How do I compare strings in Java?
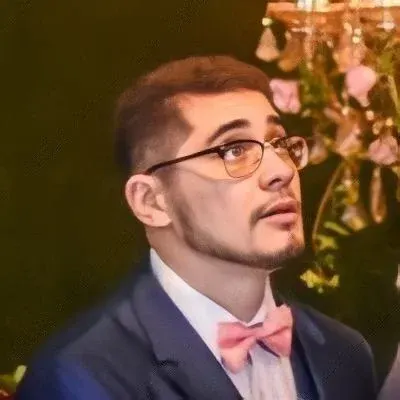
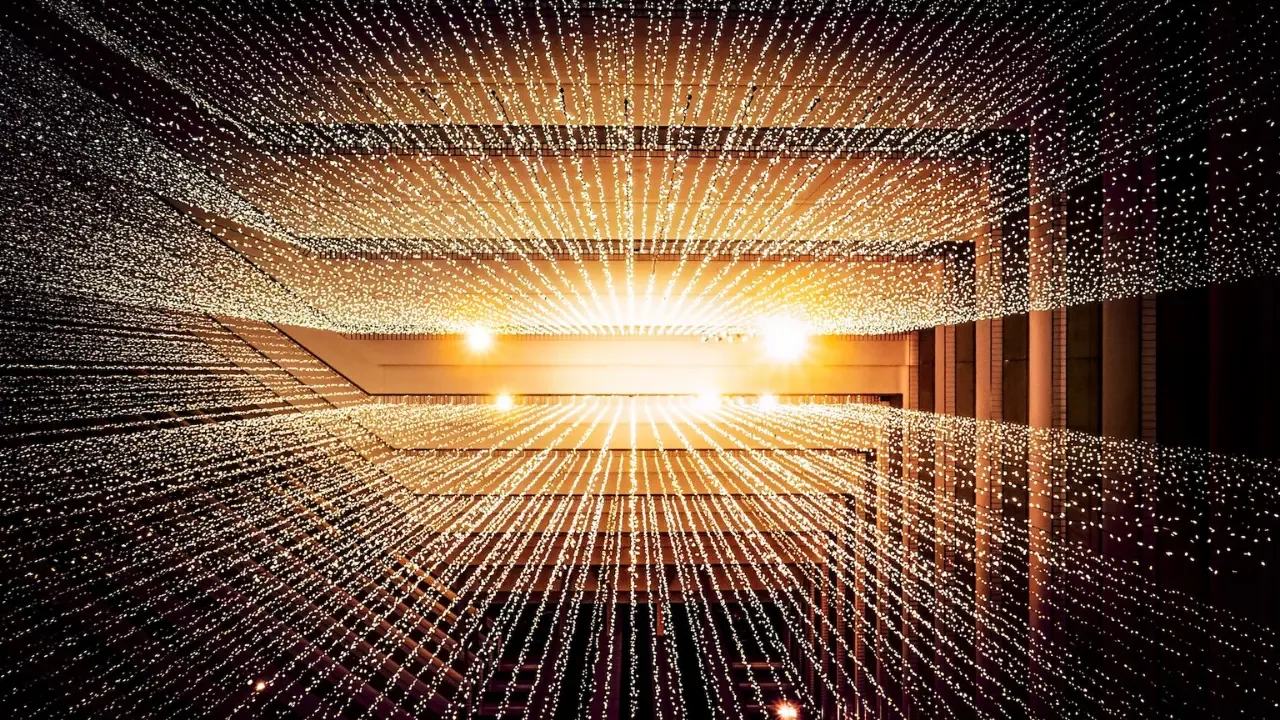
Comparing Strings in Java: The "==" vs ".equals()" Dilemma 🤔
Welcome to another blog post where we dive into the fascinating world of Java programming! Today, we're going to unravel the mystery behind comparing strings in Java and shed light on the common issue many developers face when choosing between the "==" operator and the ".equals()" method. Let's get started! 😎
The Bug Fix 💡
Our journey begins with a bug that our intrepid programmer stumbled upon. They had been using the "==" operator to compare strings throughout their program. However, after making a simple change to use ".equals()" instead, the bug magically vanished! 🐛✨
The Conundrum 🤔
Naturally, our curious programmer asks, "Is '==' bad? When should I use it, and when should I avoid it? What's the difference between '==' and '.equals()'?" These are fantastic questions, and we're here to demystify the whole situation for you! 🚀
Understanding the Difference 🔄
In Java, the "==" operator compares the references of two objects, while the ".equals()" method compares their contents. 🎯
Let's break it down with a simple example:
String firstString = "Hello";
String secondString = new String("Hello");
boolean usingDoubleEqual = (firstString == secondString); // Comparing references
boolean usingEquals = firstString.equals(secondString); // Comparing contents
In this example, "firstString" and "secondString" may seem identical, but they are not. While the contents (i.e., the actual string value) are the same, they are stored in different memory locations, resulting in different references. Here's where the difference becomes crucial! 🔍
When to Use "==" and ".equals()" 🤷♀️
Use "==" if you want to check whether two objects refer to the exact same memory location. This is often useful when comparing primitive data types or checking if an object is null:
int a = 5;
int b = 5;
boolean isEqual = (a == b); // true
String name = "John";
boolean isNull = (name == null); // false
Use ".equals()" if you need to compare the contents of two objects. This is crucial when dealing with objects that store information, such as strings, where the equality is based on the characters within the string:
String movie1 = "Black Panther";
String movie2 = "Black Panther";
boolean isSame = movie1.equals(movie2); // true
The Conclusion ✅
Now that you understand the fundamental difference between "==" and ".equals()" in Java, you can make an informed decision when comparing strings. Remember to use "==" for checking references and ".equals()" for comparing contents. And if you encounter bugs related to string comparison, always opt for ".equals()" to ensure accurate results. 🙌
Your Turn! 📣
Have you ever been caught in the "==" vs ".equals()" dilemma? Share your experience in the comments below! We love to hear your stories and insights. Let's geek out together! 👇😄
So, until next time, happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
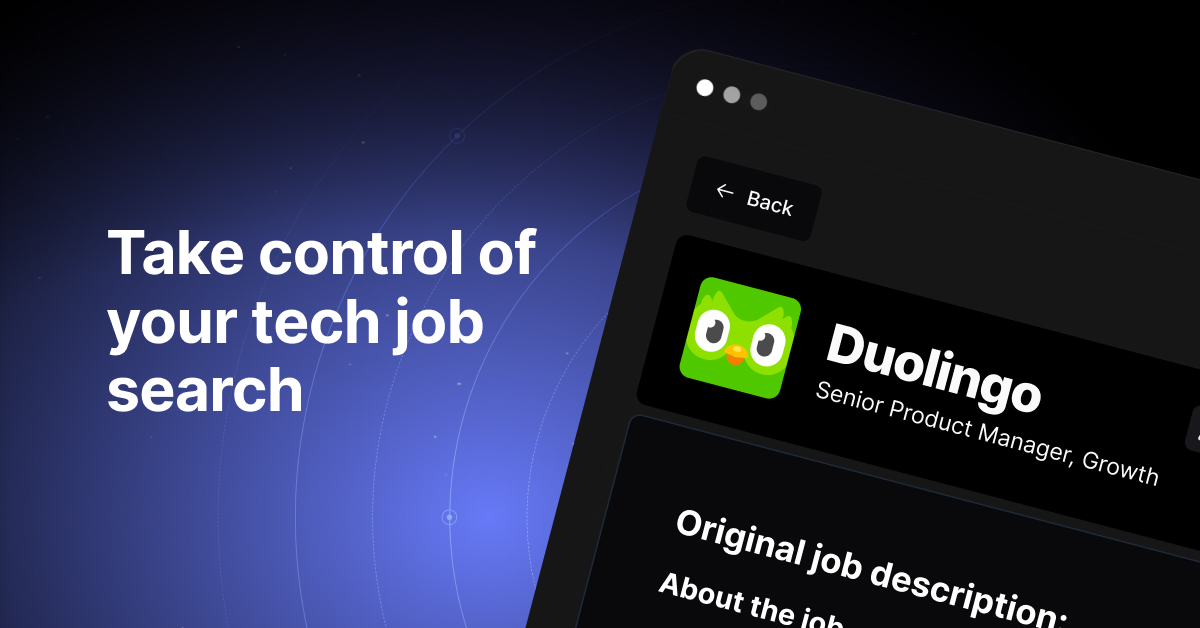