How do I convert a String to an InputStream in Java?
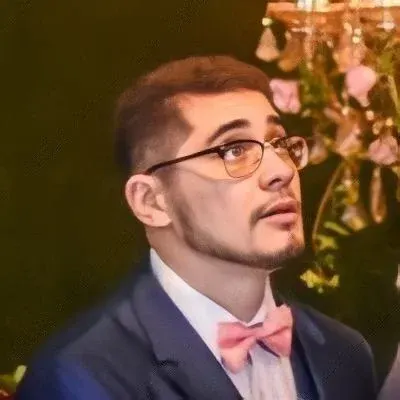
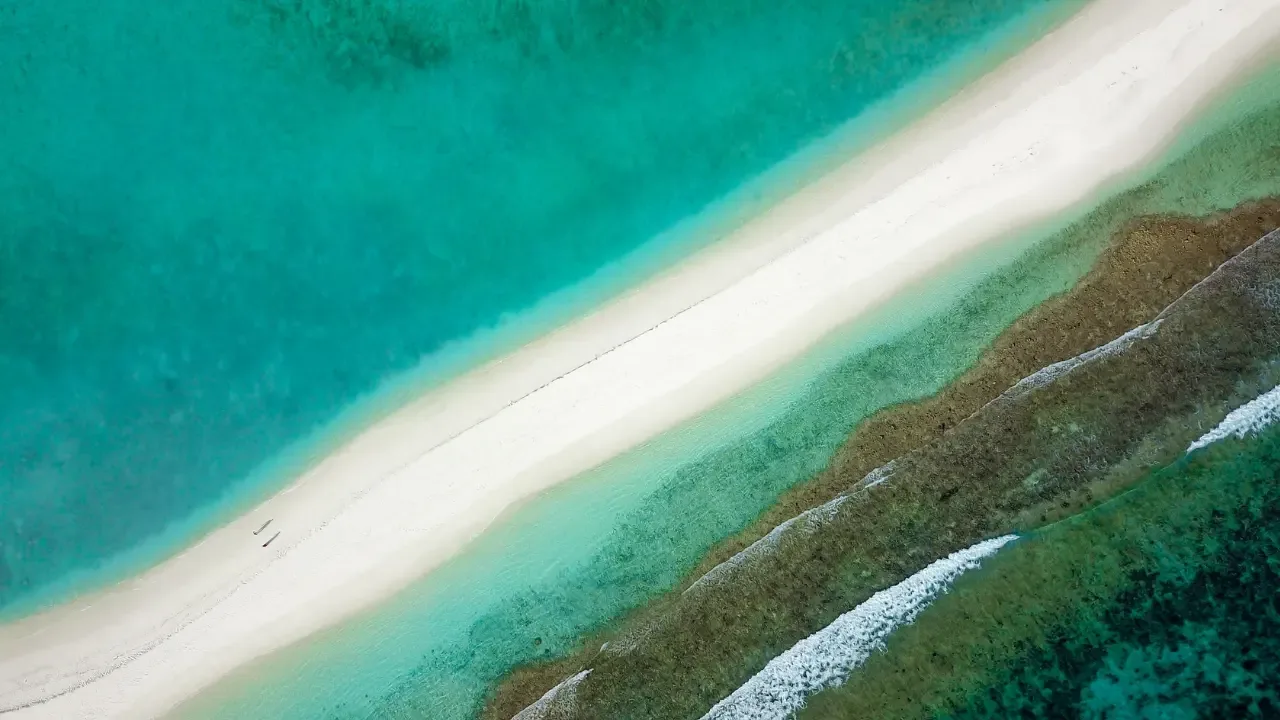
How to Convert a String to an InputStream in Java? 🌟
So, you've got a string in Java, and you want to convert it into an InputStream. Fear not, fellow developer, for I've got you covered! In this blog post, we'll explore common issues, provide easy solutions, and help you convert that string into an InputStream like a pro.
The Problem: String to InputStream Conversion
Let's start by understanding the problem at hand. You have a string, let's say our example string is "example"
, and you want to convert it into an InputStream. The good news is that there are multiple ways to achieve this in Java.
