How do I copy an object in Java?
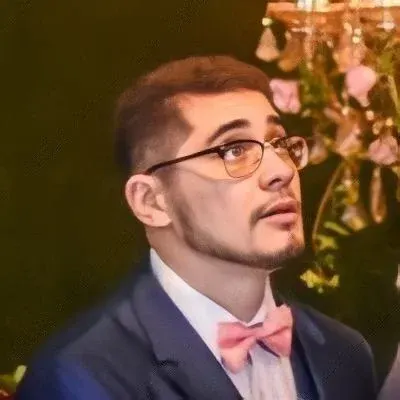
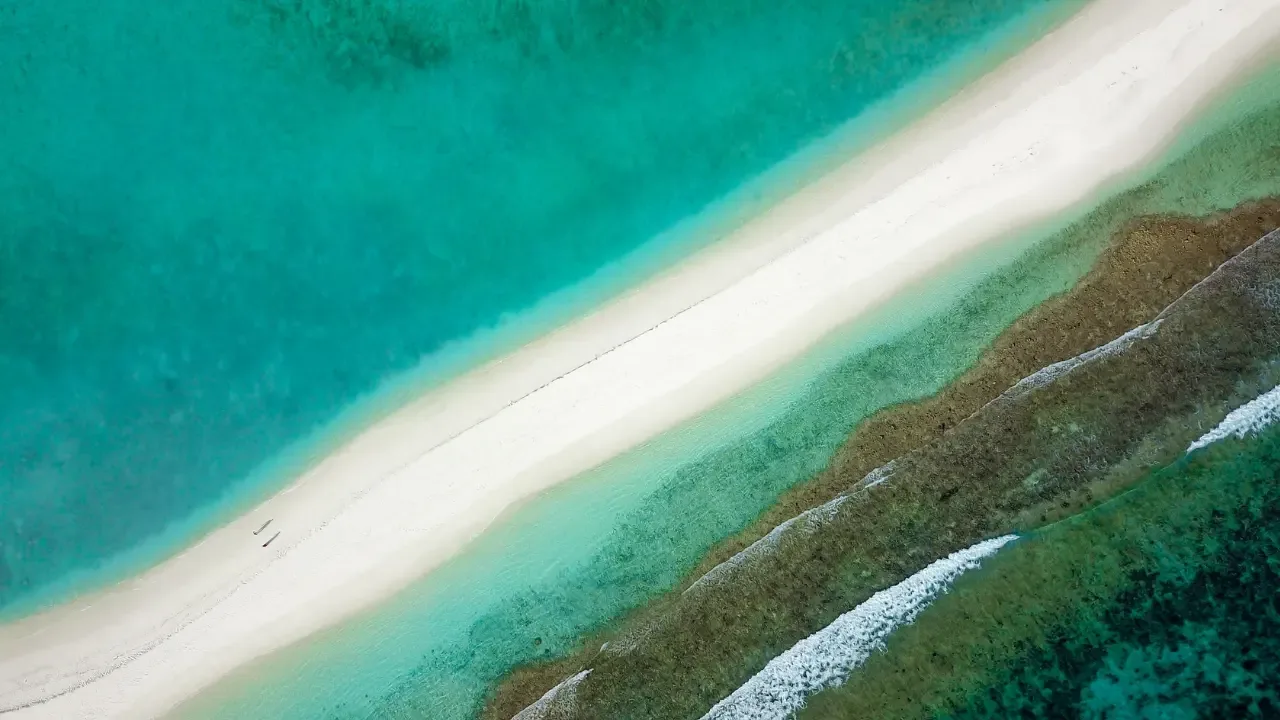
How to Copy an Object in Java? 📝🚀
Copying objects in Java can be a tricky task, especially when you want to create a fresh copy without affecting the original object. In this blog post, we'll explore a common issue and provide you with easy solutions to copy objects in Java. 🚀
Understanding the Problem
Consider the following code snippet:
DummyBean dum = new DummyBean();
dum.setDummy("foo");
System.out.println(dum.getDummy()); // prints 'foo'
DummyBean dumtwo = dum;
System.out.println(dumtwo.getDummy()); // prints 'foo'
dum.setDummy("bar");
System.out.println(dumtwo.getDummy()); // prints 'bar' but it should print 'foo'
In this example, we have an object dum
of type DummyBean
and we want to create a fresh copy of it and assign it to dumtwo
. However, when we change something in dum
, the same change is reflected in dumtwo
as well. 😱
Why does this happen?
