How do I discover memory usage of my application in Android?
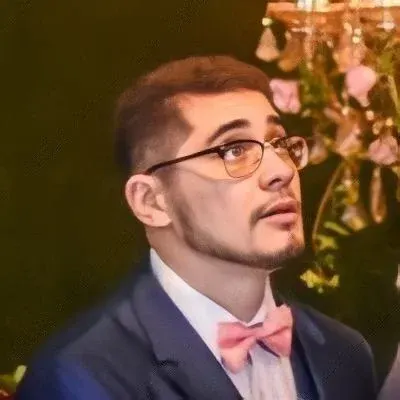
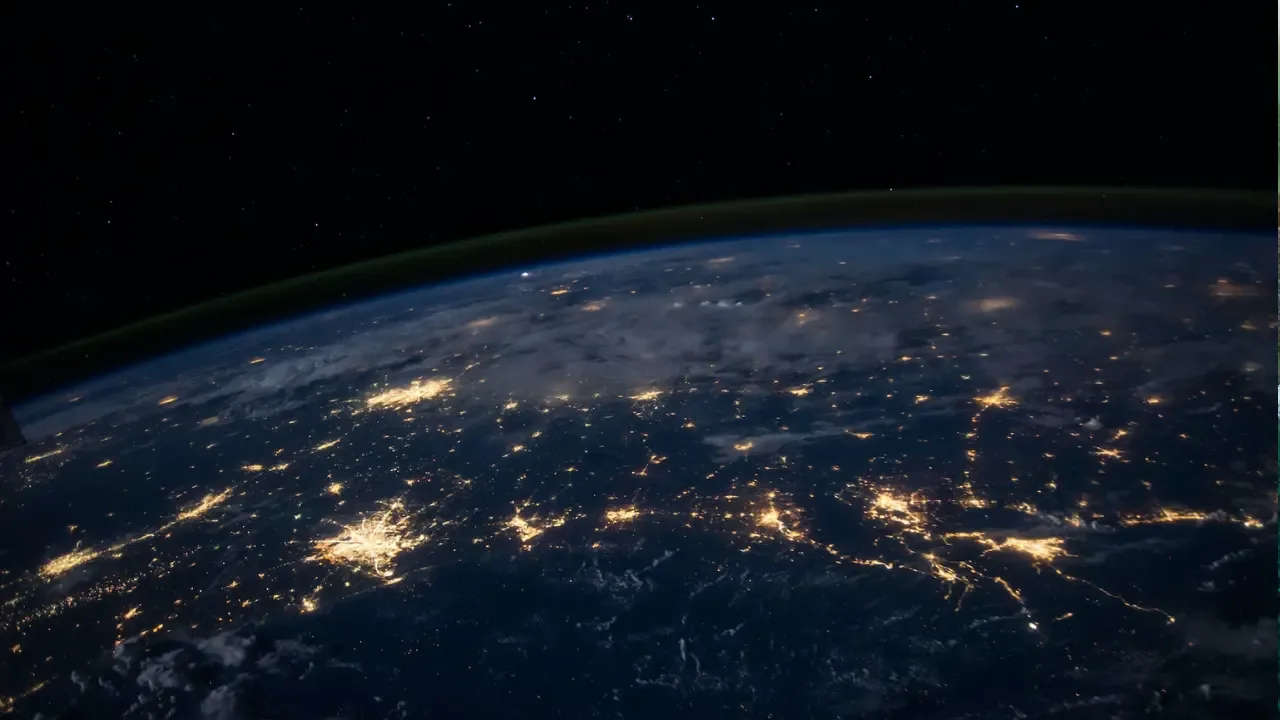
📱💡 Discovering Memory Usage of Your Android Application: A Guide for Developers 🚀
Are you an Android developer who is puzzled by the memory usage of your application? 🤔 Don't worry, we've got your back! In this blog post, we'll address common issues you might encounter and provide easy solutions for uncovering memory usage in your Android app. Let's dive in! 💪
⚠️ The Common Issue: Finding Memory Usage Programmatically ⚠️
Many developers, like you, want to programmatically fetch the memory usage of their Android applications. Luckily, there is a way to accomplish this! 🎉
💡 The Solution: Using Android's Debug.MemoryInfo Class 💡
Android provides the Debug.MemoryInfo
class, which offers valuable insights into memory usage. Here's a step-by-step approach to help you get started:
1️⃣ Import the Debug
class: First, make sure you import the Debug
class in your application file.
