How do I create a Java string from the contents of a file?
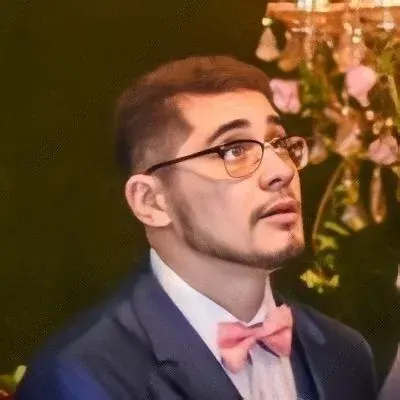
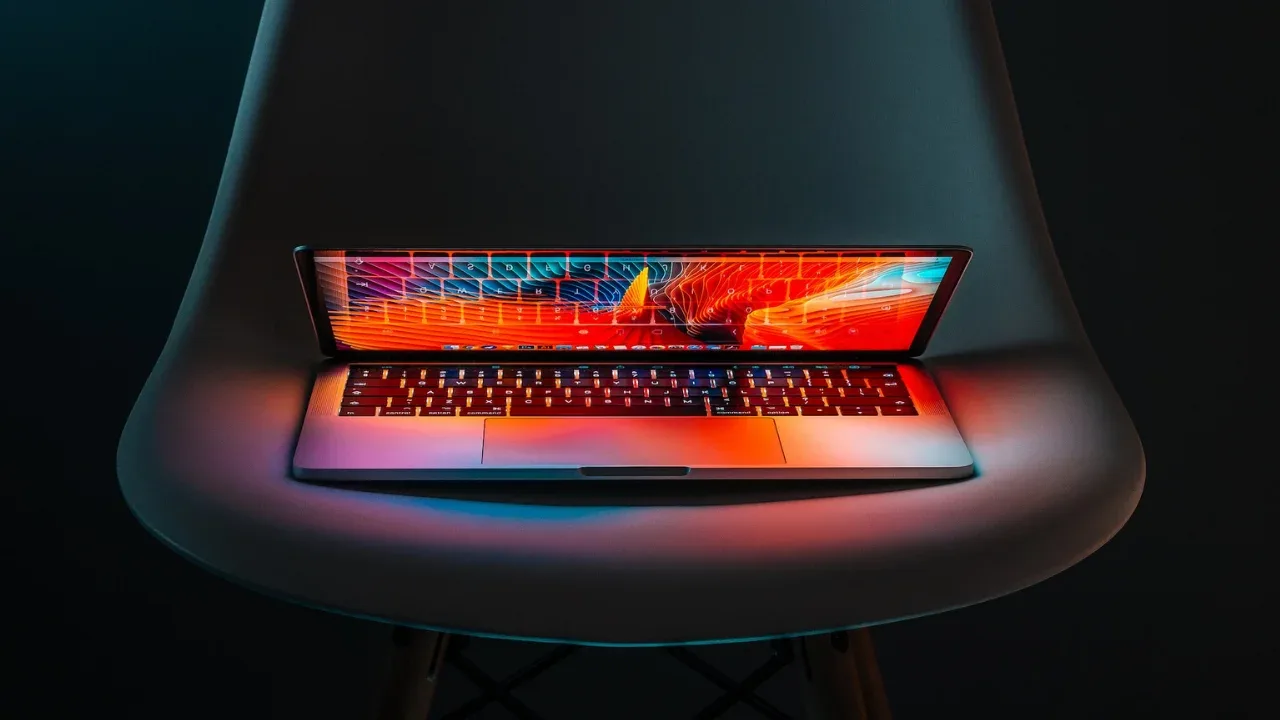
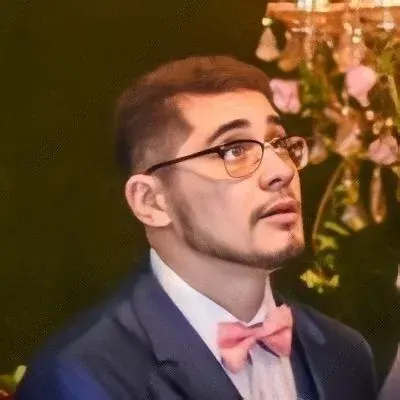
📚 Java 101: Creating a String from a File
Have you ever wondered how to create a Java string from the contents of a file? 🤔 Well, you're in luck because I'm here to guide you through it! 🎉 In this blog post, we'll explore a common approach to this problem, discuss potential issues, and provide you with some easy solutions. Let's dive in! 💪
The Traditional Approach 👵📜
The code snippet you provided is a popular method used by many developers to accomplish this task. It reads the file line by line and appends each line to a StringBuilder
object. Finally, it converts the StringBuilder
to a string and returns it. Here's the code again for reference:
private String readFile(String file) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line = null;
StringBuilder stringBuilder = new StringBuilder();
String ls = System.getProperty("line.separator");
try {
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
stringBuilder.append(ls);
}
return stringBuilder.toString();
} finally {
reader.close();
}
}
This approach is functional, reliable, and can handle files of various sizes. However, it does have some drawbacks that you should be aware of.
Potential Issues and Easy Solutions 🔍💡
1️⃣ No Error Handling: The current implementation does not adequately handle exceptions or errors. If an error occurs while reading or closing the file, the method will throw an exception, possibly leaving the file handle unclosed. A solution to this problem is to use a try-with-resources statement, introduced in Java 7, to automatically close the file reader:
private String readFile(String file) throws IOException {
StringBuilder stringBuilder = new StringBuilder();
String ls = System.getProperty("line.separator");
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
stringBuilder.append(ls);
}
}
return stringBuilder.toString();
}
By utilizing the try-with-resources statement, any resource opened within the parentheses will automatically be closed at the end of the block, ensuring proper cleanup and error handling.
2️⃣ Encoding Issues: The code assumes that the file's content is using the default character encoding of the system. This may lead to unexpected behavior if the file is encoded differently. To specify an explicit character encoding, you can use the InputStreamReader
class:
private String readFile(String file, String encoding) throws IOException {
StringBuilder stringBuilder = new StringBuilder();
String ls = System.getProperty("line.separator");
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(file), encoding))) {
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
stringBuilder.append(ls);
}
}
return stringBuilder.toString();
}
By passing the desired encoding to the InputStreamReader
constructor, you can ensure that the file is read correctly, regardless of the system's default encoding.
Your Turn! 🚀
And there you have it! You now know how to create a Java string from the contents of a file like a pro! 🎉
Go ahead, try out these solutions in your own code and let me know how it works for you! If you encounter any issues or have any further questions, feel free to leave a comment below. I'll be more than happy to help you out! 💪💬
Happy coding! 🧑💻🔥