How do I get a class instance of generic type T?
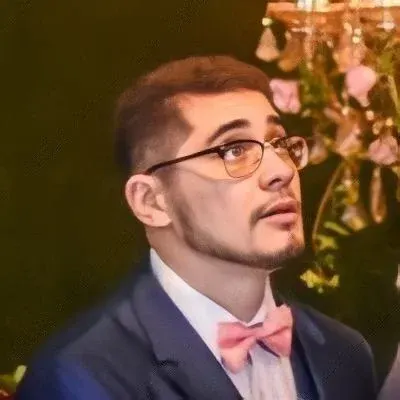
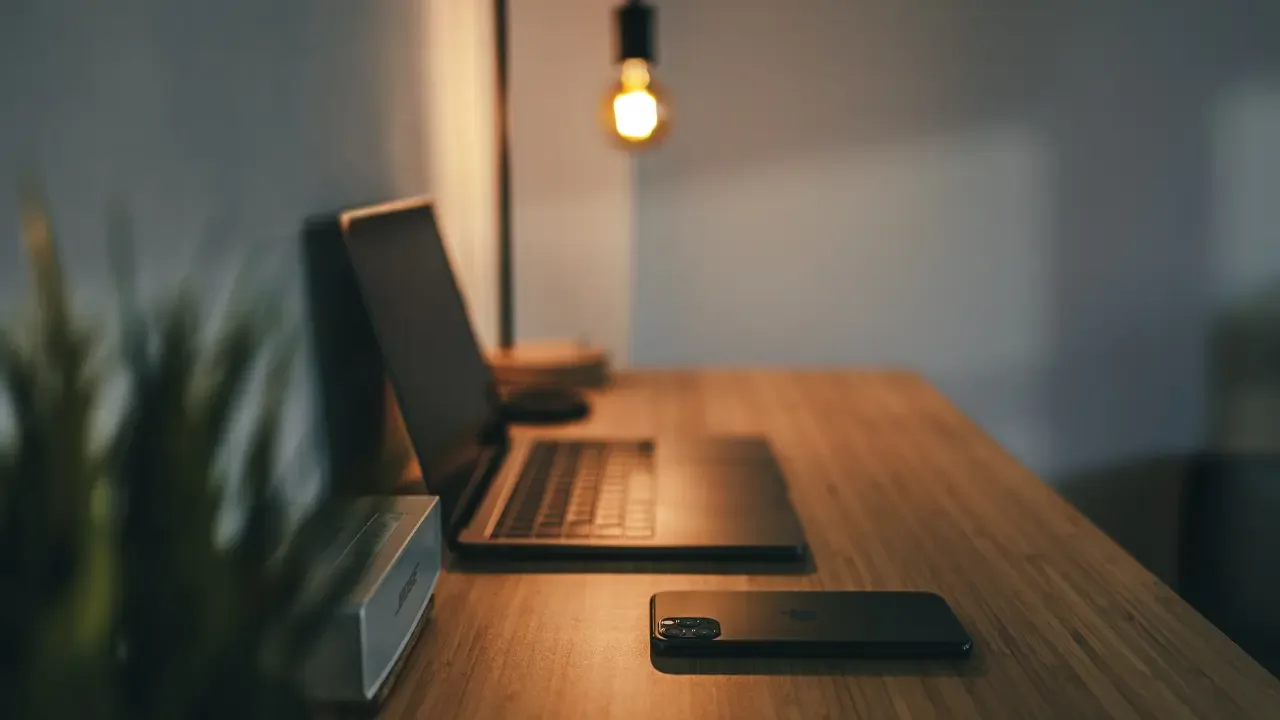
๐ Title: How to Get a Class Instance of Generic Type T: Demystifying Common Issues and Providing Easy Solutions! ๐จโ๐ป๐ค
Hello there, tech-savvy folks! Are you struggling with getting a class instance of a generic type T? Fret not, for today we shall unravel this mystery and equip you with the knowledge to conquer this common issue! ๐ฉ๐
So, picture this: you have a fancy generics class called Foo<T>
. Within one of the methods of Foo
, you desire to obtain the class instance of type T
, but alas, invoking T.class
simply won't do the trick. ๐ข
But fear not, for there's a preferred way to bypass this limitation, avoiding the dreaded T.class
obstacle course! Here's what you need to do: ๐
Option 1: Passing Class Parameter ๐๐
One straightforward approach is to tweak your Foo
constructor to allow the type T
class to be passed as a parameter. This way, you'll have direct access to the class instance whenever needed. Let's see it in action:
class Foo<T> {
private final Class<T> clazz;
public Foo(Class<T> clazz) {
this.clazz = clazz;
}
public void someMethod() {
// Access the class instance of T
if (clazz != null) {
// Do something amazing with clazz!