The issue here is that Java copies the reference of the object when we assign it to another variable. So, both dum
and dumtwo
point to the same memory location, leading to unexpected behavior when modifying one of them. 😮
Solution 1: Use the Copy Constructor
One way to achieve object copying without affecting the original object is by using a copy constructor. By implementing a copy constructor in the DummyBean
class, we can create a fresh copy of the object. Here's how you can do it:
public class DummyBean {
private String dummy;
public DummyBean() {
// default constructor
}
public DummyBean(DummyBean other) {
this.dummy = other.dummy;
}
// getters and setters...
}
Now, let's modify the code to utilize the copy constructor:
DummyBean dum = new DummyBean();
dum.setDummy("foo");
System.out.println(dum.getDummy()); // prints 'foo'
DummyBean dumtwo = new DummyBean(dum);
System.out.println(dumtwo.getDummy()); // prints 'foo'
dum.setDummy("bar");
System.out.println(dumtwo.getDummy()); // prints 'foo' (Yay! 🎉)
With the copy constructor, we are creating a new instance of DummyBean
using the values from the original object. This way, any modifications to dum
will not affect dumtwo
.
Solution 2: Use Object Cloning
Another way to achieve object copying is by using the clone()
method in Java. However, this method comes with its own set of caveats and complexities. Nonetheless, let's explore how to copy objects using cloning:
Implement the
Cloneable
interface in theDummyBean
class:public class DummyBean implements Cloneable { // class definition... }
Override the
clone()
method:@Override public Object clone() throws CloneNotSupportedException { return super.clone(); }
Modify the code to utilize object cloning:
DummyBean dum = new DummyBean(); dum.setDummy("foo"); System.out.println(dum.getDummy()); // prints 'foo' DummyBean dumtwo = (DummyBean) dum.clone(); System.out.println(dumtwo.getDummy()); // prints 'foo' dum.setDummy("bar"); System.out.println(dumtwo.getDummy()); // prints 'foo' (Wohoo! 🎉)
By calling the clone()
method on dum
, we create a separate copy of the object, allowing independent modifications on dum
and dumtwo
.
Conclusion
Copying objects in Java can be a challenge, but with the right techniques, it becomes much simpler. In this blog post, we explored two solutions: using a copy constructor and utilizing object cloning. Choose the approach that best suits your needs.
Now, go ahead and copy those objects without any worries! 👯♂️
Have you encountered any other methods to copy objects in Java? Share your thoughts and experiences in the comments below. Let's learn from each other! 💬😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
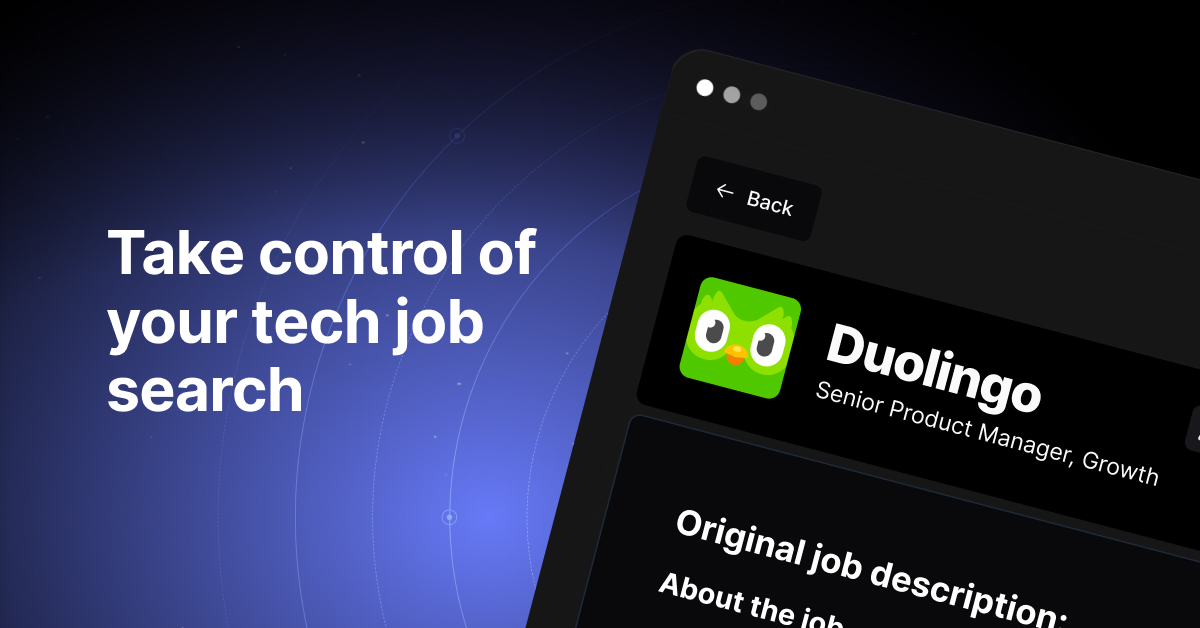