How do I create a file and write to it?
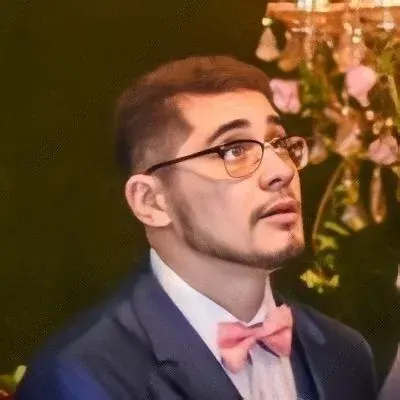
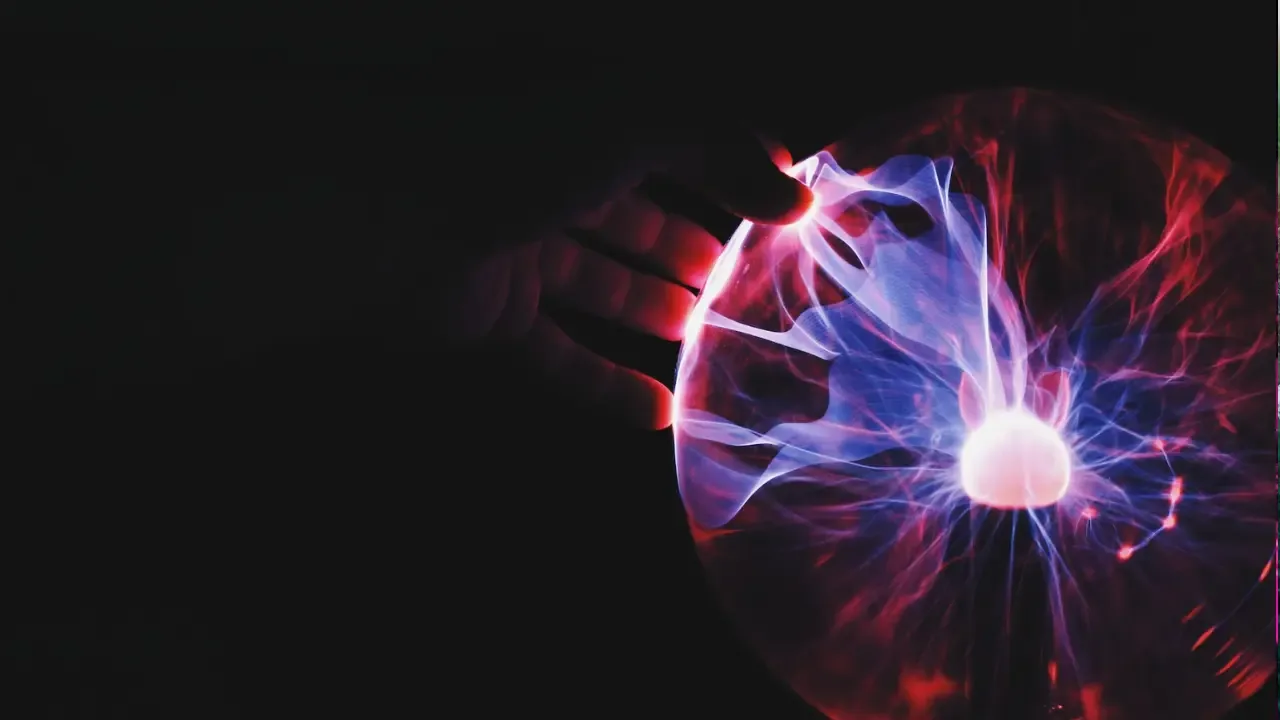
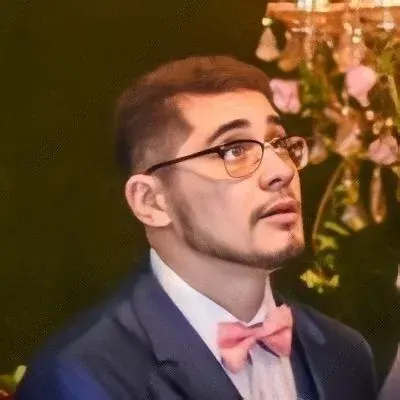
📝 Creating and Writing to a File in Java: Simplest Solutions
Are you feeling puzzled about creating and writing to a file in Java? Don't worry, we got you covered! In this blog post, we will explore the simplest way to tackle this task, addressing common issues and providing easy solutions.
💡 Understanding the Basics
Before diving into the solutions, let's quickly review the basics. In Java, the java.io
package provides classes and methods for file input and output operations. To create and write to a file, you need to:
Import the necessary classes:
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
Specify the file path and name:
String filePath = "path/to/your/file.txt";
Handle any potential exceptions by using the
try-catch
block.
Now, let's explore some common scenarios and their corresponding solutions!
🔍 Scenario 1: Creating a New File and Writing to It
If you want to create a new file and write some content to it, follow these steps:
Create a
File
object using the specified file path:
File file = new File(filePath);
Use a
FileWriter
object to write content to the file:
try (FileWriter writer = new FileWriter(file)) {
writer.write("Hello, World!");
} catch (IOException e) {
e.printStackTrace();
}
That's it! You have successfully created a new file and written the desired content to it.
🔍 Scenario 2: Appending to an Existing File
In case you need to append new content to an existing file, modify the solution as follows:
Create a
FileWriter
object with theappend
parameter set totrue
:
try (FileWriter writer = new FileWriter(file, true)) {
writer.write("Appending text to an existing file!");
} catch (IOException e) {
e.printStackTrace();
}
By setting append
to true
, you ensure that the newly written content is appended to the end of the file, rather than overwriting its existing content.
🌟 Call-to-Action: Share Your Thoughts and Experiences!
Now that you have learned the basic techniques for creating and writing to a file in Java, why not put your new knowledge into action? Try out these solutions and share your thoughts and experiences in the comments section below! We'd love to hear how this guide helped you.
Keep in mind that this is just the tip of the iceberg when it comes to file manipulation in Java. If you want to explore more advanced techniques or have specific questions, feel free to reach out!
Happy coding! 😄🚀