}
}
}
Now, when you create an instance of Foo
, you can simply pass the class type as a parameter:
Foo<String> foo = new Foo<>(String.class);
foo.someMethod();
See, it's as simple as that! You now have a ready-to-use clazz
which opens doors to limitless possibilities! ๐ช๐
Option 2: Extracting Type Information from a Subclass ๐งช๐ฌ
If altering the constructor of Foo
is not suitable for your specific scenario, fear not! Another option involves creating a subclass that extends Foo
and provides the necessary type information. Here's how it can be done:
class FooChild<T> extends Foo<T> {
public FooChild() {
super(extractClass());
}
private static <T> Class<T> extractClass() {
// Implement your own logic to extract the class instance of T
// Return the desired Class<T>
}
}
In this approach, the FooChild
subclass takes charge of extracting the class instance of T
. By implementing the extractClass
method, you can unleash your ingenuity to accomplish this.
FooChild<String> fooChild = new FooChild<>();
fooChild.someMethod();
Voila! With a sprinkle of inheritance magic, you've obtained the class instance of T
without breaking a sweat! ๐ช๐ก
Enjoy the Power of T! ๐ฅ๐
Now that you're armed with these foolproof techniques, let your imagination run wild! You can use the class instance of type T
to achieve remarkable feats: creating instances, invoking methods, or even performing astonishing reflections. The possibilities are endless! ๐๐
So go forth, fellow developers, and conquer your generic type challenges with confidence! If you found this guide helpful, share it with your squad of tech enthusiasts. Remember, knowledge is meant to be shared! ๐ฃ๐ค
If you have any other tech conundrums or want to share your success story, drop a comment below. Let's keep the tech community buzzing with excitement and engagement! โจ๏ธ๐ฌโจ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
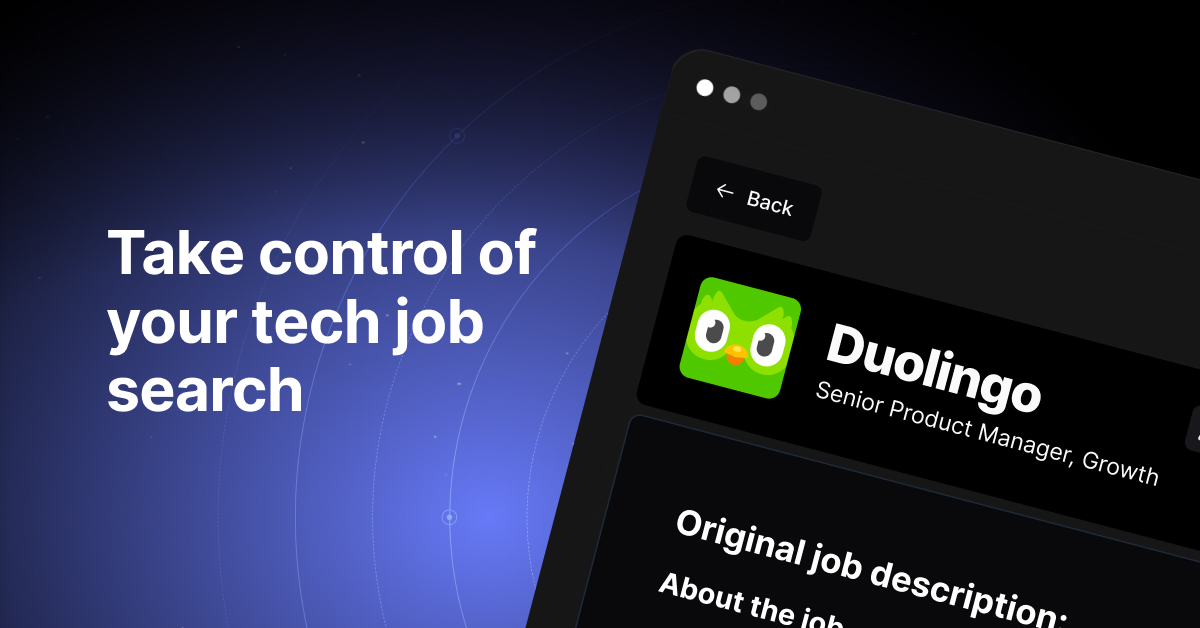