How do I call the default deserializer from a custom deserializer in Jackson
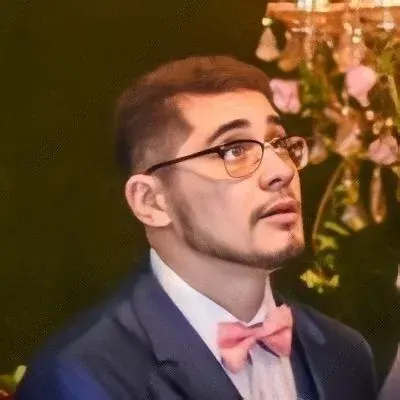
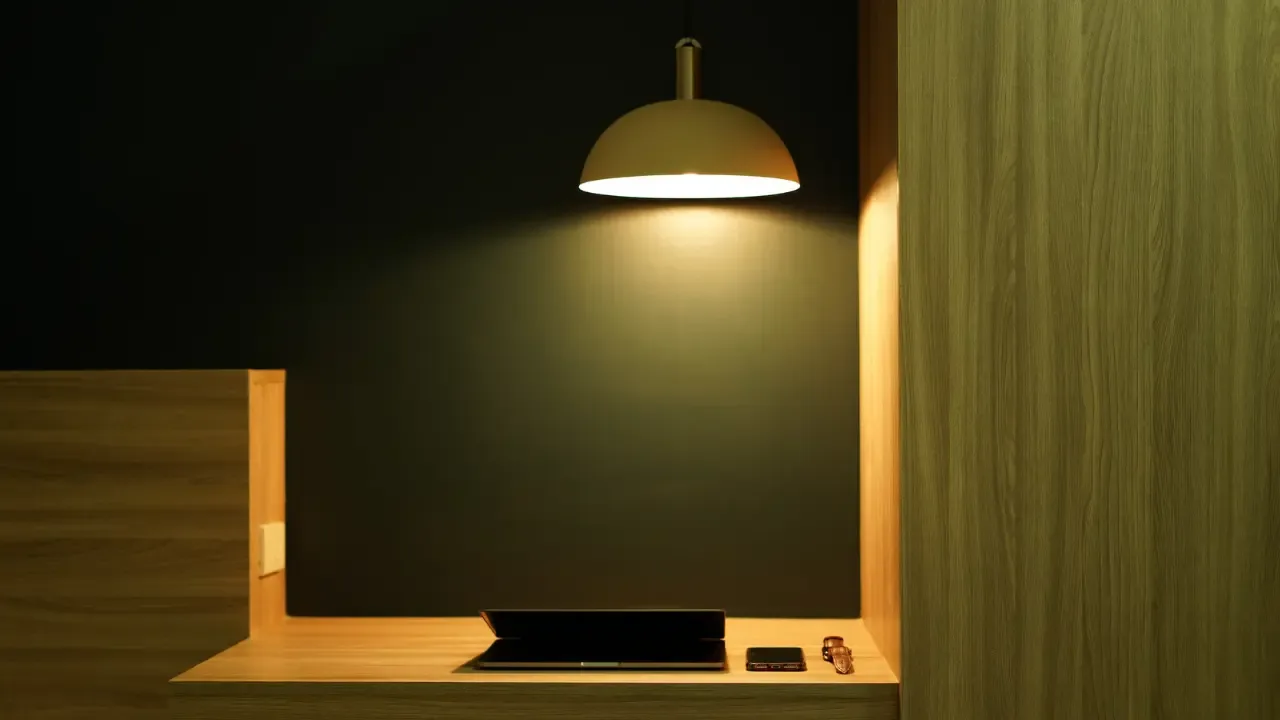
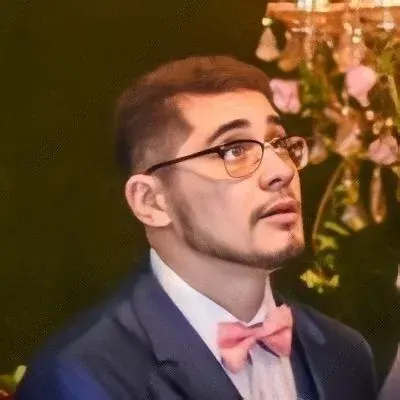
Calling the Default Deserializer from a Custom Deserializer in Jackson 🧩
So, you're facing a problem in your custom deserializer in Jackson. You want to access the default deserializer to populate the object you're deserializing into, before performing some custom actions. But how do you do that?
The Current Code ⚙️
First, let's take a look at your current code:
public class UserEventDeserializer extends StdDeserializer<User> {
...
@Override
public User deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
ObjectCodec oc = jp.getCodec();
JsonNode node = oc.readTree(jp);
User deserializedUser = null;
deserializedUser = super.deserialize(jp, ctxt, new User());
// Exception - UnsupportedOperationException
// I want to access the default spring deserializer for my User class.
// Special logic
return deserializedUser;
}
}
In this code, you tried to call the default deserializer by using super.deserialize(jp, ctxt, new User())
. However, this approach throws an UnsupportedOperationException
because the deserializer is not implemented.
Getting Access to the Default Deserializer 🌟
To access the default deserializer and populate your POJO object before executing your custom logic, you can do the following:
Create a new instance of the object you're deserializing into:
User defaultUser = new User();
Use
readValue()
to deserialize into this default object:User deserializedUser = oc.readValue(node.traverse(), User.class);
Perform any additional custom logic you need:
// Special logic
Return the final deserialized object:
return deserializedUser;
Final Solution 🎉
With these steps, your updated code should look like this:
public class UserEventDeserializer extends StdDeserializer<User> {
...
@Override
public User deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
ObjectCodec oc = jp.getCodec();
JsonNode node = oc.readTree(jp);
User defaultUser = new User(); // Step 1
User deserializedUser = oc.readValue(node.traverse(), User.class); // Step 2
// Special logic
return deserializedUser; // Steps 3 and 4
}
}
Explaining the Solution 📚
We create a new instance of the object we're deserializing into. This object will be used as the default object before any custom logic is applied.
Using the
ObjectCodec
obtained from theJsonParser
, we can callreadValue()
to deserialize the JSON directly into theUser
object. This effectively uses the default deserialization behavior provided by Jackson.After deserialization, we can perform any additional custom logic required.
Finally, we return the deserialized object.
Additional Approaches and Considerations 🤔
You mentioned trying different approaches like initializing a BeanDeserializer
, overloading the AnnotationIntrospector
, and using JsonDeserializerBuilders
, but with limited success. While those approaches may work in some scenarios, they can add unnecessary complexity.
The solution we provided offers a clean and straightforward approach to access the default deserializer in Jackson without having to read the JsonDeserializer
annotation or deal with complicated context manipulation.
If you encounter any further issues or have additional questions, please feel free to ask! We're here to help. 👍
Your Turn! 📣
Have you come across this issue in your own Jackson deserialization work? How did you solve it? Share your experiences and insights in the comments below! Let's learn from each other and make our code even stronger. 💪