How do I call one constructor from another in Java?
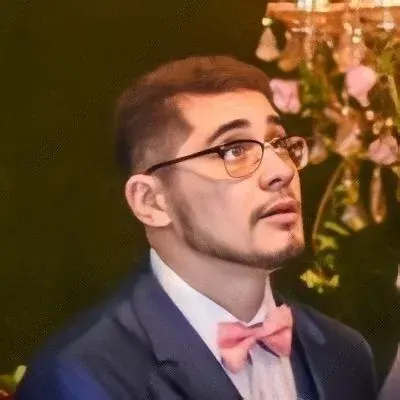
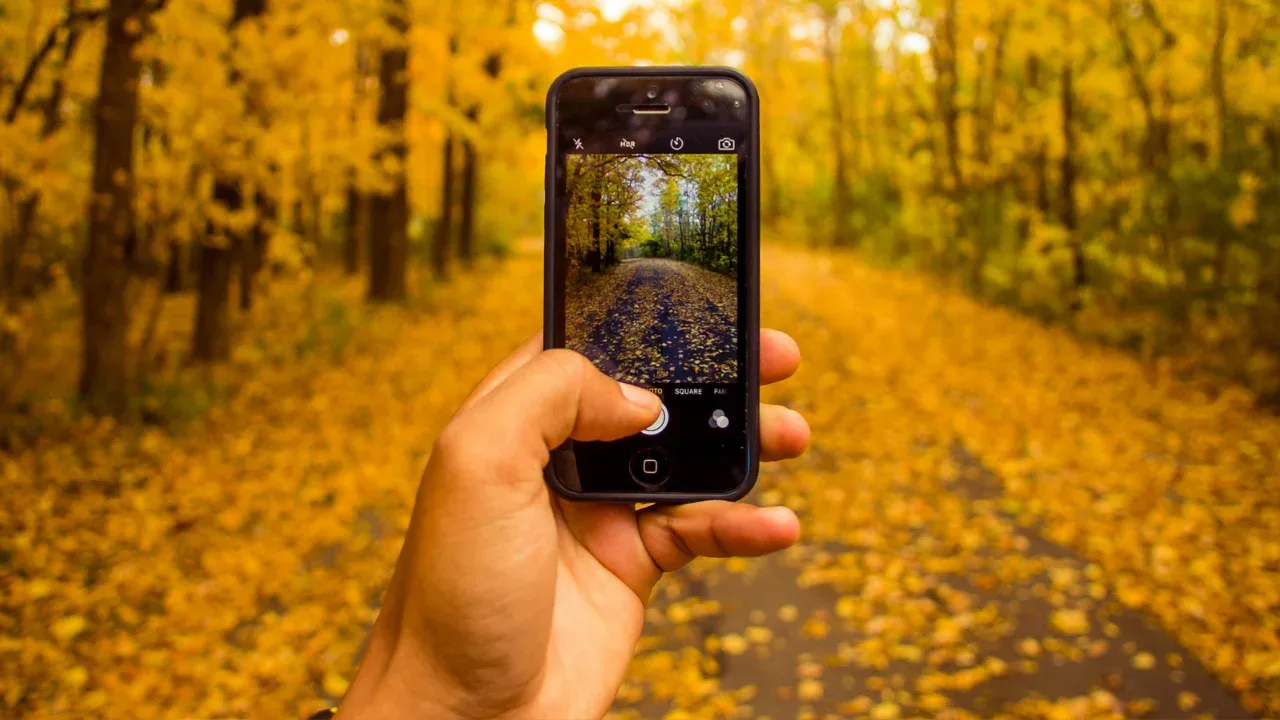
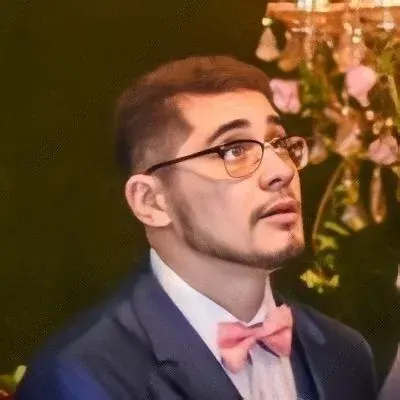
How to Call One Constructor from Another in Java 😎
So you've found yourself in a situation where you need to call one constructor from another in Java? Don't worry, it's a common question and fortunately, there's a straightforward solution! In this blog post, we'll explore how to call constructors within the same class, discuss different approaches, and provide you with practical examples. Let's dive in! 🚀
Understanding the Need for Calling Constructors
Before we jump into the "how," let's understand why we might want to call one constructor from another. Sometimes, we come across scenarios where multiple constructors are provided in a class to handle different situations or input variations. However, certain pieces of code common to all constructors need to be executed. To avoid duplicating code, we can call one constructor from another. It ensures that the common code is executed only once, increasing efficiency and maintainability. 🙌
The Default Approach: this()
To call one constructor from another, Java provides a simple yet powerful keyword - this()
. By using this()
followed by parentheses with appropriate arguments, we can invoke another constructor within the same class. This approach allows us to reuse code and delegate work from one constructor to another.
Here's an example to illustrate how it works:
public class MyClass {
private int value;
public MyClass() {
this(0); // Calling another constructor with default value
}
public MyClass(int value) {
this.value = value;
}
}
In the code snippet above, the parameterless constructor MyClass()
calls the second constructor MyClass(int value)
using this(0)
. The value 0
is passed as an argument to set the initial value of value
.
Calling Multiple Constructors: Chaining Approach
In scenarios where we have multiple constructors and want to call a specific constructor with added functionality, the method chaining approach can be useful. Let's see an example to make it crystal clear:
public class Employee {
private String name;
private int age;
private String department;
public Employee(String name) {
this(name, 0); // Calling another constructor with default values
}
public Employee(String name, int age) {
this(name, age, "Unknown"); // Calling another constructor with default department
}
public Employee(String name, int age, String department) {
this.name = name;
this.age = age;
this.department = department;
}
}
In the code snippet above, the first constructor Employee(String name)
calls the second constructor Employee(String name, int age)
using this(name, 0)
. Similarly, the second constructor calls the third constructor Employee(String name, int age, String department)
using this(name, age, "Unknown")
. This way, we can chain our constructors, each providing default or additional parameters.
📢 Action Time: Level Up Your Java Constructor Game!
Now that you've learned how to call one constructor from another, go ahead and try it out in your code. Look for opportunities where code can be reused, and use this()
to avoid duplication. Not only will it make your code cleaner and more maintainable, but it will also impress other developers with your Java skills! 💪
Remember, practice makes perfect! So keep exploring and experimenting with constructor calls in Java. And don't forget to share your experiences and questions in the comments below. Let's continue learning together and level up our Java skills! 👩💻👨💻
Happy coding! 😄