How do I time a method"s execution in Java?
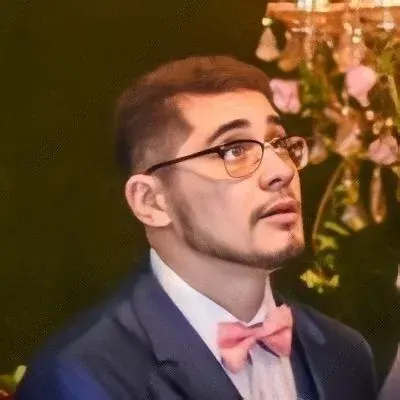
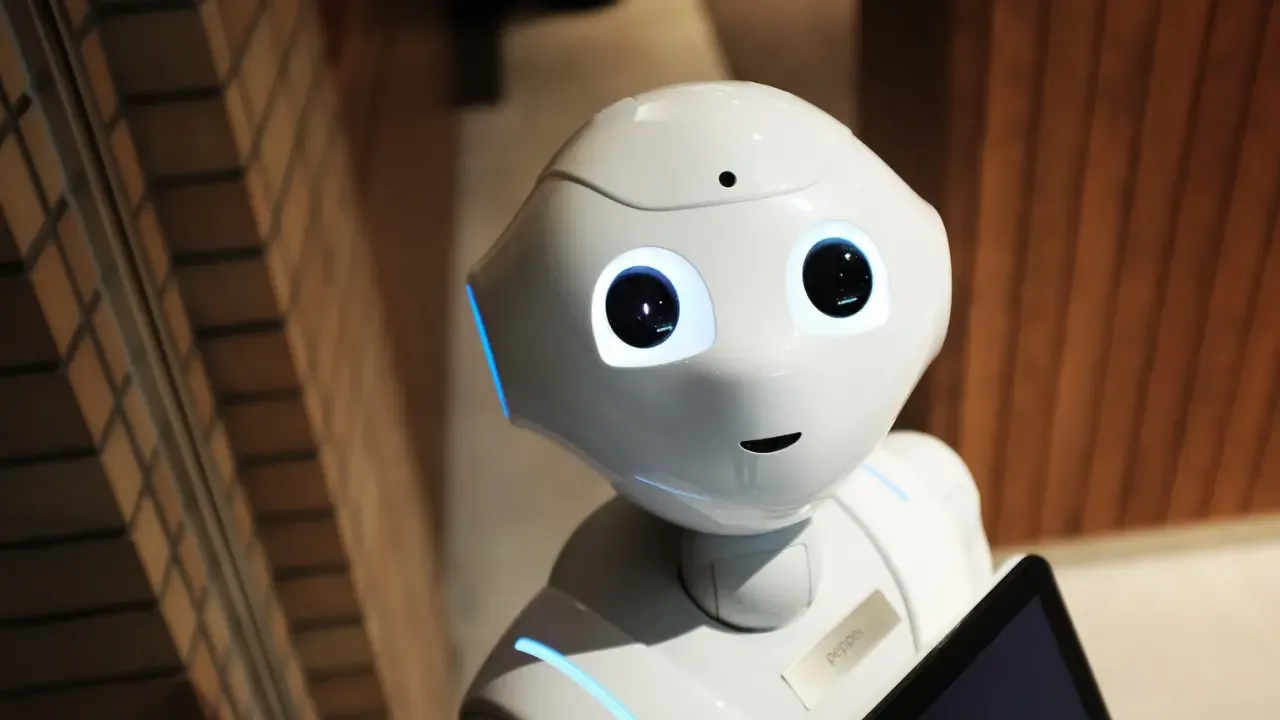
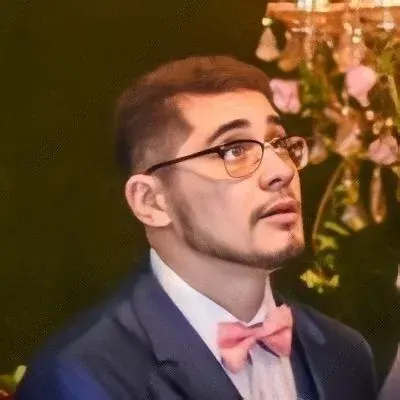
How to Time a Method's Execution in Java
:clock3: Have you ever wondered how long it takes for a method to execute in your Java program? Whether you are optimizing your code or just curious about its performance, being able to time a method's execution is a valuable skill to have. :hourglass_flowing_sand:
:question: So, how do you actually time a method's execution in Java? Let's dive into it and address some common issues along the way. :computer:
Common Issues
:exclamation: One common issue is that most searches on Google return results for timers that schedule threads and tasks, which is not what we want. Timing a method's execution is a different concept altogether. :thinking:
Solution
:zap: The solution to time a method's execution in Java is simple yet effective. We can achieve this by using the System.currentTimeMillis()
method. Let's walk through the steps:
:watch: First, record the current time using
System.currentTimeMillis()
before the method begins executing. This will give us the starting time for our timer.
long startTime = System.currentTimeMillis();
:stopwatch: Next, execute the method that you want to time.
// Method to be timed
yourMethod();
:watch: Finally, calculate the elapsed time by subtracting the starting time from the current time after the method has finished executing.
long endTime = System.currentTimeMillis();
long elapsedTime = endTime - startTime;
:hourglass: Voila! You now have the elapsed time in milliseconds. You can use this information for optimization purposes or to measure the performance of your code.
Example
Let's put this solution into action with a simple example. Suppose we have a method called calculateSum()
that finds the sum of numbers from 1 to a specified value n
. We want to time its execution.
public class Main {
public static void main(String[] args) {
int n = 100000000;
long startTime = System.currentTimeMillis();
calculateSum(n);
long endTime = System.currentTimeMillis();
long elapsedTime = endTime - startTime;
System.out.println("Elapsed Time: " + elapsedTime + " milliseconds");
}
public static int calculateSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
}
When we run this code, we will get the output:
Elapsed Time: 40 milliseconds
This means that it took approximately 40 milliseconds for the calculateSum()
method to execute with n
as 100,000,000.
Engage and Share!
:loudspeaker: Now that you have learned how to time a method's execution in Java, why not try it out in your own code? Experiment with different methods and see how their execution times vary.
:star: If you found this blog post helpful, make sure to share it with your fellow Java enthusiasts! Let's spread the knowledge and make everyone's code more efficient and performant. :rocket:
Leave a comment below and let me know how you have used method timing in your Java projects. I'd love to hear your experiences and any additional tips or tricks you have! :bulb: