How do I join two lists in Java?
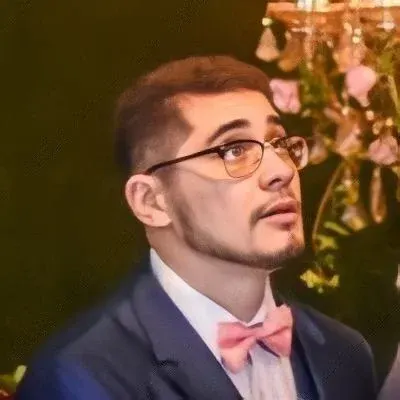
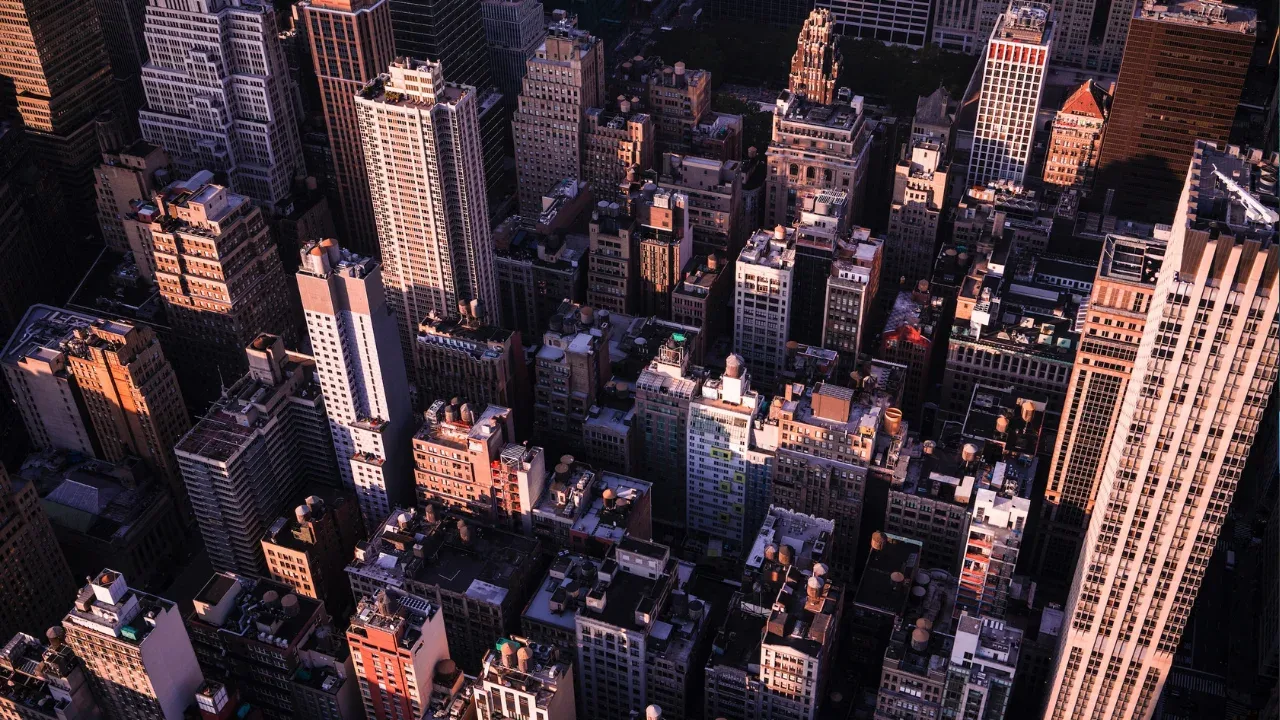
😎 Joining Two Lists in Java: The Easy Way 😎
Are you tired of going through several lines of code just to join two lists in Java? Look no further, because I've got a simple solution for you! 🚀
The Problem 😩
You may have found yourself in a situation where you need to merge two lists in Java. Perhaps you want to combine two lists of names, or maybe you need to concatenate two lists of integers. Whatever the case may be, you want an effortless way to achieve this without modifying the original lists or relying on external libraries. 💪
The Solution 🙌
Luckily, Java provides a straightforward solution using the addAll()
method. 💡 Instead of creating a new list and adding each element from both lists individually, you can simplify the process into a single line of code. Check it out:
List<String> newList = new ArrayList<>();
newList.addAll(listOne);
newList.addAll(listTwo);
That's it! 🎉 By calling the addAll()
method on your new list, you can merge both listOne
and listTwo
seamlessly. No complex logic, no loops, just plain and simple code. It's like combining your favorite toppings on a pizza effortlessly! 🍕
But Wait! There's More! 🌟
If you're looking for an even shorter solution, you're in luck! Java 8 introduced the Stream API, which allows for more concise and expressive code. Using a one-liner, you can join your lists like a pro:
List<String> newList = Stream.concat(listOne.stream(), listTwo.stream())
.collect(Collectors.toList());
Now, that's what I call coding efficiency! 💯
JDK 1.3 Version ⏳
But what if you're stuck using an older version of the JDK? Don't worry, I've got you covered! While the previous solutions won't work in JDK 1.3, you can still achieve the desired outcome by using a classic loop:
List<String> newList = new ArrayList<>();
for (String item : listOne) {
newList.add(item);
}
for (String item : listTwo) {
newList.add(item);
}
Although it requires a few extra lines of code, it's a small price to pay to make it work on older JDK versions. ⏰
Conclusion 🎬
Joining two lists in Java doesn't have to be a complicated and time-consuming task. By utilizing the addAll()
method or the Stream API, you can effortlessly combine your lists and achieve the desired outcome. Whether you're using the latest JDK version or stuck with an older one, we've provided solutions for you!
So go ahead, give it a try, and simplify your list-merging adventures! 🌈
Remember, simplicity is the key! 😊
Did you find this blog post helpful? Have any other questions or tricks up your sleeve? Let us know in the comments below! Let's join forces and make Java coding even more awesome! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
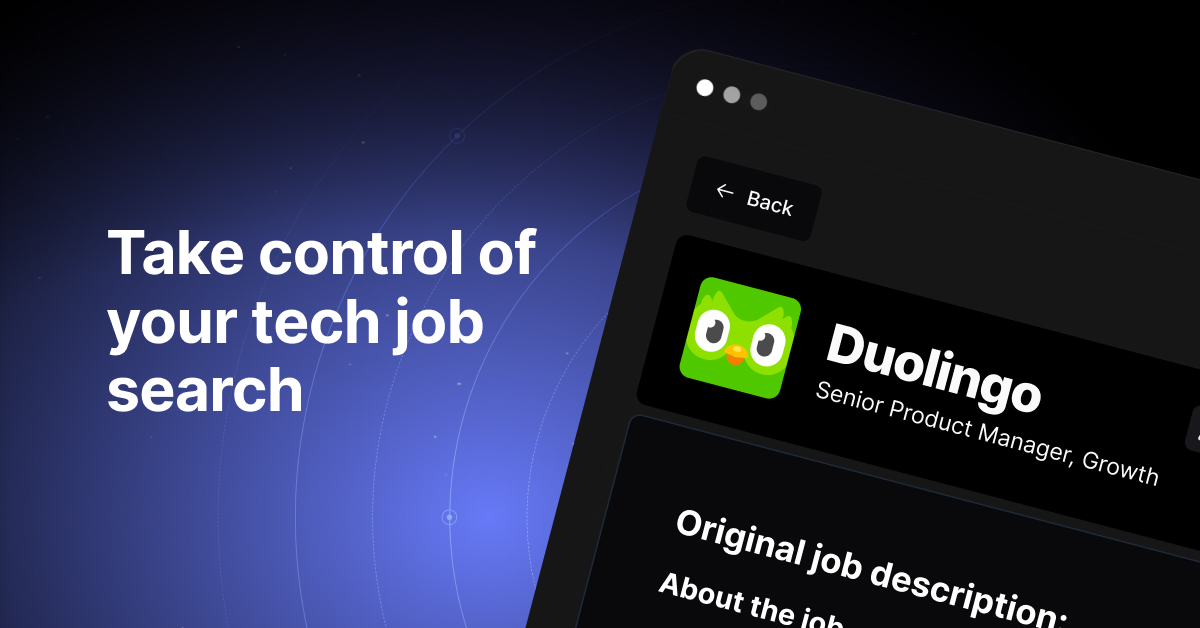