How do I determine whether an array contains a particular value in Java?
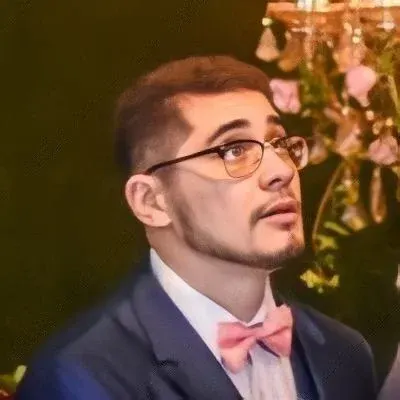
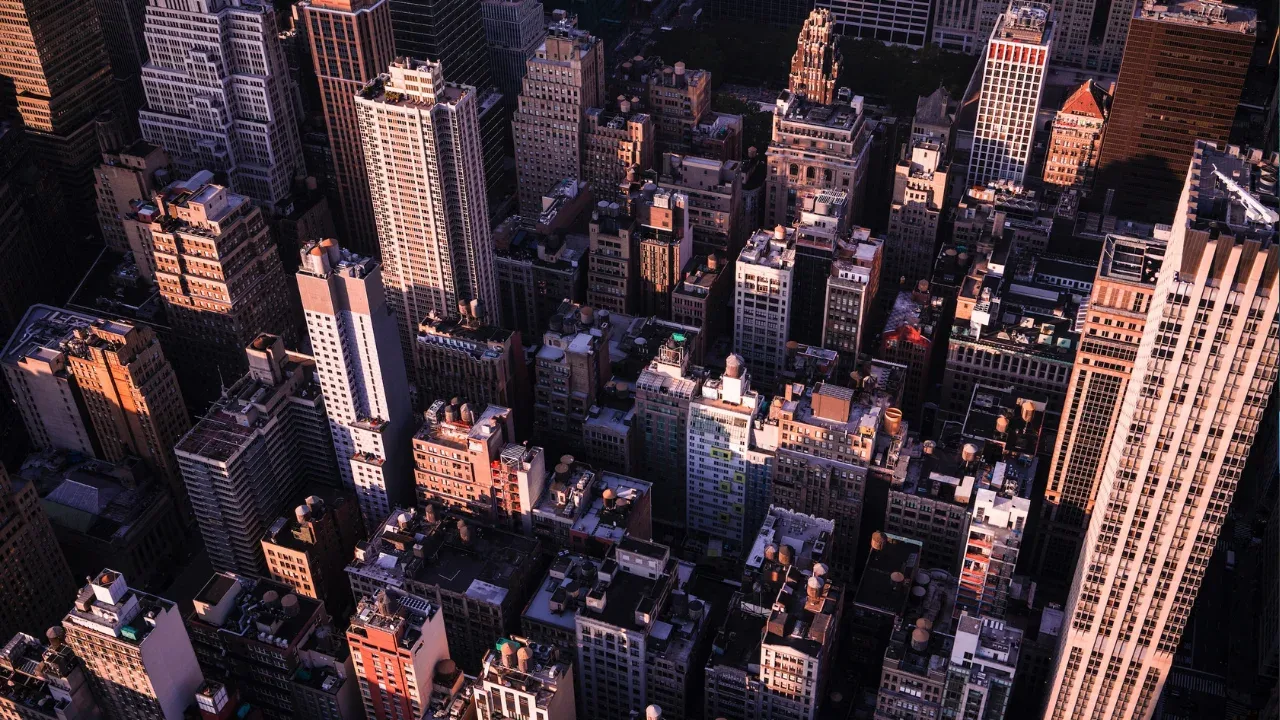
Title: How to Check if an Array Contains a Specific Value in Java 🧐
Introduction
Have you ever wondered how to determine whether an array contains a particular value in Java? 🤔 In this blog post, we will address this common issue and provide easy solutions to help you tackle this problem effortlessly! Whether you are a beginner or an experienced Java developer, we've got you covered! 💪
Problem Statement 🔍
Let's say you have a String[]
array called VALUES
, which contains various string values. You have a new string, let's call it s
, and you want to check if s
exists in the VALUES
array. How can you efficiently determine whether VALUES
contains s
? Let's find out! 💡
Solution 1: Using a For Loop 🔄
One simple approach is to iterate over each element in the array using a for
loop and check if the current element is equal to the desired value s
. Here's an example implementation:
boolean containsValue = false;
for(String value : VALUES) {
if(value.equals(s)) {
containsValue = true;
break;
}
}
In this solution, we initialize a boolean variable containsValue
to false
. Then, for each element in the VALUES
array, we compare it with s
using the equals()
method. If a match is found, we set containsValue
to true
and exit the loop using the break
statement. Finally, we can use the value of containsValue
to determine whether VALUES
contains s
.
