How do you get current active/default Environment profile programmatically in Spring?
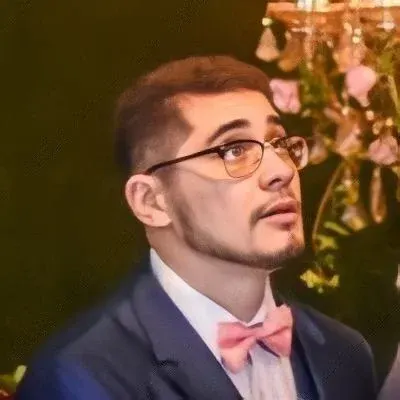
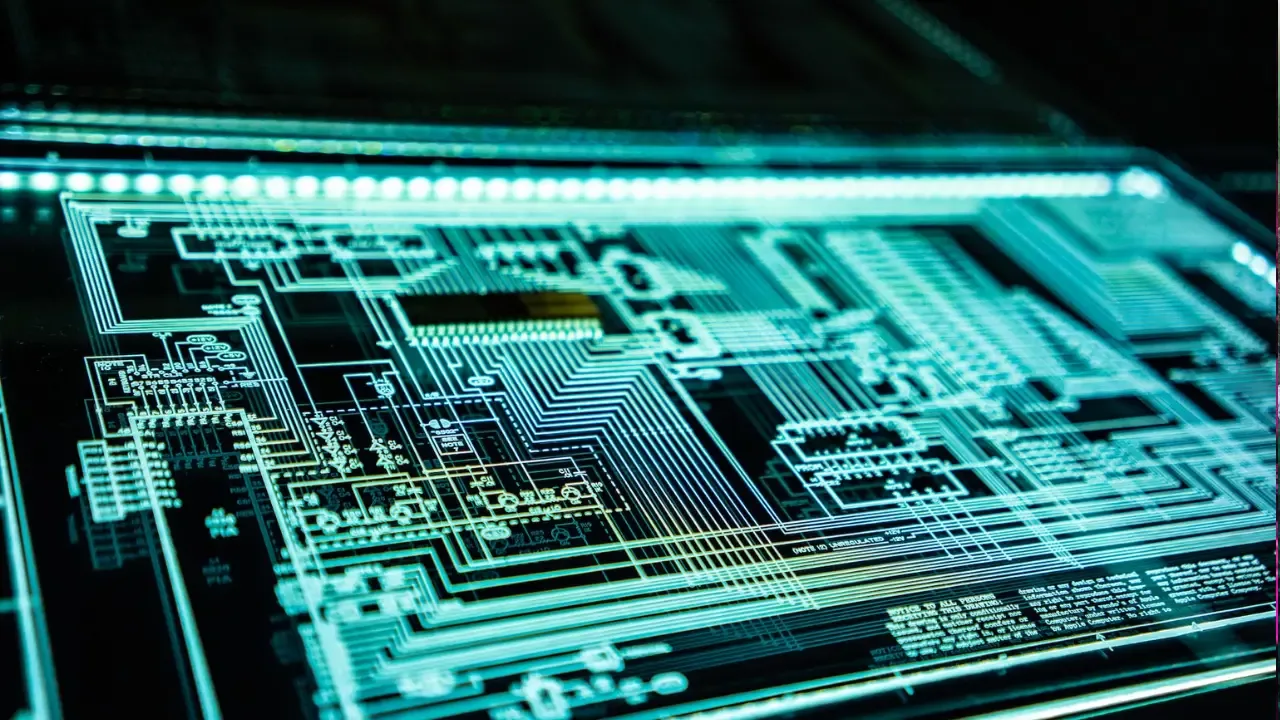
How to Get the Current Active/Default Environment Profile Programmatically in Spring? π±π
Are you finding yourself in a situation where you need to implement different logic based on the current environment profile in your Spring application? π€ Well, fret not! In this blog post, we will walk you through the process of programmatically obtaining the currently active and default profiles in Spring. π
Understanding the Context π
Before diving into the solution, let's first understand the context behind this question. In Spring, profiles allow you to define different sets of configurations that can be activated based on the current environment. This feature comes in handy when you want to have specific configurations for development, testing, production, or any other environment you may have. π¨βπ»
The Challenge π§©
To adapt your application logic based on different profiles, you need to be able to determine which profile is currently active. Additionally, you may also want to know which profile is set as the default.
