How do I split a string in Java?
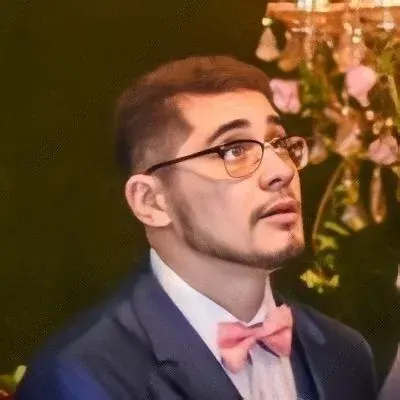
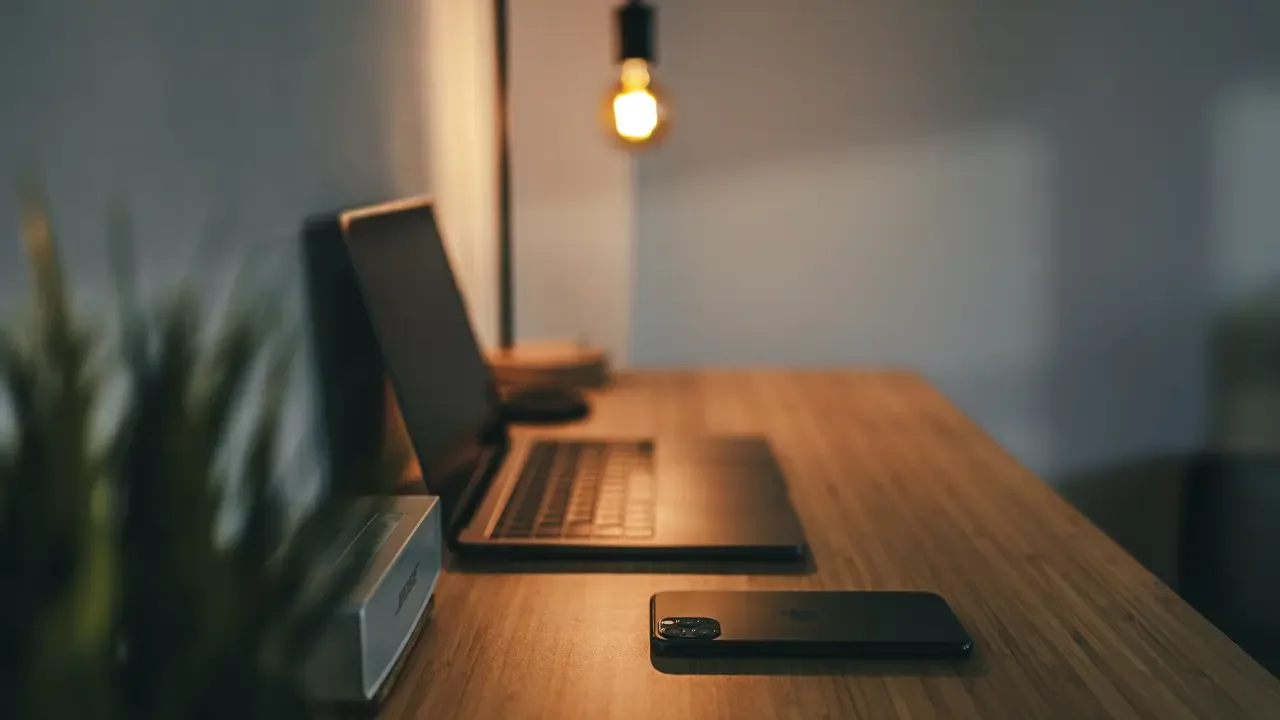
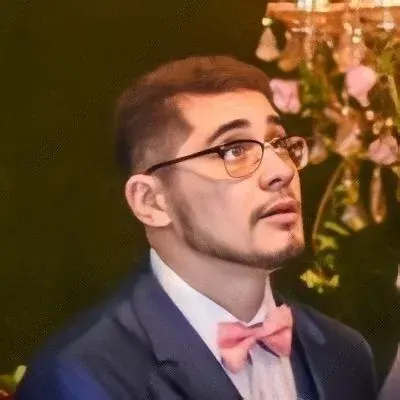
Splitting Strings in Java: A Simple Guide 🧩
Are you struggling with splitting a string in Java? Don't worry, we've got you covered! In this guide, we'll walk you through step by step on how to split a string and handle common issues. Let's dive in! 💪
Understanding the Problem 🤔
Before we start, let's take a closer look at the problem statement. You want to split the string "004-034556" into two strings using the delimiter "-". The expected outcome is:
part1 = "004";
part2 = "034556";
Additionally, you want to check if the string contains the delimiter "-". Now that we have a clear understanding, let's explore the solutions! 💡
Solution 1: Using the split()
Method ⚙️
In Java, the split()
method is the easiest way to split a string based on a delimiter. Here's how you can do it:
String inputString = "004-034556";
String[] parts = inputString.split("-");
String part1 = parts[0];
String part2 = parts[1];
In this solution, the split("-")
method returns an array of strings, splitting the original string wherever it finds the delimiter "-". By accessing parts[0]
and parts[1]
, we can assign the split substrings to part1
and part2
, respectively. 🎉
Solution 2: Using the indexOf()
Method 🧮
If you want to check if the string contains the delimiter before splitting it, you can use the indexOf()
method. Here's an example:
String inputString = "004-034556";
int delimiterIndex = inputString.indexOf("-");
if (delimiterIndex >= 0) {
String part1 = inputString.substring(0, delimiterIndex);
String part2 = inputString.substring(delimiterIndex + 1);
// Your logic here...
}
In this solution, indexOf("-")
returns the index of the first occurrence of "-". If the delimiter exists (i.e., delimiterIndex >= 0
), you can extract the substrings using substring()
. The first substring starts from index 0 to delimiterIndex
, and the second substring starts from delimiterIndex + 1
till the end. 🎈
Handling Exceptions ❗
Keep in mind that both solutions assume the delimiter exists in the string. If the delimiter is not found, the split()
method will return an array with the original string, and the indexOf()
method will return -1. Be sure to handle these cases to avoid unexpected behavior in your code. 💥
Now It's Your Turn! 🚀
Now that you've learned two different ways to split a string in Java, it's time to put your skills into practice! Test these solutions with different inputs and explore more advanced use cases. Share your experience with us in the comments below! We'd love to hear your thoughts and answer your questions. Let's keep learning together! 💡🤓💬
Remember, practice makes perfect! Keep coding, keep exploring, and keep improving. Happy coding! 😄💻✨