How do I generate random integers within a specific range in Java?
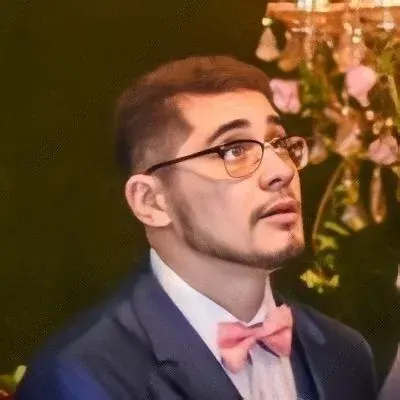
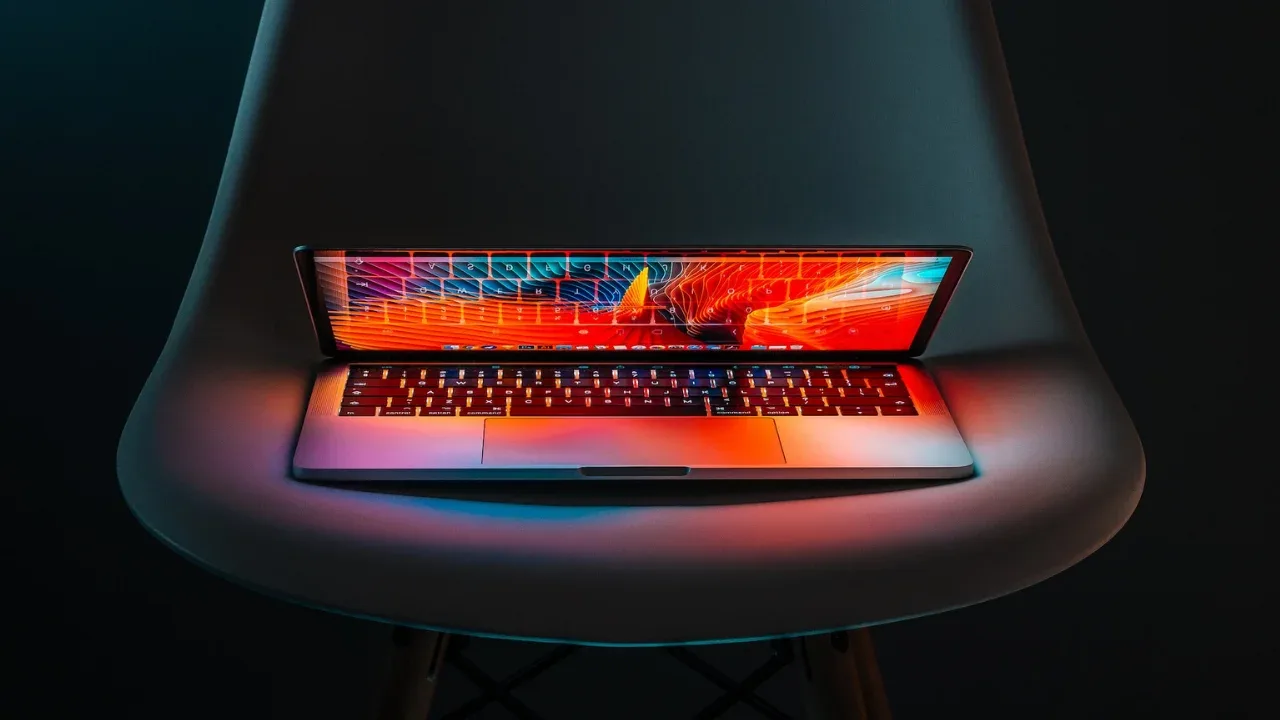
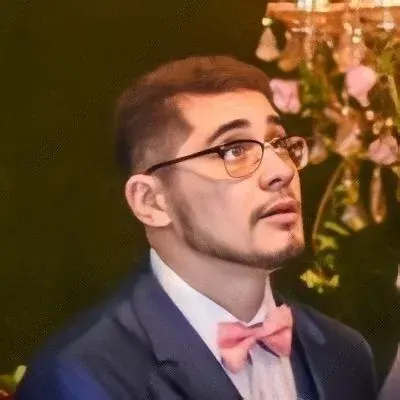
Generating Random Integers within a Specific Range in Java: The Right Way 👌
Do you need to generate random integers within a specific range in your Java program? Look no further, because we've got you covered! 😎 In this blog post, we'll explore the common issues and bugs associated with generating random integers, and provide you with easy-peasy solutions. So, let's dive right in!
The Problem: Integer Overflow and Underflow 🔄
Many Java developers face the issue of integer overflow or underflow when trying to generate random numbers within a specific range. These bugs can lead to unexpected results and may break the functionality of your program. 😱
Example #1: The Math.random() Bug 🐞
randomNum = minimum + (int)(Math.random() * maximum);
// Bug: `randomNum` can be bigger than `maximum`.
In the above code snippet, the intention is to generate a random number between minimum
and maximum
, inclusive. However, this method is flawed because it relies on the Math.random()
function, which returns a double value between 0.0 inclusive and 1.0 exclusive. Therefore, multiplying it by maximum
and casting it to an int
can result in a number greater than maximum
. This is the notorious integer overflow bug. 🙅♂️
Example #2: The nextInt() Bug 👾
Random rn = new Random();
int n = maximum - minimum + 1;
int i = rn.nextInt() % n;
randomNum = minimum + i;
// Bug: `randomNum` can be smaller than `minimum`.
In this code snippet, we use the nextInt()
method from the Random
class to generate random numbers. We calculate the range by subtracting minimum
from maximum
and adding 1
to include both bounds. However, using the remainder operator (%
) on the result of nextInt()
can lead to negative values if the random number generated is negative. This is the infamous integer underflow bug. We then add minimum
to the result, but this can still give us a number smaller than minimum
. Not cool, right? ❌
The Solution: Bound-Safe Random Number Generation 💡
To generate random integers within a specific range in Java, we need to ensure our code is free from any bugs related to integer overflow or underflow. Here's the right way to do it:
Approach #1: Using the nextInt() Method 🎯
Random rn = new Random();
int range = maximum - minimum + 1;
int randomNum = rn.nextInt(range) + minimum;
By using the nextInt(int bound)
method from the Random
class, we specify the bound as range
, which is the desired range of random numbers. This ensures that the generated random number will be between 0
(inclusive) and range
(exclusive). We then add the minimum
value to the result to shift the range to our desired bounds. Voila! No more pesky bugs! 🙌
Approach #2: Using the ThreadLocalRandom Class 🎰
Starting from Java 7, you can also use the ThreadLocalRandom
class to generate random numbers within a specific range without the need to create an instance of Random
.
int randomNum = ThreadLocalRandom.current().nextInt(minimum, maximum + 1);
The current()
method returns the ThreadLocalRandom
instance tied to the current thread, and the nextInt(int origin, int bound)
method generates a random number between origin
(inclusive) and bound
(exclusive). By adding 1
to maximum
, we include both bounds in our range. Amazing, right? 😍
Take the Leap: Generate Random Integers the Right Way! 🚀
Now that you know the correct approach to generate random integers within a specific range in Java, you can apply this knowledge to your projects and wave goodbye to those annoying bugs. Your code will be robust, efficient, and bug-free! 💪
But don't just stop here! There's so much more to discover and learn in the world of Java programming. Keep exploring, experimenting, and pushing the boundaries of your knowledge. And remember, sharing is caring! If you found this post helpful, spread the word and encourage your fellow developers to write bug-free code when generating random integers. Together, we can make the Java community even better! 😉
Happy coding! ✨