The Solution π‘
To programmatically obtain both the active and default profiles in Spring, you can leverage the Environment
interface provided by the Spring Framework. The Environment
interface offers a variety of methods to access environment-specific information, including profiles.
Here's how you can retrieve the active profiles:
import org.springframework.core.env.Environment;
@Autowired
Environment environment;
public List<String> getActiveProfiles() {
return Arrays.asList(environment.getActiveProfiles());
}
By calling getActiveProfiles()
on the Environment
object, you will receive an array of active profiles. If multiple profiles are active, they will be returned as separate elements in the array.
To obtain the default profile, you can make use of the getDefaultProfiles()
method:
public List<String> getDefaultProfiles() {
return Arrays.asList(environment.getDefaultProfiles());
}
Much like getActiveProfiles()
, calling getDefaultProfiles()
will return the default profile(s) as an array.
Going the Extra Mile πββοΈπ¨
Now that you have grasped the concept of retrieving active and default profiles, you can level up your Spring game by implementing conditional logic based on these profiles. Act on different profiles by using conditional statements such as if
or switch
. For example:
List<String> activeProfiles = getActiveProfiles();
if (activeProfiles.contains("development")) {
// Execute logic for the development profile
// ...
} else if (activeProfiles.contains("production")) {
// Execute logic for the production profile
// ...
} else {
// Execute default logic
// ...
}
Engage with the Community! ππ€
We hope that this blog post has shed some light on how to programmatically retrieve the current active and default environment profiles in Spring. Feel free to share your thoughts, comments, or experiences with profiles in Spring in the section below. Let's collaborate and help each other navigate through the wonderful world of Spring! πΌπΈ
Remember to follow us on social media and subscribe to our newsletter to stay up-to-date with the latest tech tips and tricks. Happy coding! π»πβ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
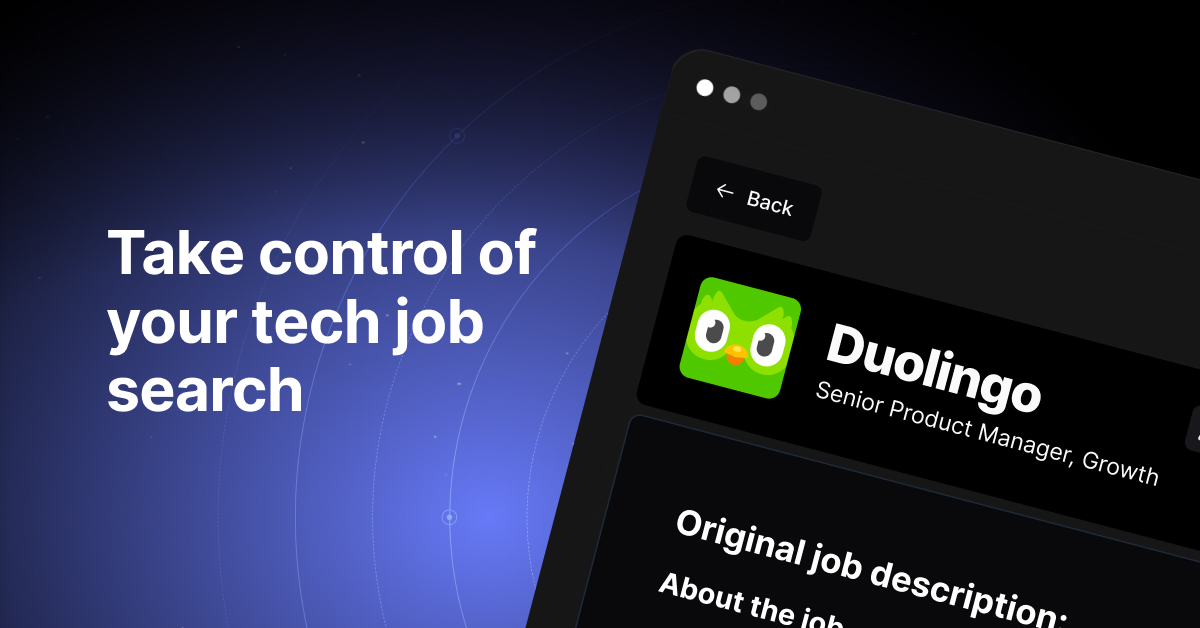