Solution 1: Using ByteArrayInputStream
The ByteArrayInputStream
class is our trusty friend when it comes to creating an InputStream from a byte array. Here's how you can do it:
String exampleString = "example";
byte[] stringBytes = exampleString.getBytes();
InputStream inputStream = new ByteArrayInputStream(stringBytes);
In this solution, we first convert the string into a byte array using the getBytes()
method. Then, we pass that byte array into the ByteArrayInputStream
constructor, which creates an InputStream object. Voilà! 🎉
Solution 2: Using StringReader and InputStreamReader
Another option is to use StringReader
and InputStreamReader
classes provided by Java's IO library. Here's how you can do it:
String exampleString = "example";
Reader reader = new StringReader(exampleString);
InputStream inputStream = new InputStreamReader(reader);
In this solution, we create a StringReader
object by passing our example string to its constructor. Then, we pass the StringReader
object to the InputStreamReader
constructor, which ultimately gives us an InputStream. Easy peasy! 😎
Solution 3: Using Apache Commons IO
If you're using the Apache Commons IO library in your project, converting a string to an InputStream becomes even simpler. First, make sure you have the Commons IO dependency added to your project. Then, you can use the IOUtils
class to accomplish this task:
String exampleString = "example";
InputStream inputStream = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
In this solution, we're leveraging the amazing IOUtils.toInputStream()
method provided by Apache Commons IO. We pass our example string and the desired character encoding (in this case, UTF-8), and the method does the rest. How convenient! 💪
Conclusion
In this blog post, we've explored multiple solutions to the common problem of converting a string to an InputStream in Java. Whether you choose to use ByteArrayInputStream
, StringReader
with InputStreamReader
, or Apache Commons IO, you now have the tools to tackle this challenge like a pro.
So, next time you find yourself grappling with converting a string to an InputStream, remember these solutions and choose the one that best fits your needs. Happy coding! 💻💡
Leave a comment below to let us know which solution you found most helpful, or share your own preferred method for string to InputStream conversion. We love hearing from fellow developers!
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
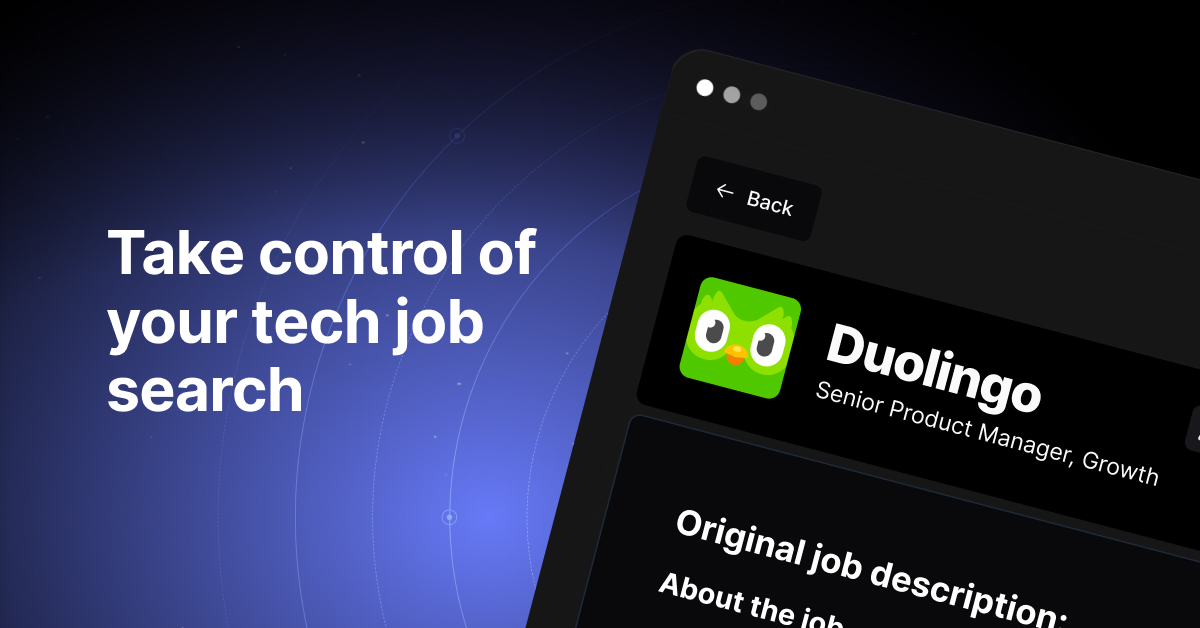