How do I declare and initialize an array in Java?
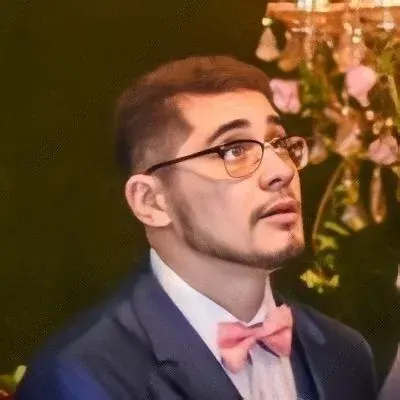
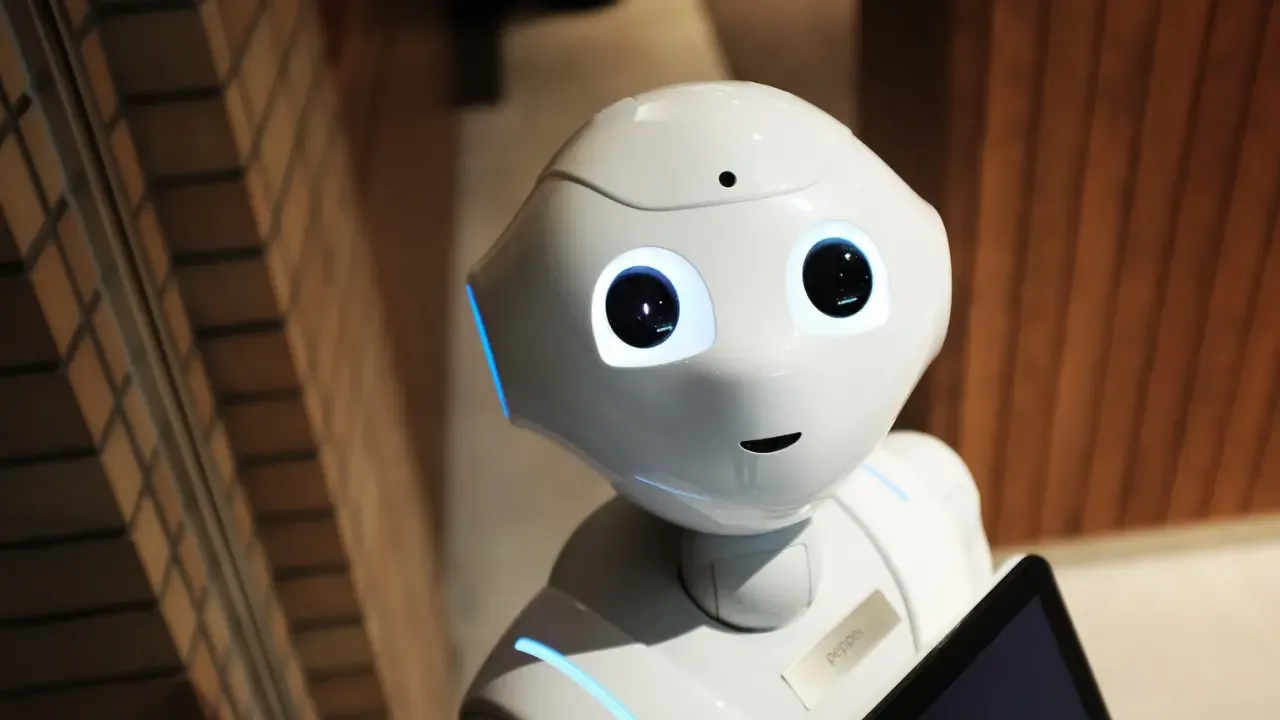
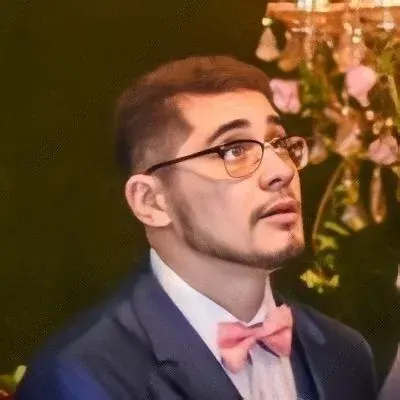
📚 The Complete Guide to Declaring and Initializing Arrays in Java 🚀
Are you new to Java and wondering how to declare and initialize an array? Look no further! In this guide, we'll walk you through the process step-by-step and provide easy solutions to common issues. Let's get started! 😊
Declaring an Array
To declare an array in Java, you'll need to specify its type and name. Here's the basic syntax:
dataType[] arrayName;
For example, if you want to declare an array of integers called "numbers", you would write:
int[] numbers;
You can also declare arrays of other data types, such as strings, booleans, or even custom objects. Just replace "dataType" with the appropriate type.
Initializing an Array
Once you've declared an array, you'll need to initialize it before you can use it. There are several ways to do this, depending on your needs.
1. Initialize with Literal Values
If you know the values you want to store in the array, you can initialize it with literal values. Here's an example:
int[] numbers = {1, 2, 3, 4, 5};
This creates an array of integers and initializes it with the values 1, 2, 3, 4, and 5.
2. Initialize with a Loop
If you want to initialize an array with a sequence of values, you can use a loop. Here's an example that initializes an array of even numbers:
int[] evenNumbers = new int[10]; // Create an array of size 10
for (int i = 0; i < evenNumbers.length; i++) {
evenNumbers[i] = i * 2;
}
In this example, we create an array of size 10 and use a loop to fill it with even numbers starting from 0.
3. Initialize with a Default Value
If you don't know the initial values or want to set all elements to a default value, Java provides a convenient way to do so. Here's an example:
boolean[] flags = new boolean[8]; // Create an array of size 8
Arrays.fill(flags, true); // Set all elements to true
In this example, we create an array of booleans and set all elements to the value "true" using the Arrays.fill()
method.
Common Issues and Solutions
Issue 1: Array Out of Bounds Exception
One common issue is accessing an invalid index of the array, which results in an ArrayIndexOutOfBoundsException
. To prevent this, make sure to use valid indices within the array's bounds.
Issue 2: Null Pointer Exception
Another issue arises when trying to access uninitialized or null arrays. To avoid this, always initialize the array before using it.
Issue 3: Forgetting Array Size
If you declare an array without specifying its size, you'll encounter a compilation error. To fix this, provide the array's size during declaration or at initialization.
Conclusion
Congratulations! You've learned how to declare and initialize arrays in Java. With this knowledge, you'll be able to store and manipulate collections of data efficiently. Remember to handle common issues such as array out of bounds exceptions and null pointer exceptions. 🎉
If you have any questions or additional tips, feel free to leave a comment below. Happy coding! 💻✨