How do I read / convert an InputStream into a String in Java?
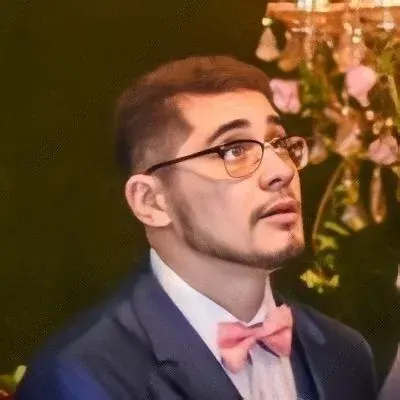
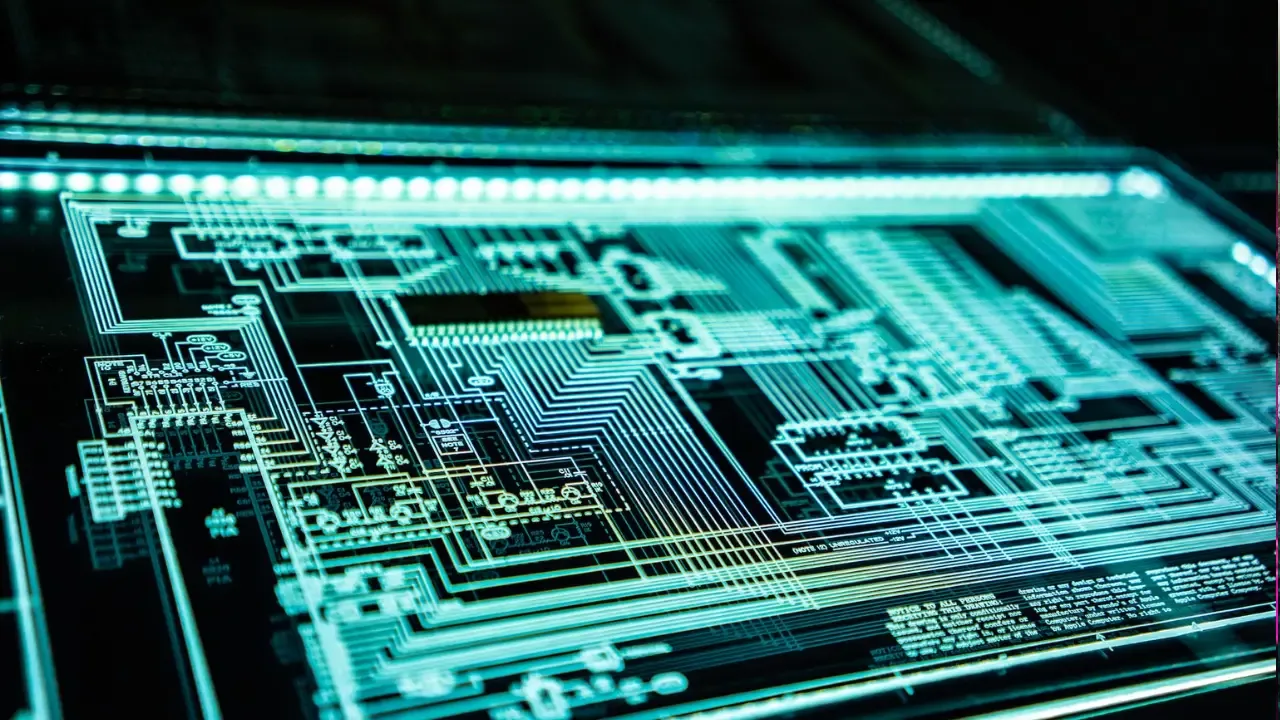
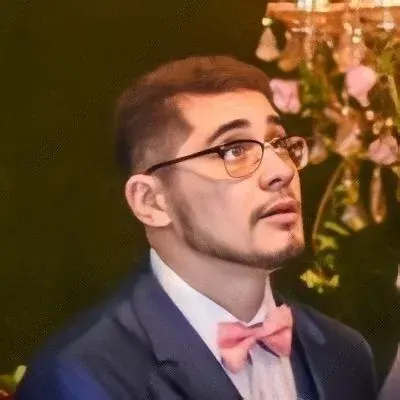
How to Read/Convert an InputStream into a String in Java? 📚
<p>Have you ever found yourself in a situation where you needed to convert an <code>InputStream</code> into a <code>String</code> in Java? Whether you want to process text data or write it to a log file, understanding how to convert an <code>InputStream</code> to a <code>String</code> is a valuable skill for any Java developer. In this blog post, we will explore some common issues, provide easy solutions, and empower you to tackle this problem like a pro. Let's dive in! 💻</p>
The Problem at Hand 🤔
<p>Let's say you have an <code>InputStream</code> that contains text data, and you want to convert it into a <code>String</code>. Here's an example method signature:</p>
public String convertStreamToString(InputStream is) {
// ??? 🤷♂️
}
<p>You are left wondering, what is the easiest and most efficient way to convert this <code>InputStream</code> into a <code>String</code>? Well, fret not! We have got your back! 😎 Let's explore some solutions below.</p>
Solution 1: Using BufferedReader and StringBuilder 📝
<p>An efficient way to convert an <code>InputStream</code> into a <code>String</code> is by using <code>BufferedReader</code> and <code>StringBuilder</code>. Here's how:</p>
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public String convertStreamToString(InputStream is) {
StringBuilder sb = new StringBuilder();
try (BufferedReader br = new BufferedReader(new InputStreamReader(is))) {
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
} catch (IOException e) {
e.printStackTrace();
}
return sb.toString();
}
<p>By using <code>BufferedReader</code> and <code>StringBuilder</code>, we read the <code>InputStream</code> line by line and append each line to the <code>StringBuilder</code> object. Finally, we return the string representation of this <code>StringBuilder</code>. 🚀</p>
Solution 2: Using Apache Commons IO 📦
<p>If you prefer using external libraries, Apache Commons IO provides a handy utility class called <code>IOUtils</code> that simplifies the task of converting an <code>InputStream</code> into a <code>String</code>. Here's how you can do it:</p>
import org.apache.commons.io.IOUtils;
public String convertStreamToString(InputStream is) {
try {
return IOUtils.toString(is, StandardCharsets.UTF_8);
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
<p>By leveraging <code>IOUtils.toString()</code>, we can easily convert the <code>InputStream</code> into a <code>String</code> using the specified character encoding (in this case, UTF-8). Apache Commons IO takes care of handling the necessary conversions and error handling. 🌟</p>
The Choice is Yours! ✨
<p>With these two solutions at your disposal, you can confidently convert an <code>InputStream</code> into a <code>String</code> in Java. Choose the approach that best suits your needs and coding style. Keep in mind that the first solution is using standard Java libraries, while the second one requires an external dependency. 🔀</p>
<p>We hope this blog post helps you overcome this common challenge in Java development. If you have any questions, suggestions, or alternative approaches, feel free to share them in the comments section below. Happy coding! 😄👩💻👨💻</p>