How do you assert that a certain exception is thrown in JUnit tests?
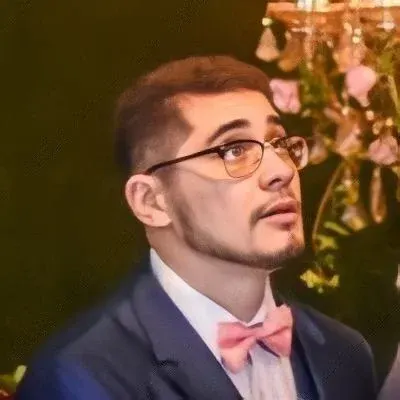

How to Assert That a Certain Exception is Thrown in JUnit Tests ๐งช
Are you tired of writing convoluted code to test if a specific exception is thrown in your JUnit tests? ๐คฏ Well, fret no more! In this blog post, we will show you a more elegant and idiomatic way to tackle this common testing problem. ๐๐ผ
The Old Kludgy Way ๐ฉ
Before we dive into the better solution, let's take a look at the old, cumbersome approach that you might be familiar with. ๐
@Test
public void testFooThrowsIndexOutOfBoundsException() {
boolean thrown = false;
try {
foo.doStuff();
} catch (IndexOutOfBoundsException e) {
thrown = true;
}
assertTrue(thrown);
}
In this code snippet, we wrap the foo.doStuff()
call in a try-catch block and set a boolean flag thrown
to true if the expected exception is caught. Finally, we assert that the thrown
flag is true using assertTrue
. ๐
This approach works, but let's be honest, it feels clunky and unidiomatic. It would be great if there was a simpler and more elegant way to handle this situation. ๐ค
The Elegant JUnit Way ๐
Luckily, JUnit provides a delightful solution to address this issue. You can achieve the same result using, drumroll, please... annotations! ๐
The annotation we're looking for is @Test
and expected
. It allows us to assert that a specific exception is thrown during our test. Let's see how it looks:
@Test(expected = IndexOutOfBoundsException.class)
public void testFooThrowsIndexOutOfBoundsException() {
foo.doStuff();
}
๐ฅ Whoa! It's as simple as that! ๐ฅ
By adding the expected
attribute to the @Test
annotation and specifying the exception class, we tell JUnit that we expect this exception to be thrown during the test. If the exception is thrown, the test passes. If not, the test fails. ๐ฏ
A Quick Word on Exception Superclasses or Subclasses ๐
Sometimes, you may want to test that an exception derived from a specific superclass is thrown. JUnit has got you covered! You can use the @Test
annotation with both the expected
and org.junit.rules.ExpectedException
together. ๐ช๐ผ
For example, to assert that an IOException
or any of its subclasses is thrown, you can do the following:
@Rule
public ExpectedException exceptionRule = ExpectedException.none();
@Test
public void testFooThrowsIOException() {
exceptionRule.expect(IOException.class);
foo.doStuff();
}
Time to Clean Up Your Tests! ๐งน
Now that you know the elegant way of asserting that a certain exception is thrown in JUnit tests, go ahead and refactor your existing tests to make them more readable and maintainable. Your future self will thank you! ๐๐ปโโ๏ธ
Share Your Thoughts and Experiences! ๐ฌ
Have you encountered any struggles when testing exceptions in JUnit? What other testing challenges would you like us to address in future blog posts? Let's start a lively discussion in the comments below! Let's share knowledge and level up our testing game together! ๐โจ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
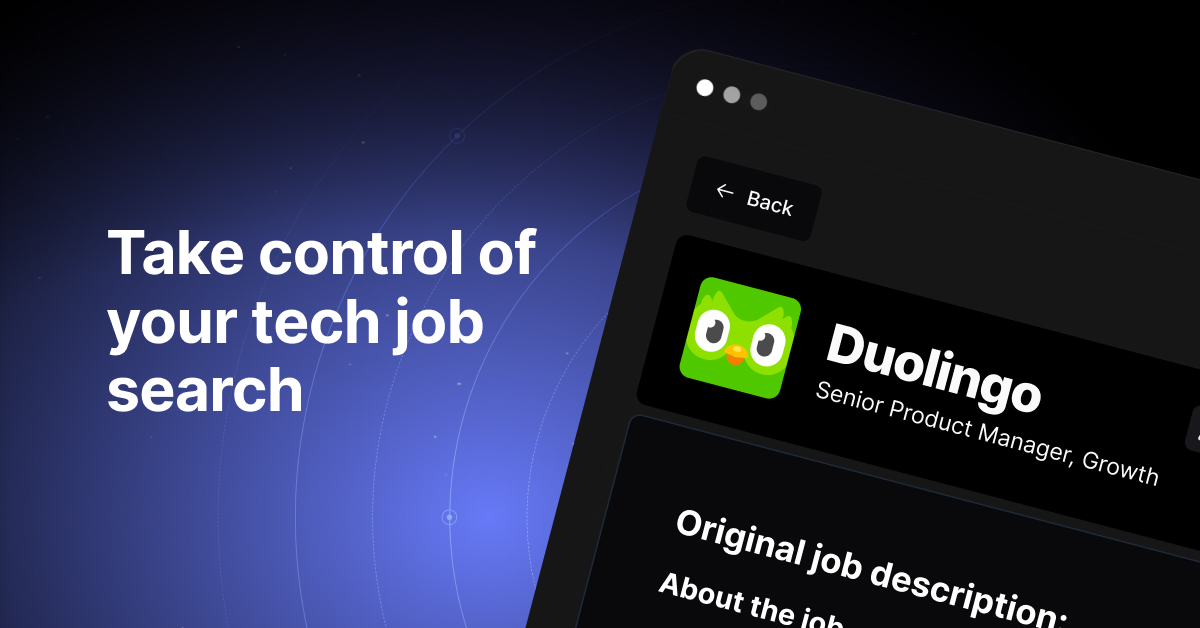