Solution 2: Using the Arrays Class 💼
Java provides a handy Arrays
class that offers utility methods for working with arrays. One such method is Arrays.asList()
, which converts an array into a List
object. We can take advantage of this method to simplify our code. Here's how:
List<String> valuesList = Arrays.asList(VALUES);
boolean containsValue = valuesList.contains(s);
In this solution, we convert the VALUES
array into a List
using Arrays.asList()
. Then, we use the contains()
method of the List
object to check if s
exists in the list. The result of contains()
will be assigned to the containsValue
boolean variable.
Solution 3: Using Java 8 Streams 🌊
If you prefer a more concise and modern approach, you can utilize Java 8 Streams to solve this problem. Here's an example implementation:
boolean containsValue = Arrays.stream(VALUES).anyMatch(value -> value.equals(s));
In this solution, we use the Arrays.stream()
method to convert the VALUES
array into a stream. Then, we apply the anyMatch()
method with a lambda expression to check if any elements in the stream match the given condition. If a match is found, the containsValue
variable will be set to true
.
Conclusion 💡
Now you know multiple ways to determine whether an array contains a specific value in Java! You can choose the approach that best suits your coding style and requirements. We covered solutions using a for loop, the Arrays
class, and Java 8 Streams. 💪
Remember, understanding these concepts is crucial, as they can greatly simplify your code and make it more efficient. So go ahead, implement these solutions in your projects, and level up your Java skills! 😎
If you found this blog post helpful, make sure to share it with your friends and colleagues. Also, feel free to leave a comment below, sharing your thoughts and any additional questions you may have. Let's keep the conversation going! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
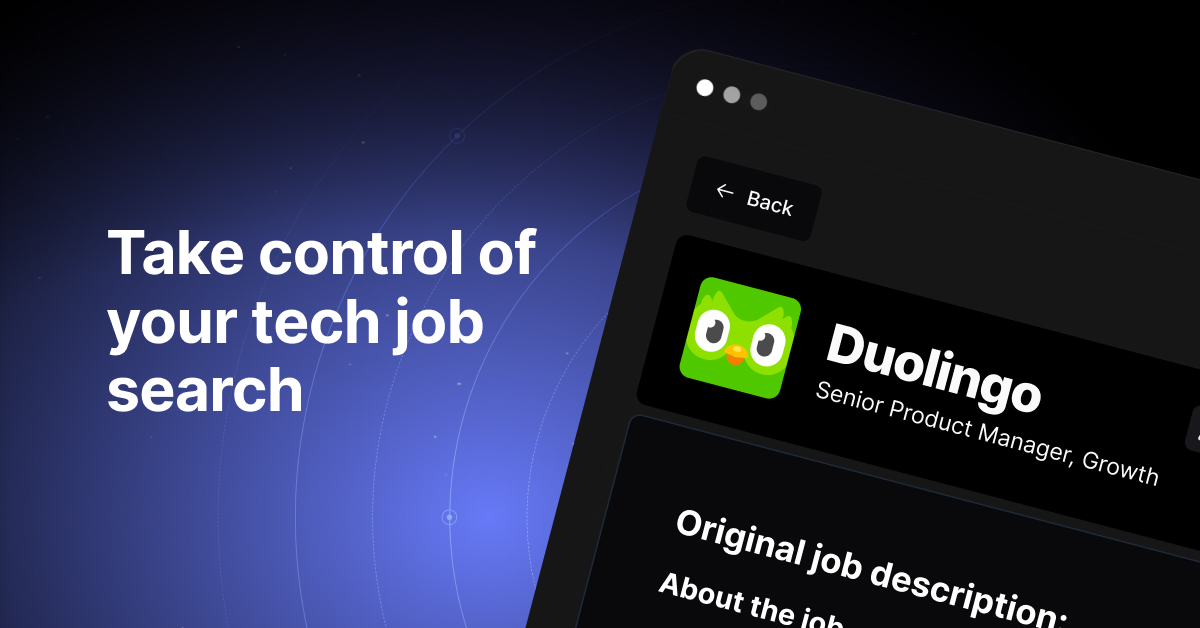