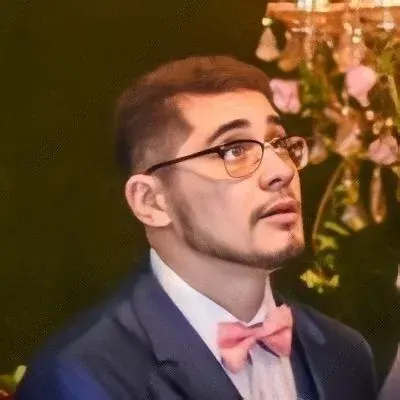
How Does Autowiring Work in Spring? 🌱
Have you ever been confused about how the inversion of control (IoC) works in Spring? Don't worry, you're not alone! Autowiring is a powerful feature in Spring that can simplify your code and make your life easier. In this blog post, we'll delve into the world of autowiring and discuss common issues and easy solutions. So, let's get started!
Understanding Autowiring 🤔
Autowiring is a mechanism in Spring that allows the framework to automatically wire up dependencies for your classes. Instead of manually instantiating and injecting the dependencies, Spring takes care of it for you. This promotes loose coupling, increases code modularity, and makes your application more maintainable.
Wiring Up Your Service Class ⚙️
Let's take an example where you have a service class called UserServiceImpl
that implements the UserService
interface. To autowire this service, you simply need to add the @Autowired
annotation. Here's how it would look:
@Autowired
private UserService userService;
By adding this annotation, Spring will scan your application context, find the implementation of the UserService
, and inject it for you.
Instantiating the Service in Your Controllers 🎮
Now, let's move on to how you would instantiate an instance of the UserService
in your controllers. In the past, you might have done something like this:
UserService userService = new UserServiceImpl();
But with Spring's autowiring, you don't need to do this manual instantiation anymore. Instead, you can simply declare the dependency using the @Autowired
annotation in your controller class. Here's an example:
@Controller
public class UserController {
@Autowired
private UserService userService;
// Your controller methods go here...
}
Spring will take care of injecting the instance of the UserService
for you, allowing you to use it within your controller methods.
Common Issues and Easy Solutions 💡
Issue 1: Multiple Implementations
If you have multiple implementations of the same interface, Spring might not know which one to pick for autowiring. In such cases, you can use the @Qualifier
annotation to specify the exact implementation you want. For example:
@Autowired
@Qualifier("userServiceImpl")
private UserService userService;
Issue 2: Circular Dependencies
Circular dependencies can cause a headache when it comes to autowiring. If you find yourself stuck in a circular dependency, consider using constructor-based dependency injection instead. This approach helps break the circular reference and allows you to resolve the issue. Here's an example:
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
Engage with the Community! 🌐
Now that you have a better understanding of how autowiring works in Spring, why not share your thoughts with the community? Leave a comment below and let us know your experiences, challenges, or any additional tips you have. We'd love to hear from you! 🙌
Remember, autowiring is just one aspect of Spring's magic. Explore more features and unleash the full power of this amazing framework. Happy coding! 😄✨