How do I convert a Map to List in Java?
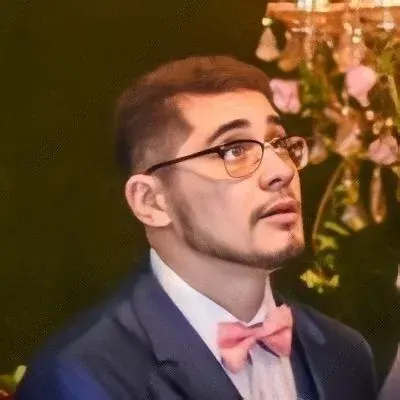
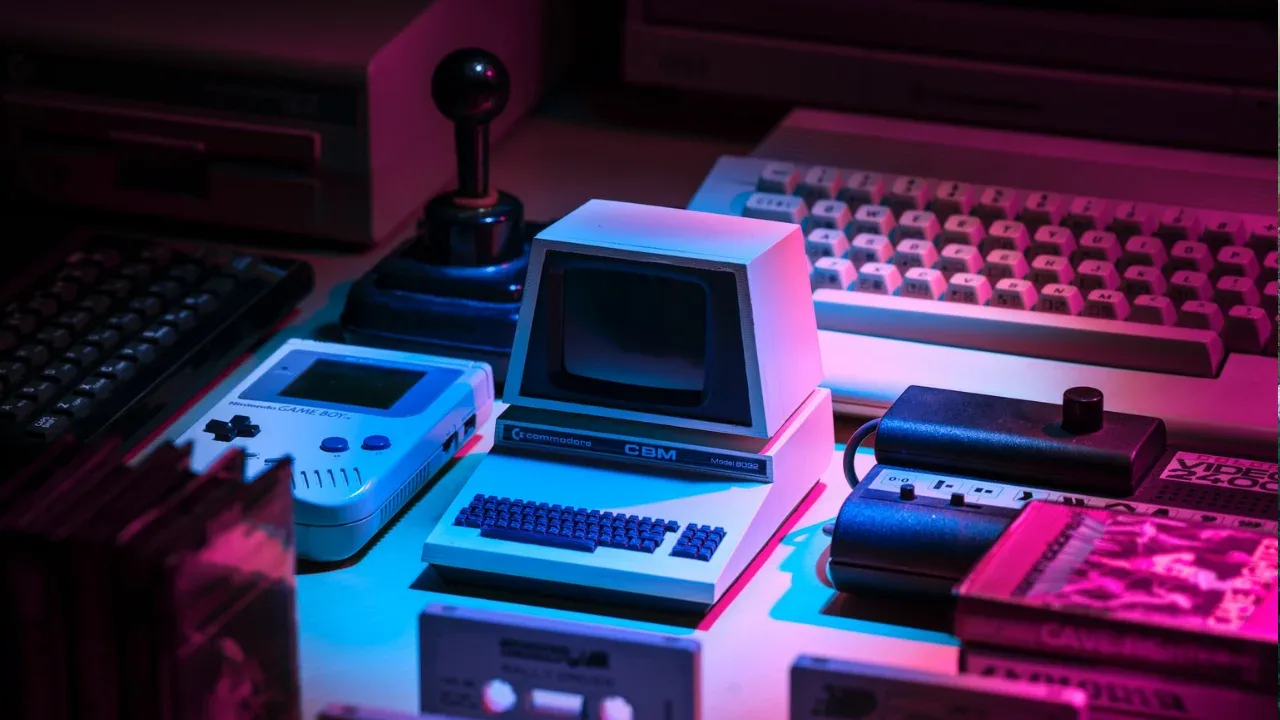
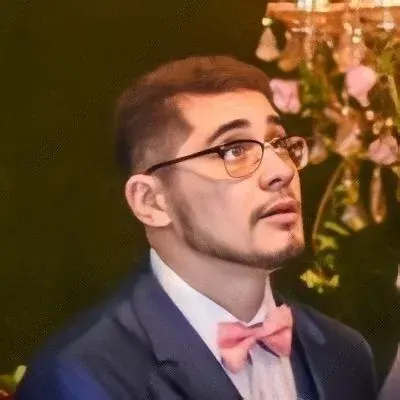
Converting a Map to List in Java: The Simple Solution! 😎📝🔨
Are you struggling to convert a Map<key,value>
to a List<value>
in Java? Don't worry, we've got you covered! This blog post will walk you through the common issues faced when dealing with this problem and provide you with an easy solution. Let's dive right in! 💪💡
The Problem: Converting a Map to List
So, you have a Map<key,value>
and you need to convert it into a List<value>
. The question arises: should you iterate over all map values and insert them manually into a list? Well, there's a simpler and more efficient way to achieve this! 🤔
The Easy Solution: Utilizing the Power of Java Streams! ⚡🚀
Java Streams, introduced in Java 8, provide a convenient and expressive way to perform operations on collections. To convert a Map<key,value>
to a List<value>
, we can leverage the power of Streams and follow these steps:
Retrieve the values from the map using the
values()
method.Stream the values using the
stream()
method.Collect the streamed values into a
List
using thecollect()
method.
Sounds easy, right? Let's write some code to solidify our understanding! 👩💻💡
Map<key, value> map = new HashMap<>();
// Populate the map with data
List<value> list = map.values().stream().collect(Collectors.toList());
Voila! 🎉 With just a few lines of code, you've successfully converted your Map<key,value>
to a List<value>
using Java Streams!
Handling Edge Cases
In some cases, you might encounter null values or duplicate entries in your Map. If you want to exclude null values or remove duplicates while converting, you can use additional Stream operations to filter or distinct the values accordingly. The flexibility of Java Streams allows you to handle these edge cases seamlessly! 😊🔍🔍
Summing It Up
Converting a Map<key,value>
to a List<value>
doesn't have to be a daunting task. By harnessing the power of Java Streams, you can achieve this in a simple and efficient manner. Remember to handle any edge cases that might arise, such as null values or duplicates, using additional Stream operations. Now, go ahead and give it a try! Happy coding! 🙌👨💻
Did you find this blog post helpful? Have any questions or suggestions? We'd love to hear from you in the comments below! Let's make learning and coding fun together! 😄💬👇
Don't forget to share this post with your friends and colleagues who might find it useful. Sharing is caring after all! 🌟🤝
Stay tuned for more exciting tech tips, tricks, and tutorials. Until then, happy coding! 🚀💻😊