How do I count the number of occurrences of a char in a String?
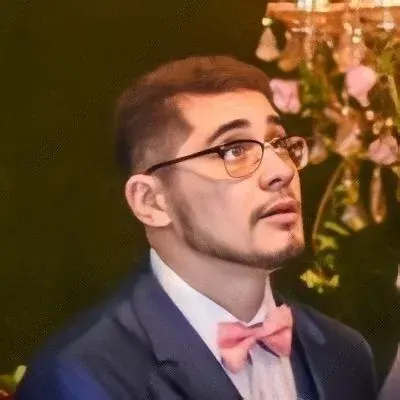
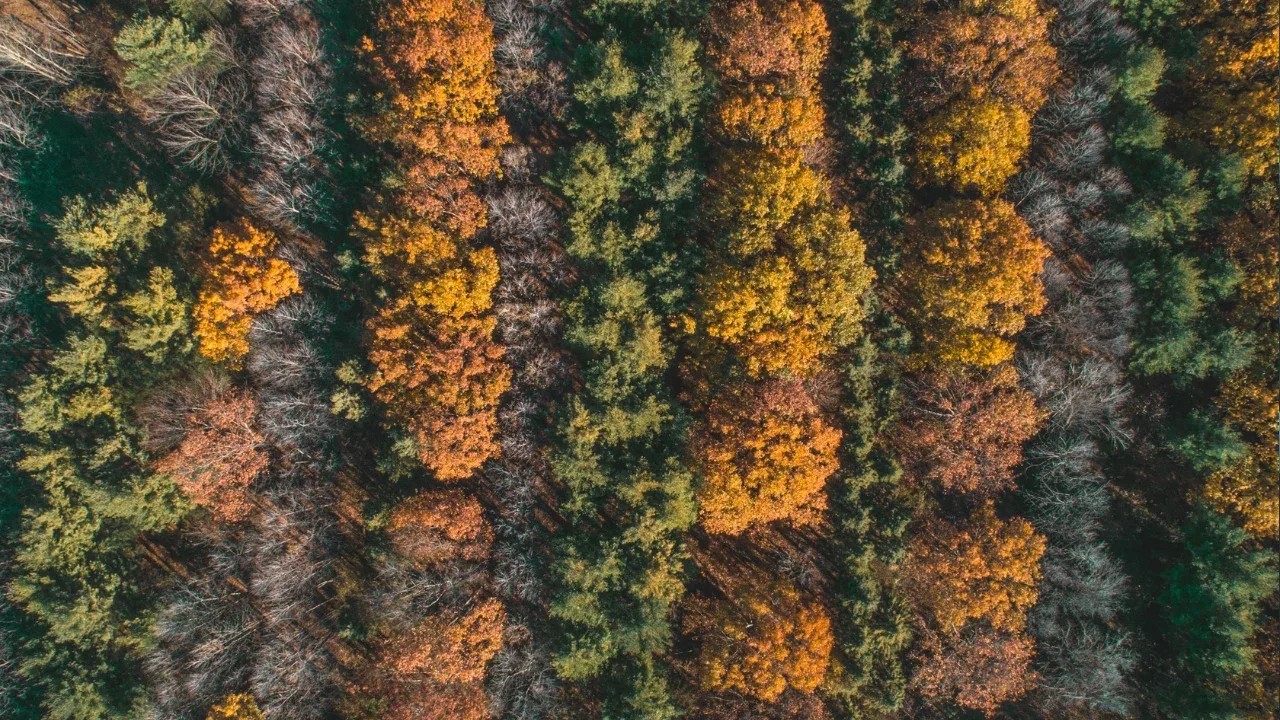
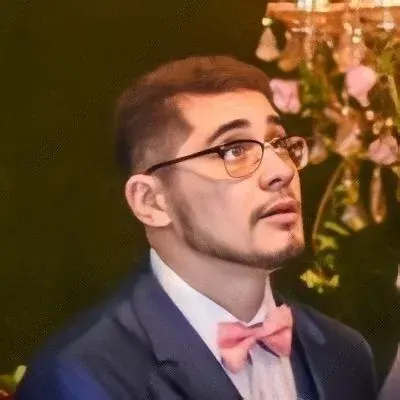
How to Count the Number of Occurrences of a Character in a String
Have you ever found yourself in a situation where you needed to count the number of occurrences of a specific character in a string? Maybe you want to see how many times a particular symbol appears in a sentence or extract some useful data from a string. Whatever the case may be, counting the occurrences of a character in a string can be a useful skill to have.
In this blog post, we'll explore a few easy and idiomatic ways to count the number of occurrences of a character in a string. We'll also address a common constraint mentioned by the original questioner - how to achieve this without using a loop.
The Problem: Counting the Occurrences of a Character in a String
Let's start by understanding the problem at hand. We have a string:
a.b.c.d
And we want to count the occurrences of the period character ('.') in this string. The goal is to find an idiomatic solution, preferably a one-liner, that counts the occurrences without using a loop. Challenges like these help us explore different approaches and learn new techniques.
Solution 1: Using the String.prototype.split()
method
One easy way to count the occurrences of a character in a string is by splitting the string into an array based on the character we want to count. We can then determine the number of occurrences by examining the length of the resulting array.
Here's an example using JavaScript:
const string = 'a.b.c.d';
const charToCount = '.';
const occurrences = string.split(charToCount).length - 1;
console.log(`The character "${charToCount}" appears ${occurrences} times.`);
In this solution, we split the string into an array using the period character as the delimiter. We then subtract 1 from the length of the resulting array to account for the fact that splitting the string creates one extra element. Finally, we log the number of occurrences to the console.
Solution 2: Using the Regular Expression
Another approach to counting the occurrences of a character in a string is by using Regular Expressions. Regular Expressions provide a powerful way to match and manipulate strings based on patterns.
Here's an example using JavaScript:
const string = 'a.b.c.d';
const charToCount = '.';
const regex = new RegExp(/./, 'g');
const occurrences = (string.match(regex) || []).length;
console.log(`The character "${charToCount}" appears ${occurrences} times.`);
In this solution, we create a Regular Expression that matches the character we want to count. We then use the match()
method on the string with the Regular Expression, which returns an array of all the matches. Finally, we access the length of the resulting array to get the number of occurrences.
Solution 3: Using the reduce()
method
If you're looking for a more functional approach, you can use the reduce()
method to count the occurrences in a one-liner.
Here's an example using JavaScript:
const string = 'a.b.c.d';
const charToCount = '.';
const occurrences = Array.from(string).reduce((count, char) => count + (char === charToCount), 0);
console.log(`The character "${charToCount}" appears ${occurrences} times.`);
In this solution, we convert the string into an array using Array.from()
. Then, we use the reduce()
method to iterate over each character in the array. We increment the count by 1 whenever we encounter the character we want to count. Finally, we log the number of occurrences to the console.
Conclusion: Counting the Occurrences Made Easy!
Counting the number of occurrences of a character in a string doesn't have to be complicated. By using simple techniques like splitting, Regular Expressions, or the reduce()
method, you can easily tackle this problem without breaking a sweat.
Next time you find yourself needing to count the occurrences of a character in a string, remember the techniques we explored today. They'll save you time and make your code more efficient.
Now it's your turn! Try out these solutions on your own and see which one works best for you. Do you have any other creative ways to count occurrences? Share them with us in the comments below!
Happy coding! 🚀✨