import android.os.Debug;
2️⃣ Utilize the getProcessMemoryInfo
method: To retrieve memory usage information, you can use the getProcessMemoryInfo
method of the Debug
class. Here's an example of how to use it:
Debug.MemoryInfo memoryInfo = new Debug.MemoryInfo();
Debug.getMemoryInfo(memoryInfo);
long memoryUsed = memoryInfo.getTotalPss() * 1024; // Total memory used in bytes
🌟 Congratulations! You now have the total memory used by your application. 🌟
📱 Capturing Free Memory on the Phone 📱
But wait, there's more! You also asked about getting the free memory of the phone. Here's an extra tip to help you achieve that:
1️⃣ Utilize the ActivityManager
class: The ActivityManager
class offers methods to retrieve various system-related information, including free memory. Follow these steps to obtain free memory details:
ActivityManager activityManager = (ActivityManager) getSystemService(ACTIVITY_SERVICE);
ActivityManager.MemoryInfo memoryInfo = new ActivityManager.MemoryInfo();
activityManager.getMemoryInfo(memoryInfo);
long memoryFree = memoryInfo.availMem; // Free memory in bytes
🔍 Now you have the free memory available on the phone! 🔍
📢 Take Action & Share Your Experience 📢
We hope this guide has been helpful in unraveling the mystery of memory usage in your Android application. Now it's time for you to put it into practice! Try retrieving memory usage and free memory in your app, and don't forget to share your experience in the comments below. We'd love to hear from you! 😄
🏆 Remember, understanding memory usage is crucial for optimizing your app's performance and ensuring a smooth user experience. Keep exploring and keep coding! 💻 Happy developing, fellow Android enthusiasts! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
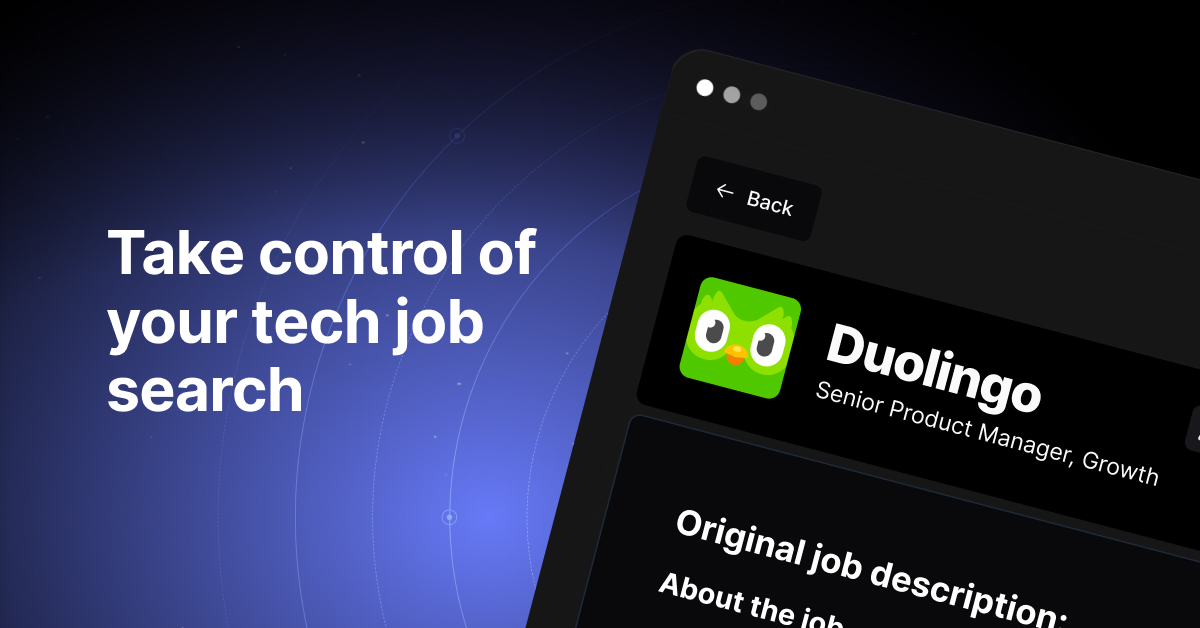