How do I efficiently iterate over each entry in a Java Map?
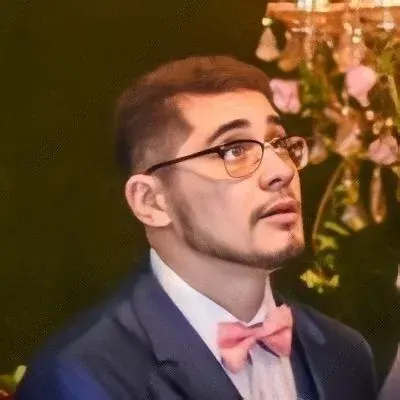
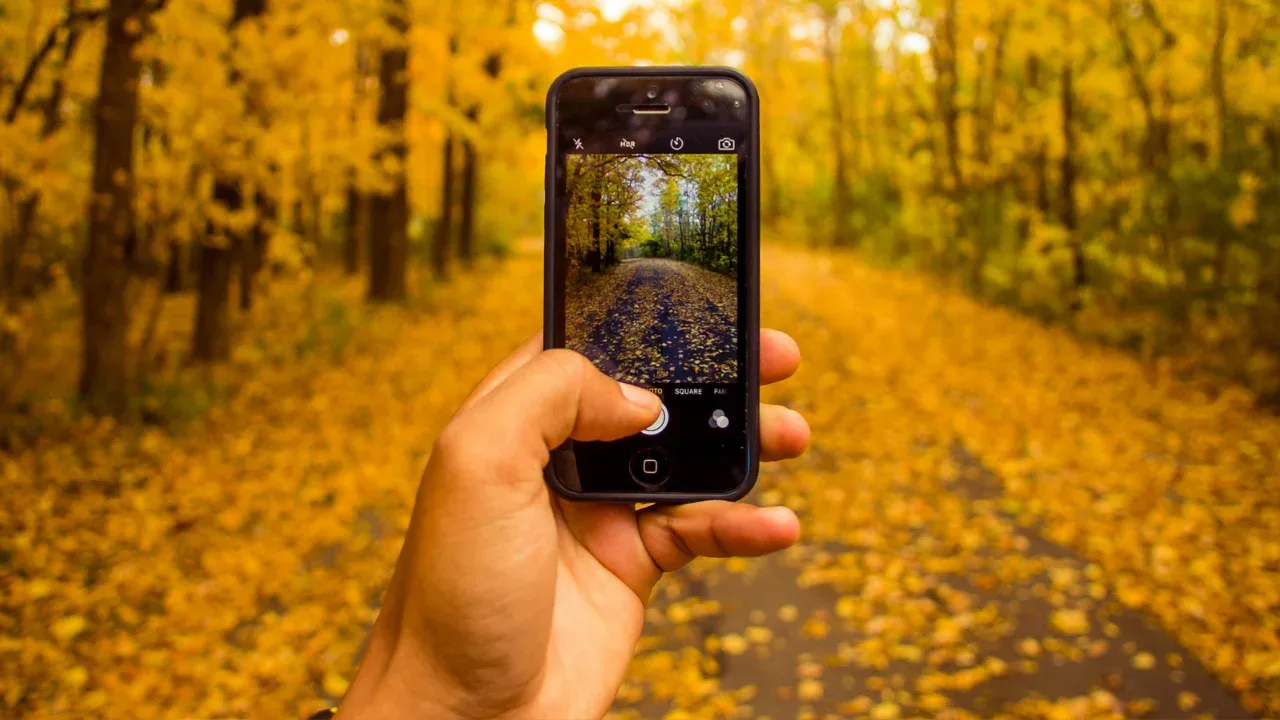
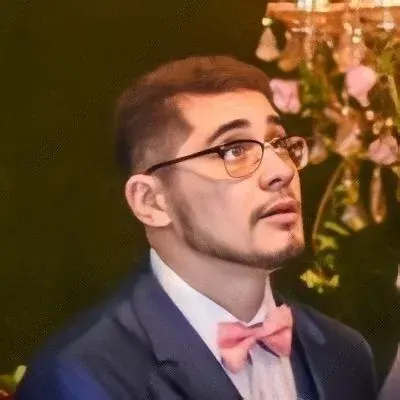
šš„ Hey there, tech enthusiasts! Today we're going to tackle a question that often pops up when working with Java Maps: how to efficiently iterate over each entry in a Java Map? š§
š” First things first, let's address the concern about the ordering of elements in a Map. It's important to note that the ordering of elements in a Map does depend on the specific implementation you're using. There are multiple implementations of the Map interface in Java, such as HashMap, TreeMap, and LinkedHashMap, each with its own characteristics.
š Now, let's dive into the most efficient way to iterate over every pair contained within a Java Map. Drumroll, please! š„
Using the keySet() method: One straightforward approach is to use the
keySet()
method, which returns a Set of all the keys in the Map. You can then iterate over this Set and access the corresponding values from the Map.Map<String, Integer> myMap = new HashMap<>(); // Populate the Map for (String key : myMap.keySet()) { Integer value = myMap.get(key); // Do something with the key-value pair }
This method is efficient as it avoids iterating over the values directly and simplifies accessing both keys and values.
Using the entrySet() method: Another efficient approach is to use the
entrySet()
method, which returns a Set of Map.Entry objects representing each key-value pair in the Map. This method allows you to directly access both the key and value of each entry.Map<String, Integer> myMap = new HashMap<>(); // Populate the Map for (Map.Entry<String, Integer> entry : myMap.entrySet()) { String key = entry.getKey(); Integer value = entry.getValue(); // Do something with the key-value pair }
Using the
entrySet()
method eliminates the need to callget()
on each iteration, making it more efficient when dealing with large Maps.Using Java 8 Streams: If you're using Java 8 or later, you can take advantage of Streams to iterate over a Map easily and elegantly.
Map<String, Integer> myMap = new HashMap<>(); // Populate the Map myMap.forEach((key, value) -> { // Do something with the key-value pair });
Leveraging lambdas and the
forEach()
method, this concise approach offers a functional programming style to iterate over the Map.
Now that you've learned some efficient ways to iterate over a Java Map, it's time to apply this knowledge in your projects and unleash your coding powers! šŖš»
š£ We love hearing from our readers! If you have any other tech questions, need further clarification, or have a brilliant solution to share, leave a comment below and let's start a discussion! Let's keep the tech community thriving with knowledge sharing! š
Happy coding! šš